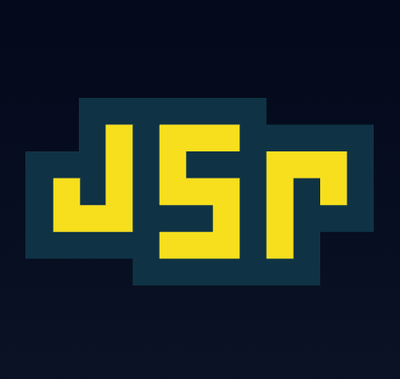
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@faker-js/faker
Advanced tools
The @faker-js/faker package is a powerful tool for generating massive amounts of fake (but realistic) data for various purposes such as testing, bootstrapping databases, and creating rich prototypes. It can create data for names, addresses, phone numbers, and much more.
Basic random data generation
Generate random names, addresses, and other basic personal information.
const { faker } = require('@faker-js/faker');
console.log(faker.name.findName()); // Generates a random name
Localization
Support for localized data, allowing generation of data that is locale-specific.
const { faker } = require('@faker-js/faker');
faker.locale = 'de';
console.log(faker.name.findName()); // Generates a random name with German locale
Random numbers
Create random numbers, including options for specifying ranges and precision.
const { faker } = require('@faker-js/faker');
console.log(faker.datatype.number()); // Generates a random number
Date and time
Generate random dates and times, with the ability to specify past or future dates.
const { faker } = require('@faker-js/faker');
console.log(faker.date.future()); // Generates a random future date
Internet-related data
Create fake internet data such as email addresses, domain names, and URLs.
const { faker } = require('@faker-js/faker');
console.log(faker.internet.email()); // Generates a random email address
Commerce data
Generate commerce-related data like product names, prices, and categories.
const { faker } = require('@faker-js/faker');
console.log(faker.commerce.productName()); // Generates a random product name
Images
Create URLs for random placeholder images.
const { faker } = require('@faker-js/faker');
console.log(faker.image.imageUrl()); // Generates a random image URL
Chance is a minimalist generator of random strings, numbers, etc. to help reduce some monotony particularly while writing automated tests or anywhere else you need anything random.
Casual is a fake data generator for JavaScript which is more lightweight than @faker-js/faker and provides an easy-to-use API with a smaller set of functionalities.
Randexp generates strings that match a given regular expression which is useful for creating random data that fits a specific pattern, unlike @faker-js/faker which provides a wide range of predefined data types.
Please proceed to the Getting Started Guide for the stable release of Faker.
For detailed API documentation, please select the version of the documentation you are looking for.
Version | Website |
---|---|
v9 (next) | https://next.fakerjs.dev/ |
v8 (stable) | https://fakerjs.dev/ |
v7 (old) | https://v7.fakerjs.dev/ |
Note: Faker tries to generate realistic data and not obvious fake data. The generated names, addresses, emails, phone numbers, and/or other data might be coincidentally valid information. Please do not send any of your messages/calls to them from your test setup.
npm install --save-dev @faker-js/faker
// ESM
import { faker } from '@faker-js/faker';
// CJS
const { faker } = require('@faker-js/faker');
export function createRandomUser(): User {
return {
userId: faker.string.uuid(),
username: faker.internet.userName(),
email: faker.internet.email(),
avatar: faker.image.avatar(),
password: faker.internet.password(),
birthdate: faker.date.birthdate(),
registeredAt: faker.date.past(),
};
}
export const USERS: User[] = faker.helpers.multiple(createRandomUser, {
count: 5,
});
An in-depth overview of the API methods is available in the documentation for v8 (stable) and v8.* (next).
Faker contains a generator method faker.helpers.fake
for combining faker API methods using a mustache string format.
console.log(
faker.helpers.fake(
'Hello {{person.prefix}} {{person.lastName}}, how are you today?'
)
);
Faker has support for multiple locales.
The main faker
instance uses the English locale.
But you can also import instances using other locales.
// ESM
import { fakerDE as faker } from '@faker-js/faker';
// CJS
const { fakerDE: faker } = require('@faker-js/faker');
See our documentation for a list of provided languages.
Please note: Not every locale provides data for every module. In our pre-made faker instances, we fall back to English in such a case as this is the most complete and most commonly used language. If you don't want that or prefer a different fallback, you can also build your own instances.
import { de, de_CH, Faker } from '@faker-js/faker';
export const faker = new Faker({
locale: [de_CH, de],
});
If you want consistent results, you can set your own seed:
faker.seed(123);
const firstRandom = faker.number.int();
// Setting the seed again resets the sequence.
faker.seed(123);
const secondRandom = faker.number.int();
console.log(firstRandom === secondRandom);
Faker is an MIT-licensed open source project with its ongoing development made possible entirely by the support of these awesome backers
Please make sure to read the Contributing Guide before making a pull request.
Thanks to all the people who already contributed to Faker!
The fakerjs.dev website is kindly hosted by the Netlify Team. Also the search functionality is powered by algolia.
Detailed changes for each release are documented in the release notes.
Read the team update (January 14th, 2022).
9.0.0-alpha.0 (2024-04-12)
multiple
(#2563)multiple
(#2563) (2b15f2e)commerce: remove v8 deprecated commerce method (#2752) (8c80877)
company: remove v8 deprecated company methods (#2726) (19bcf88)
datatype: remove v8 deprecated datatype methods (#2694) (682a427)
finance: remove v8 deprecated finance methods (#2727) (a536a9d)
helpers: remove v8 deprecated helpers methods (#2729) (1169a05)
internet: remove v8 deprecated internet methods (#2699) (3024d9e)
location: remove default_country definitions (#2740) (a409b46)
location: remove v8 deprecated location methods (#2753) (6dee178)
number: remove v8 deprecated number parameter (#2738) (a672d27)
random: remove v8 deprecated image methods (#2697) (e99fba9)
FAQs
Generate massive amounts of fake contextual data
The npm package @faker-js/faker receives a total of 4,252,704 weekly downloads. As such, @faker-js/faker popularity was classified as popular.
We found that @faker-js/faker demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.