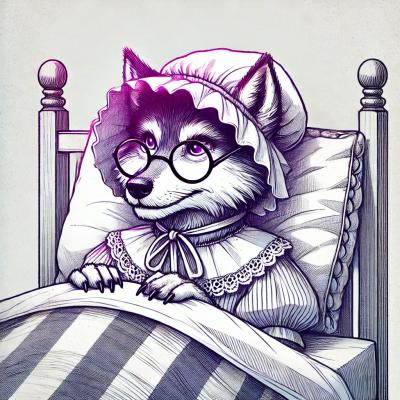
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@fast-csv/format
Advanced tools
The @fast-csv/format package is a powerful, fast, and flexible library for formatting CSV data in Node.js. It allows users to easily convert arrays or streams of objects into CSV format, supporting complex features like custom headers, transforming data, and more.
Formatting an array of objects to CSV
This feature allows you to easily convert an array of objects into a CSV string. The example demonstrates how to use the `writeToString` method to convert an array of objects into a CSV format string, including headers.
const { writeToString } = require('@fast-csv/format');
const rows = [
{ id: 'A1', name: 'John Doe' },
{ id: 'A2', name: 'Jane Doe' }
];
writeToString(rows, { headers: true })
.then(csvString => console.log(csvString));
Streaming data to CSV
This feature demonstrates how to stream data to a CSV file. It uses the `format` function to create a stream that formats objects into CSV format and pipes the output to a file. This is useful for handling large datasets or real-time data processing.
const { createWriteStream } = require('fs');
const { format } = require('@fast-csv/format');
const stream = format({ headers: true });
stream.pipe(createWriteStream('path/to/file.csv'));
stream.write({ id: 'A1', name: 'John Doe' });
stream.write({ id: 'A2', name: 'Jane Doe' });
stream.end();
csv-writer is another npm package for generating CSV files. It provides a similar functionality to @fast-csv/format, allowing for the creation of CSV files from arrays or objects. However, @fast-csv/format offers more flexibility in terms of streaming and transforming data before writing.
Papa Parse is a comprehensive CSV library that can parse CSV files or strings into JSON and vice versa. While it is more known for its parsing capabilities, it also supports CSV writing. Compared to @fast-csv/format, Papa Parse offers a wider range of parsing features but might not match the specific formatting and streaming capabilities of @fast-csv/format.
@fast-csv/format
fast-csv
package to create CSVs.
npm i -S @fast-csv/format
To use fast-csv
in javascript
you can require the module
const csv = require('@fast-csv/format');
To import with typescript
import * as format csv '@fast-csv/format';
delimiter: {string} = ','
: Specify an alternate field delimiter such as ;
or \t
.
delimiter
you may only pass in a single character delimiterrowDelimiter: {string} = '\n'
: Specify an alternate row delimiter (i.e \r\n
)quote: {string|boolean} = '"'
:
"first,name",last name
quote
is set to true
the default quote will be used.
quote
false then quoting will be disabled
escape: {string} = '"'
: The character to use when escaping a value that is quoted
and contains a quote
character that is not the end of the field.
i.e
: First,"Name"' => '"First,""Name"""
includeEndRowDelimiter: {boolean} = false
: Set to true
to include a row delimiter at the end of the csv.writeBOM: {boolean} = false
: Set to true if you want the first character written to the stream to be a utf-8 BOM character.headers: {null|boolean|string[]} = null
:
[ ['header', 'column'], ['header2', 'column2'] ]
) then the first element in each array will be used.string[]
alwaysWriteHeaders: {boolean} = false
: Set to true if you always want headers written, even if no rows are written.
quoteColumns: {boolean|boolean[]|{[string]: boolean} = false
true
then columns and headers will be quoted (unless quoteHeaders
is specified).quoteHeaders
is specified)quoteHeaders
is specified)quoteHeaders
is not specified this option will apply to both columns and headers.quoteHeaders: {boolean|boolean[]|{[string]: boolean} = quoteColumns
quoteColumns
option.true
then all headers will be quoted.transform: {(row) => Row | (row, cb) => void} = null
: A function that accepts a row and returns a transformed one to be written, or your function can accept an optional callback to do async transformations.When creating a CSV fast-csv
supports a few row formats.
{[string]: any}
You can pass in object to any formatter function if your CSV requires headers the keys of the first object will be used as the header names.
{
a: "a1",
b: "b1",
c: "c1",
}
//Generated CSV
//a,b,c
//a1,b1,c1
string[]
You can also pass in your rows as arrays. If your CSV requires headers the first row passed in will be the headers used.
[
["a", "b", "c"],
["a1", "b1", "c1"]
]
//Generated CSV
//a,b,c
//a1,b1,c1
[string, any][]
This is the least commonly used format but can be useful if you have requirements to generate a CSV with headers with the same column name (Crazy we know but we have seen it).
[
[
["a", "a1"],
["a", "a2"],
["b", "b1"],
["b", "b2"],
["c", "c1"],
["c", "c2"]
]
]
//Generated CSV
//a,a,b,b,c,c
//a1,a2,b1,b2,c1,c2
csv.format(options): CsvFormatterStream
This is the main entry point for formatting CSVs. It is used by all other helper methods.
const stream = csv.format();
stream.pipe(process.stdout);
stream.write([ 'a', 'b' ]);
stream.write([ 'a1', 'b1' ]);
stream.write([ 'a2', 'b2' ]);
stream.end();
Expected output
a,b
a1,b1
a2,b2
write(rows[, options]): CsvFormatterStream
Create a formatter, writes the rows and returns the CsvFormatterStream
.
const rows = [
[ 'a', 'b' ],
[ 'a1', 'b1' ],
[ 'a2', 'b2' ],
];
csv.write(rows).pipe(process.stdout);
Expected output
a,b
a1,b1
a2,b2
writeToStream(stream, rows[, options])
Write an array of values to a WritableStream
, and returns the original stream
const rows = [
[ 'a', 'b' ],
[ 'a1', 'b1' ],
[ 'a2', 'b2' ],
];
csv.writeToStream(process.stdout, rows);
Expected output
a,b
a1,b1
a2,b2
writeToPath(path, rows[, options])
Write an array of values to the specified path
const rows = [
[ 'a', 'b' ],
[ 'a1', 'b1' ],
[ 'a2', 'b2' ],
];
csv.writeToPath(path.resolve(__dirname, 'tmp.csv'), rows)
.on('error', err => console.error(err))
.on('finish', () => console.log('Done writing.'));
Expected file content
a,b
a1,b1
a2,b2
writeToString(arr[, options]): Promise<string>
Formats the rows and returns a Promise
that will resolve with the CSV content as a string
.
const rows = [
[ 'a', 'b' ],
[ 'a1', 'b1' ],
[ 'a2', 'b2' ],
];
csv.writeToString(rows).then(data => console.log(data));
writeToBuffer(arr[, options]): Promise<Buffer>
Formats the rows and returns a Promise
that will resolve with the CSV content as a Buffer
.
const rows = [
[ 'a', 'b' ],
[ 'a1', 'b1' ],
[ 'a2', 'b2' ],
];
csv.writeToBuffer(rows).then(data => console.log(data.toString()));
FAQs
fast-csv formatting module
The npm package @fast-csv/format receives a total of 1,336,750 weekly downloads. As such, @fast-csv/format popularity was classified as popular.
We found that @fast-csv/format demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.