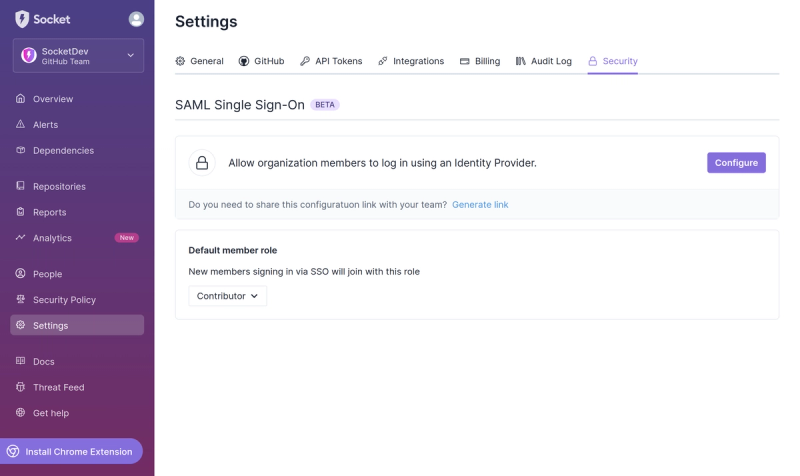
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
@fastify/accepts
Advanced tools
Readme
Add accepts parser to fastify
npm i @fastify/accepts
const fastify = require('fastify')
const Boom = require('@hapi/boom')
fastify.register(require('@fastify/accepts'))
fastify.post('/', function (req, reply) {
const accept = req.accepts() // Accepts object
switch(accept.type(['json', 'html'])) {
case 'json':
reply.type('application/json').send({hello: 'world'})
break
case 'html':
reply.type('text/html').send('<b>hello, world!</b>')
break
default:
reply.send(Boom.notAcceptable('unacceptable'))
break
}
})
See accepts package for all available APIs.
This plugin adds to Request
object all Accepts
object methods.
fastify.post('/', function (req, reply) {
req.charset(['utf-8'])
req.charsets()
req.encoding(['gzip', 'compress'])
req.encodings()
req.language(['es', 'en'])
req.languages()
req.type(['image/png', 'image/tiff'])
req.types()
})
Licensed under MIT
FAQs
Add accept parser to fastify
The npm package @fastify/accepts receives a total of 104,827 weekly downloads. As such, @fastify/accepts popularity was classified as popular.
We found that @fastify/accepts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 20 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.