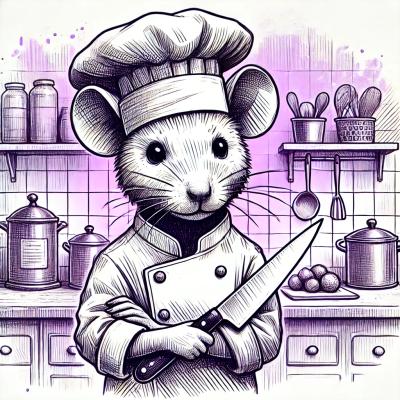
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@evandrolg/thequeue
Advanced tools
Easy way to queue delayed callbacks. Designed to be used on both client and server-side.
To install thequeue
, execute:
$ npm install @evandrolg/thequeue
or
$ yarn add @evandrolg/thequeue
thequeue
was designed to call functions that need to be invoked at a given time (e.g tracking functions that should be performed at a given time for performance reasons).
Its api is quite simple, as the example below shows:
import thequeue from 'thequeue';
const q = thequeue();
const fn1 = () => console.log('fn1');
q.register(fn1);
const fn2 = () => console.log('fn2');
q.register(fn2);
const fn3 = () => console.log('fn3');
q.register(fn3);
q.start();
// the functions will be called in the order in which they were added to the queue.
thequeue
was also designed with performance in mind and all registered functions are in a Queue that was implemented using a LinkedList. This means that the functions are registered in constant time and are processed (when the start
method is invoked) in linear time, without adding any extra space in memory.
FAQs
Easy way to queue delayed callbacks.
We found that @evandrolg/thequeue demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.