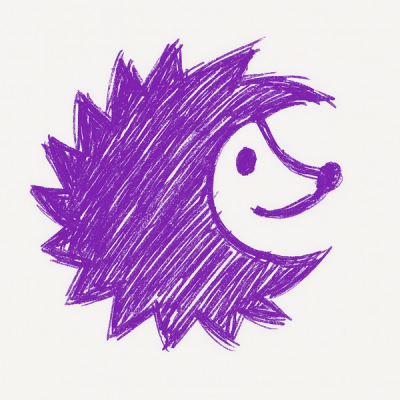
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
@expo/json-file
Advanced tools
The @expo/json-file npm package is a utility library for reading and writing JSON files. It provides a simple and convenient API for these operations, handling various edge cases and providing helpful error messages.
Read JSON file
This feature allows you to read a JSON file asynchronously and parse its contents into a JavaScript object.
{"const { readAsync } = require('@expo/json-file');
async function readJsonFile(filePath) {
const json = await readAsync(filePath);
return json;
}
readJsonFile('path/to/file.json').then(data => console.log(data));"}
Write JSON file
This feature enables you to write a JavaScript object to a file as JSON asynchronously, with options to format the output.
{"const { writeAsync } = require('@expo/json-file');
async function writeJsonFile(filePath, data) {
await writeAsync(filePath, data);
}
const data = { key: 'value' };
writeJsonFile('path/to/file.json', data);"}
Modify JSON file
This feature allows you to modify an existing JSON file by providing an object with the modifications you want to apply.
{"const { modifyAsync } = require('@expo/json-file');
async function modifyJsonFile(filePath, modifications) {
await modifyAsync(filePath, modifications);
}
modifyJsonFile('path/to/file.json', { key: 'newValue' });"}
The 'jsonfile' package offers similar functionality to '@expo/json-file' for reading and writing JSON files. It provides methods like 'readFileSync', 'writeFileSync', 'readFile', and 'writeFile'. The main difference is that 'jsonfile' does not provide modification capabilities directly, and it has both synchronous and asynchronous methods.
While 'fs-extra' is a more general file system utility library, it includes methods for reading and writing JSON files, such as 'readJson', 'writeJson', 'readJsonSync', and 'writeJsonSync'. It extends the built-in 'fs' module in Node.js and provides additional file operation methods. It is more comprehensive but less specialized than '@expo/json-file'.
48.0.0 — 2023-02-09
@stripe/stripe-react-native
from 0.19.0
to 0.23.3
. (#20964 by @aleqsio, #21117 by @kudo)react-native-webview
from 11.23.1
to 11.26.0
. (#20933 by @aleqsio)react-native-gesture-handler
from 2.8.0
to 2.9.0
. (#20930 by @tsapeta)react-native-shared-element
from 0.8.4
to 0.8.7
. (#20593 by @ijzerenhein)@react-native-async-storage/async-storage
from 1.17.3
to 1.17.11
. (#20780 by @kudo)react-native-reanimated
from 2.12.0
to 2.14.4
. (#20798 by @kudo, #20990 by @tsapeta)@shopify/react-native-skia
from 0.1.157
to 0.1.172
. (#20857, #21014 by @kudo)react-native-safe-area-context
from 4.4.1
to 4.5.0
. (#20899 by @gabrieldonadel)react-native-screens
from 3.18.0
to 3.20.0
. (#20938 by @lukmccall, #21186 by @tsapeta)react-native-pager-view
from 6.0.1
to 6.1.2
. (#20932 by @gabrieldonadel)@react-native-community/slider
from 4.2.4
to 4.4.2
. (#20903 by @gabrieldonadel, #21055 by @kudo)react-native-shared-element
from 0.8.7
to 0.8.8
. (#20929 by @byCedric)@react-native-community/datetimepicker
from 6.5.2
to 6.7.3
. (#20926 by @byCedric)@shopify/flash-list
from 1.3.1
to 1.4.0
. (#20927 by @lukmccall)expo-contacts
expo-keep-awake
KeepAwake.activateKeepAwake
has been deprecated in favor of KeepAwake.activateKeepAwakeAsync
. (#15826 by @EvanBacon)expo-linking
expo-apple-authentication
expo-av
expo-barcode-scanner
expo-blur
expo-brightness
expo-constants
expo-crypto
randomUUID
method to get a random UUIDv4 string. (#20274 by @aleqsio)getRandomValues
method to fill typed arrays. (#20257 by @aleqsio)getRandomBytes
, getRandomBytesAsync
methods from expo-random
. (#20217 by @aleqsio)digest
method to get a cryptographic digest of a typed array. (#20886 by @aleqsio)expo-device
expo-document-picker
expo-gl
expo-haptics
expo-keep-awake
KeepAwake.isAvailableAsync
which returns false on certain web browsers. (#15826 by @EvanBacon)KeepAwake.addListener
to observe state changes on web. (#15826 by @EvanBacon)expo-intent-launcher
expo-local-authentication
expo-image-picker
expo-media-library
expo-modules-core
TypedArray
and additional union types for Int, Uint and Float TypedArrays. (#20257 by @aleqsio)executeOnJavaScriptThread
method to appContext
to allow for running code blocks on the JS thread. (#20161 by @aleqsio)Exceptions.MissingActivity
on Android. (#20174 by @lukmccall)Events
component can now be initialized with an array of event names (not only variadic arguments). (#20590 by @tsapeta)Property
component can now take the native shared object instance as the first argument. (#20608 by @tsapeta)Property
's owner properties using Swift key paths. (#20610 by @tsapeta)Long
type as function parameters on Android. (#20787 by @lukmccall)expo-network
expo-sharing
expo-sms
expo-store-review
expo-speech
expo-video-thumbnails
expo-asset
/
. (#20258 by @EvanBacon)expo-av
HTMLMediaElement.play
and HTMLMediaElement.pause
calls on the Web aren't properly awaited. (#20439) by @zhigang1992 (#20439 by @zhigang1992)SimpleExoPlayer
implementation on Android. (#21055 by @kudo)expo-barcode-scanner
expo-blur
-webkit-backdrop-filter
to support blurring on Safari. (#21003 by @EvanBacon)expo-clipboard
ImageFormat
or the StringFormat
not working in the release builds on Android. (#20155 by @lukmccall)expo-constants
expo-camera
Cannot set prop 'barCodeScannerSettings' on view 'class expo.modules.camera.ExpoCameraView'
on Android. (#21033 by @lukmccall)expo-file-system
expo-gl
expo-haptics
expo-image-picker
expo-mail-composer
composeAsync
