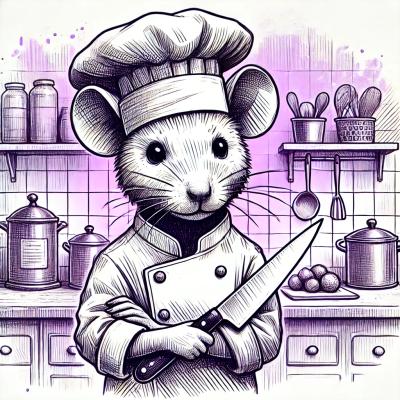
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@fablevision/interaction
Advanced tools
Accessible interactivity designed for Canvas-based (Pixi, Phaser, EaselJS, etc) games.
Accessible interactivity designed for Canvas-based (Pixi, Phaser, EaselJS, etc) games.
Both Keyboard and InteractionManager use stacks of interaction contexts, so that interactivity can be quickly superseded and then restored
for things like popups or items that are grouped in the UI for keyboard controls. You'll be expected to use activateContext()
with a context name
in order to enable interactivity, and then popContext()
to remove it when you are done. Listeners all use the Disposable pattern, so return an object that has a dispose()
method for cleanup.
The Keyboard class is built upon KeyboardJS, so uses key names/combinations as documented there.
// initialize singleton
const keyboard = new Keyboard();
// elsewhere in code
const removeListener = Keyboard.instance.add('ctrl + s', () => save());
The main classes can be imported with import { InteractionManager, Keyboard } from '@fablevision/interaction';
, but each plugin handler should be imported like so: import { PhaserHandler } from '@fablevision/interaction/dist/phaser';
Current plugin support: Pixi, Phaser 3
// keyboard must be created first
const keyboard = new Keyboard();
// initialize singleton
// note that this should be done AFTER the constructor for something like Phaser, as when setting up the Handler this.game isn't generated until after construction
const interaction = new InteractionManager({
// This can be the id of a div, or the HTMLDivElement directly, and will be filled with interactive divs.
// *You* are responsible for ensuring that the div is the same size/scale/location as your canvas.
// Note that you may need to keep it from scrolling, if elements are sometimes partially offscreen (but still focusable). You may also wish to use `user-select: none;` on it to prevent the text/image selection tinting.
accessiblityDiv: 'interaction',
// a handler specific to your canvas rendering style
renderer: new PixiHandler(myPixiRenderer),
});
// manually enable interaction manager (there will be no input handling otherwise)
interaction.enabled = true;
// elsewhere in code
// use the interactive class specific to your canvas rendering style
const buttonInteractive = new PixiInteractive({
pixi: myPixiButton,
});
buttonInteractive.onActivate.on(() => doTheThing());
InteractionManager.instance.activateContext({items: [buttonInteractive], name: 'MyContext'});
FAQs
Accessible interactivity designed for Canvas-based (Pixi, Phaser, EaselJS, etc) games.
The npm package @fablevision/interaction receives a total of 2 weekly downloads. As such, @fablevision/interaction popularity was classified as not popular.
We found that @fablevision/interaction demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.