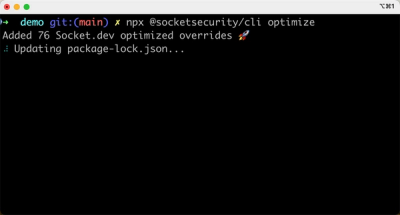
Product
Introducing Socket Optimize
We're excited to introduce Socket Dependency Optimization, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.
@fp-ts/optic
Advanced tools
A porting of zio-optics to TypeScript
flowchart TD
Optic --> Iso
Iso --> Lens
Iso --> Prism
Lens --> Optional
Prism --> Optional
Optional --> Getter
Optional --> Setter
interface Street {
num: number;
name: string;
}
interface Address {
city: string;
street: Street;
}
interface Company {
name: string;
address: Address;
}
interface Employee {
name: string;
company: Company;
}
Let's say we have an employee and we need to upper case the first character of his company street name. Here is how we could write it in vanilla TypeScript
const employee: Employee = {
name: "john",
company: {
name: "awesome inc",
address: {
city: "london",
street: {
num: 23,
name: "high street",
},
},
},
};
const capitalize = (s: string): string =>
s.substring(0, 1).toUpperCase() + s.substring(1);
const employeeCapitalized = {
...employee,
company: {
...employee.company,
address: {
...employee.company.address,
street: {
...employee.company.address.street,
name: capitalize(employee.company.address.street.name),
},
},
},
};
As we can see copy is not convenient to update nested objects because we need to repeat ourselves. Let's see what could we do with @fp-ts/optic
import * as Optic from "@fp-ts/optic";
const _name = Optic.id<Employee>()
.compose(Optic.key("company"))
.compose(Optic.key("address"))
.compose(Optic.key("street"))
.compose(Optic.key("name"));
const capitalizeName = Optic.modify(_name)(capitalize);
expect(capitalizeName(employee)).toEqual(employeeCapitalized);
To install the alpha version:
npm install @fp-ts/optic
The MIT License (MIT)
FAQs
Unknown package
The npm package @fp-ts/optic receives a total of 469 weekly downloads. As such, @fp-ts/optic popularity was classified as not popular.
We found that @fp-ts/optic demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
We're excited to introduce Socket Dependency Optimization, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.
Product
We're excited to announce that Socket now supports the Java programming language.
Security News
Socket detected a malicious Python package impersonating a popular browser cookie library to steal passwords, screenshots, webcam images, and Discord tokens.