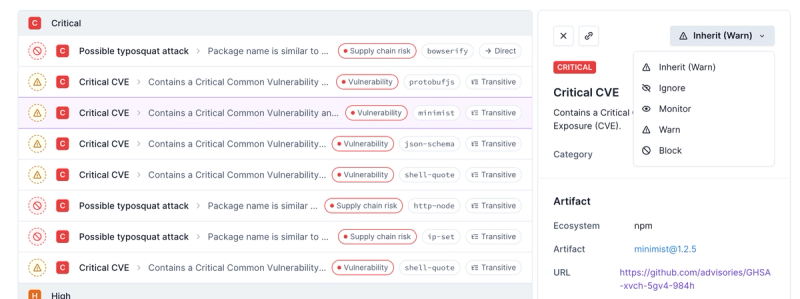
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
@fishawack/lab-d3
Advanced tools
Readme
Reusable chart visualizations where dynamic data consistent data structures are key.
Prevent code repetition.
npm install @fishawack/lab-d3
Add a color list variable to your defaults.scss
which is used to generate the colors for all charts.
$colors-list: $color1 $color2 $color3 $color4;
Import the base lab-d3 style code in your general.scss.
@import "@fishawack/lab-d3/_Build/sass/components/_chart.scss";
Add a html tag that the script can attach onto. The labD3 parent is used for positioning.
<div class="labD3">
<svg id="chart--bar"></svg>
</div>
Initialize the chart with the selector from the html above.
import { Bar } from "@fishawack/lab-d3";
var myBar = new Bar('#chart--bar')
.init()
.data([
{"value": 10},
{"value": 20}
])
.render();
HTML rich text isn't supported in the charts (due to IE not supporting foreignObject tag) so if you need superscript you'll need to use the following characters as a compromise but please check with the writer/editor that this is acceptable as it's almost always easier to move the references off the charts and put them elsewhere.
⁰¹²³⁴⁵⁶⁷⁸⁹
ᵃᵇᶜᵈᵉᶠᵍʰⁱʲᵏˡᵐⁿᵒᵖʳˢᵗᵘᵛʷˣʸᶻ
Resizing charts is a pretty deep subject, you might think.. this is svg, surely it resizes automatically? That is true, but being a chart that's drawn via javascript and needs maths to calculate positions its not as simple as it looks. I'll go over the 2 main ways to do resizing and the ups and downsides of each
This may seem finicky, but this is the suggested route to take. By listening to the window resize event and calling .resize().renderSync() on the chart object we can recalculate the underlying maths instantly.
window.onresize = function() {
myBar.resize()
.renderSync();
};
The main up side to this approach is all font sizes will be maintained. This means even if the screen is now half the size, the text will still be 16px rather than scaling down to 8px.
By default charts will take their parents width and use their aspect ratio to size themselves. You can however pass in a hard coded width and/or height. This basically makes it so that when the chart is at that width it will appear as intended. If the screen grows or shrinks then the chart will scale up and down relative to the hardcoded width
myBar.att({
width: 400
})
.init()
.render();
Use this if its a fixed width site as it removes the need for event handlers. This method also makes charts work more like images where the text in the chart will shrink relative to the width compared to its hardcoded width.
{
colors : ["fill1", "fill2", "fill3", "fill4"],
colorsKey : "key",
minmaxKey : "key",
aspectRatio : 0.5625,
width: 0,
height: 0,
transitionType: "CubicInOut",
transitionSpeed: 800,
delaySpeed : 0,
stagger : 800,
startOpacity: 0,
hide: {},
min: {
x: null,
y: null
},
max: {
x: null,
y: null
},
minmax: 0.01,
label: {
x: null,
y: null
},
labelWidth: "auto",
roundPoints: false,
cb: null,
plot: {
x: "key",
y: "value",
value: "value",
label: "label"
},
tooltip: {
},
totalCount: 100,
value: {
structure: "{value}",
decimal: 0,
html: false,
format: {
value: ".0f",
percent: ".0f",
total: ".0f"
},
offset: {
ratio: false,
y: 0,
x: 0
}
},
chockData: false
}
{
margin : {ratio: false, top: 10, right: 10, bottom: 10, left: 10},
padding : {ratio: false, outer: 0, inner: 0, space: 10},
axis: {
x: {
ratio: false,
flip: false,
reverse: false,
rotate: false,
inside: false,
hide: false,
ticks: 5,
structure: null,
format: {
value: null,
percent: ".0f",
total: ".0f"
},
tickValues: null,
tickSizeInner: null,
tickSizeOuter: null
},
y: {
ratio: false,
flip: false,
reverse: false,
rotate: false,
inside: false,
hide: false,
ticks: 5,
structure: null,
format: {
value: null,
percent: ".0f",
total: ".0f"
},
tickValues: null,
tickSizeInner: null,
tickSizeOuter: null
}
},
scale: {
x: "band",
y: "linear"
},
autoAxis: "x",
parseDate: d3.timeParse("%Y-%m-%d"),
formatDate: d3.timeFormat("%Y-%m-%d"),
primaryIndex: null,
symbols: ["Cross"],
symbolColors : ["fill2"],
symbolsSize: 100,
symbolsRatio: false,
symbolsKey : "key",
symbolColorsKey : "key",
radius: {
top: {
left: 4,
right: 4
},
bottom: {
left: 4,
right: 4
}
},
inject: {}
}
FAQs
Abstract layer built on top of d3
The npm package @fishawack/lab-d3 receives a total of 165 weekly downloads. As such, @fishawack/lab-d3 popularity was classified as not popular.
We found that @fishawack/lab-d3 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.