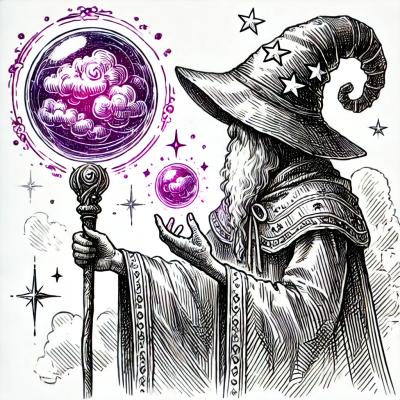
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@fluentui/react-theme
Advanced tools
@fluentui/react-theme is a package that provides theming capabilities for Fluent UI React components. It allows developers to define and apply custom themes to their applications, ensuring a consistent look and feel across all components.
Custom Theme Creation
This feature allows you to create a custom theme by defining a palette of colors. The `createTheme` function takes an object with color definitions and returns a theme object that can be applied to Fluent UI components.
const { createTheme } = require('@fluentui/react-theme');
const myCustomTheme = createTheme({
palette: {
themePrimary: '#0078d4',
themeLighterAlt: '#eff6fc',
themeLighter: '#deecf9',
themeLight: '#c7e0f4',
themeTertiary: '#71afe5',
themeSecondary: '#2b88d8',
themeDarkAlt: '#106ebe',
themeDark: '#005a9e',
themeDarker: '#004578',
neutralLighterAlt: '#faf9f8',
neutralLighter: '#f3f2f1',
neutralLight: '#edebe9',
neutralQuaternaryAlt: '#e1dfdd',
neutralQuaternary: '#d0d0d0',
neutralTertiaryAlt: '#c8c6c4',
neutralTertiary: '#a19f9d',
neutralSecondary: '#605e5c',
neutralPrimaryAlt: '#3b3a39',
neutralPrimary: '#323130',
neutralDark: '#201f1e',
black: '#000000',
white: '#ffffff'
}
});
Applying a Theme
This feature allows you to apply a custom theme to your application using the `ThemeProvider` component. By wrapping your application or specific components with `ThemeProvider` and passing the custom theme, you ensure that the theme is applied consistently.
const { ThemeProvider } = require('@fluentui/react-theme-provider');
const { myCustomTheme } = require('./myCustomTheme');
const App = () => (
<ThemeProvider theme={myCustomTheme}>
<YourComponent />
</ThemeProvider>
);
Using Theme Tokens
This feature allows you to access the current theme within your components using the `useTheme` hook. You can then use the theme tokens, such as colors, to style your components dynamically based on the active theme.
const { useTheme } = require('@fluentui/react-theme');
const ThemedComponent = () => {
const theme = useTheme();
return (
<div style={{ backgroundColor: theme.palette.themePrimary }}>
Themed Content
</div>
);
};
styled-components is a popular library for styling React applications using tagged template literals. It allows for defining component-level styles and supports theming through a ThemeProvider. Compared to @fluentui/react-theme, styled-components offers more flexibility in defining styles but does not come with a predefined set of UI components.
Emotion is a performant and flexible CSS-in-JS library that allows for writing styles with JavaScript. It supports theming through a ThemeProvider and offers a similar API to styled-components. Emotion is more focused on providing low-level styling capabilities, whereas @fluentui/react-theme is tailored for theming Fluent UI components.
Material-UI is a popular React UI framework that follows Google's Material Design guidelines. It provides a comprehensive set of components and theming capabilities. While @fluentui/react-theme is specific to Fluent UI components, Material-UI offers a broader range of components and a different design language.
React Theme for Fluent UI React
Import a theme and tokens:
import { webLightTheme, tokens } from '@fluentui/react-components';
Pass the theme to the FluentProvider
:
<FluentProvider theme={webLightTheme}>
<App />
</FluentProvider>
Use the tokens
in your styles:
const useStyles = makeStyles({
root: {
color: tokens.colorNeutralForeground1,
},
});
To use a theme based on a custom brand ramp, use the createXXXTheme
function:
import { createWebLightTheme } from '@fluentui/react-components';
const customBrandRamp: BrandVariants = {
10: `#2b2b40`,
// ...
160: `#e8ebfa`,
};
const customTheme = createWebLightTheme(customBrandRamp);
The color tokens are generated by token pipeline. Do not edit colors directly. Instead, update the token pipeline first and once the changes are merged there, run:
yarn token-pipeline
To add a new color token, besides updating the token pipeline, you also need to update corresponding typings and the tokens
object in src/tokens.ts
.
To update any tokens not related to colors, update the values directly in this package.
FAQs
Fluent UI themes
The npm package @fluentui/react-theme receives a total of 100,526 weekly downloads. As such, @fluentui/react-theme popularity was classified as popular.
We found that @fluentui/react-theme demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 12 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.