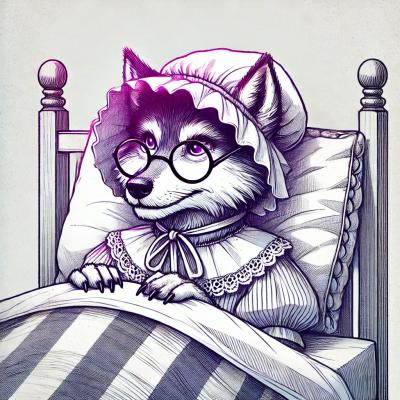
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@exodus/schemasafe
Advanced tools
The @exodus/schemasafe npm package is a tool for validating JSON objects against JSON Schema definitions. It is designed to be secure, fast, and standards-compliant, providing developers with a reliable way to ensure that their data conforms to specified formats and rules. This package supports JSON Schema Draft-07 and offers various options for customization and optimization.
Validation
This feature allows you to validate JSON objects against a JSON Schema. The example demonstrates creating a schema for an object with 'name' and 'age' properties and then validating objects against this schema.
{"const validator = require('@exodus/schemasafe').validator;\nconst schema = {\n type: 'object',\n properties: {\n name: { type: 'string' },\n age: { type: 'number' }\n },\n required: ['name', 'age'],\n additionalProperties: false\n};\n\nconst validate = validator(schema);\n\nconst valid = validate({ name: 'John Doe', age: 30 });\nconsole.log('Valid:', valid); // Valid: true\nconst invalid = validate({ name: 'John Doe' });\nconsole.log('Valid:', invalid); // Valid: false"}
Error Reporting
This feature enhances validation by including detailed error reports when validation fails. The example shows how to enable error reporting and how to access the errors after a validation attempt.
{"const validator = require('@exodus/schemasafe').validator;\nconst schema = {\n type: 'object',\n properties: {\n name: { type: 'string' },\n age: { type: 'number', minimum: 18 }\n },\n required: ['name', 'age']\n};\n\nconst validate = validator(schema, { includeErrors: true });\n\nconst result = validate({ name: 'John Doe', age: 17 });\nif (!result) {\n console.log('Validation errors:', validate.errors);\n}"}
Ajv is a popular JSON schema validator that supports JSON Schema drafts 04, 06, 07, and 2019-09. It is known for its performance and extensive feature set, including custom keywords and asynchronous validation. Compared to @exodus/schemasafe, Ajv offers broader schema version support and additional features, but both aim to provide fast and secure JSON validation.
The jsonschema package is another validator that supports JSON Schema Draft-04 (with partial Draft-06 and Draft-07 support). It focuses on simplicity and compliance with the JSON Schema specification. While it may not offer the same performance optimizations as @exodus/schemasafe or Ajv, it is a solid choice for projects that prioritize strict adherence to the JSON Schema standards.
@exodus/schemasafe
A JSONSchema validator that uses code generation to be extremely fast.
npm install --save @exodus/schemasafe
Simply pass a schema to compile it
const { validator } = require('@exodus/schemasafe')
const validate = validator({
type: 'object',
required: ['hello'],
properties: {
hello: {
type: 'string'
}
}
})
console.log('should be valid', validate({ hello: 'world' }))
console.log('should not be valid', validate({}))
// get the last list of errors by checking validate.errors
// the following will print [{field: 'data.hello', message: 'is required'}]
console.log(validate.errors)
@exodus/schemasafe
supports the formats specified in JSON schema v4 (such as date-time).
If you want to add your own custom formats pass them as the formats options to the validator
const validate = validator({
type: 'string',
format: 'only-a'
}, {
formats: {
'only-a': /^a+$/
}
})
console.log(validate('aa')) // true
console.log(validate('ab')) // false
You can pass in external schemas that you reference using the $ref
attribute as the schemas
option
const ext = {
type: 'string'
}
const schema = {
$ref: 'ext#' // references another schema called ext
}
// pass the external schemas as an option
const validate = validator(schema, { schemas: { ext: ext }})
console.log(validate('hello')) // true
console.log(validate(42)) // false
When the verbose
options is set to true
, @exodus/schemasafe
also outputs:
value
: The data value that caused the errorschemaPath
: a JSON pointer string as an URI fragment indicating which sub-schema failed, e.g. #/type
const schema = {
type: 'object',
required: ['hello'],
properties: {
hello: {
type: 'string'
}
}
}
const validate = validator(schema, {
includeErrors: true,
verboseErrors: true
})
validate({ hello: 100 });
console.log(validate.errors)
// [ { field: 'data["hello"]',
// message: 'is the wrong type',
// type: 'string',
// schemaPath: '#/properties/hello',
// value: 100 } ]
To compile a validator function to an IIFE, call validate.toModule()
:
const { validator } = require('@exodus/schemasafe')
const schema = {
type: 'string',
format: 'hex'
}
// This works with custom formats as well.
const formats = {
hex: (value) => /^0x[0-9A-Fa-f]*$/.test(value),
}
const validate = validator(schema, { formats })
console.log(validate.toModule())
/** Prints:
* (function() {
* const format0 = (value) => /^0x[0-9A-Fa-f]*$/.test(value);
* return (function validate(data) {
* if (data === undefined) data = null
* let errors = 0
* if (!(typeof data === "string")) {
* return false
* } else {
* if (!format0(data)) {
* return false
* }
* }
* return errors === 0
* })})();
*/
@exodus/schemasafe
uses code generation to turn a JSON schema into javascript code that is easily optimizeable by v8.
This is based on a heavily rewritten version of the amazing (but outdated) is-my-json-valid by @mafintosh.
Compared to is-my-json-valid
, @exodus/schemasafe
adds security-first design, many new features,
newer spec versions support, slimmer and more maintainable code, 0 dependencies, self-contained JS
module generation, fixes bugs and adds better test coverage, and drops support for outdated Node.js
versions.
MIT
FAQs
JSON Safe Parser & Schema Validator
The npm package @exodus/schemasafe receives a total of 334,107 weekly downloads. As such, @exodus/schemasafe popularity was classified as popular.
We found that @exodus/schemasafe demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 89 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.