not resolving promise after sending/ discarding email. (#20869 by @keith-kurak)expo-media-library
expo-modules-core
new NativeEventEmitter() was called with a non-null argument without the required addListener method.
warnings on Android with JSC. (#19920 by @kudo)ViewDefinitionBuilder
crashes when ProGuard or R8 is enabled on Android. (#20197 by @lukmccall)SharedObject
initializer being inaccessible due to internal
protection level. (#20588 by @tsapeta)null
or undefined
. (#20755 & #20766 by @tsapeta & @lukmccall) (#20755, #20766 by @tsapeta, @lukmccall)expo-location
isolatedModules
flag. (#20239 by @zakharchenkoAndrii)expo-print
printAsync
not reflecting custom width/ height, useMarkupFormatter
option preventing custom width/ height/ margin from being reflected. (#18873 by @keith-kurak) (#20046 by)expo-sensors
isolatedModules
flag. (#20239 by @zakharchenkoAndrii)expo-web-browser
url
for web. (#20708 by @EvanBacon)expo-asset
expo-application
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-auth-session
expo-random
package. (#21063 by @lukmccall)expo-background-fetch
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-av
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-barcode-scanner
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-branch
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-brightness
useSystemBrightnessAsync
and add it as renamed restoreSystemBrightnessAsync
method to avoid violating Rules of Hooks. (#19701 by @Simek)compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-cellular
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-calendar
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-clipboard
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-constants
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-contacts
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-crypto
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-battery
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-device
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-document-picker
uuid
. (#20477 by @LinusU)compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-camera
videoStabilizationMode
option. (#20130 by @simek)compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-error-recovery
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-file-system
DeletingOptions
, InfoOptions
, RelocatingOptions
and MakeDirectoryOptions
types. (#20103 by @Simek)UploadProgressData
totalByteSent
field to totalBytesSent
. (#20804 by @gabrieldonadel)compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-font
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-face-detector
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-gl
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-haptics
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-image-manipulator
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-image-loader
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-keep-awake
KeepAwakeOptions
type, update the doc comments. (#20489 by @Simek)compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-linear-gradient
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-intent-launcher
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-local-authentication
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-linking
expo-image-picker
uuid
. (#20476 by @LinusU)compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-localization
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-mail-composer
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-media-library
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-modules-core
ArgumentCastException
to use ordinal numbers. (#19912 by @tsapeta)Enumerable
protocol implement CaseIterable
to get rid of operating on unsafe pointers. (#20640 by @tsapeta)compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-location
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-network
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-print
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-random
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-permissions
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-screen-capture
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-notifications
getExpoPushTokenAsync
to make projectId
required. (#20833 by @gabrieldonadel)compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)getExpoPushTokenAsync
parameter type. (#21104 by @Simek)expo-sharing
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-screen-orientation
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-sms
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-sensors
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-store-review
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-secure-store
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-speech
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-sqlite
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-task-manager
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-video-thumbnails
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)expo-web-browser
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)unimodules-app-loader
compileSdkVersion
and targetSdkVersion
to 33
. (#20721 by @lukmccall)FAQs
A module for reading, writing, and manipulating JSON files
The npm package @expo/json-file receives a total of 2,752,440 weekly downloads. As such, @expo/json-file popularity was classified as popular.
We found that @expo/json-file demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 28 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.