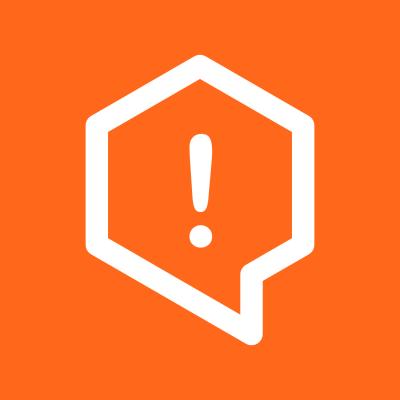
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@expo/config
Advanced tools
@expo/config is a package that provides utilities for reading, writing, and modifying Expo configuration files. It is particularly useful for managing app.json and app.config.js files in Expo projects.
Reading Configuration
This feature allows you to read the configuration of an Expo project. The `getConfig` function reads the app.json or app.config.js file and returns the configuration object.
const { getConfig } = require('@expo/config');
const config = getConfig('/path/to/project');
console.log(config);
Modifying Configuration
This feature allows you to modify the configuration of an Expo project. The `modifyConfigAsync` function takes the path to the project and an object with the modifications you want to make.
const { modifyConfigAsync } = require('@expo/config');
(async () => {
const result = await modifyConfigAsync('/path/to/project', {
updates: {
enabled: true
}
});
console.log(result);
})();
Writing Configuration
This feature allows you to write a new configuration to the app.json file. The `writeConfigJsonAsync` function takes the path to the project and the new configuration object.
const { writeConfigJsonAsync } = require('@expo/config');
(async () => {
const result = await writeConfigJsonAsync('/path/to/project', {
name: 'NewAppName',
slug: 'new-app-slug'
});
console.log(result);
})();
Cosmiconfig is a popular package for finding and loading configuration files in various formats (JSON, YAML, JS, etc.). It is more general-purpose compared to @expo/config, which is specifically tailored for Expo projects.
The 'config' package is a configuration manager for Node.js applications. It allows you to define a set of default parameters and override them with environment-specific values. Unlike @expo/config, it is not specific to Expo projects and can be used in any Node.js application.
The 'rc' package is a non-opinionated configuration loader for Node.js applications. It loads configuration options from a variety of sources, including command-line arguments, environment variables, and configuration files. It is more flexible but less specialized than @expo/config.
FAQs
A library for interacting with the app.json
The npm package @expo/config receives a total of 1,162,097 weekly downloads. As such, @expo/config popularity was classified as popular.
We found that @expo/config demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 28 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.