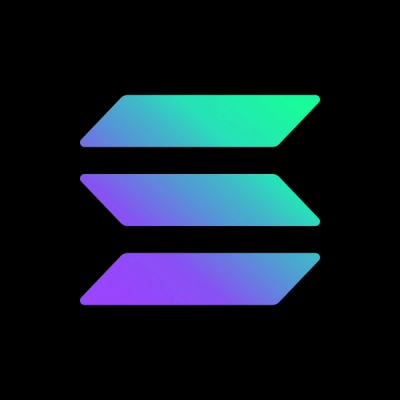
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
@frangiskos/git-secrets
Advanced tools
Save environment secrets or any other type of information encrypted in GitHub
A simple way to handle secrets by saving them in GitHub
npm install @frangiskos/github-secrets -D
import { sql, SqlConfig } from '@frangiskos/mssql';
const sqlConfig: SqlConfig = {
user: 'my_db_user',
password: 'my_super_secret_password',
database: 'my_database_name',
server: 'the_sql_server',
};
await sql.init(sqlConfig);
Lets say you have 2 files you want to encrypt:
For each file create the corresponding files with the encryption key and add a key / code phrase to use for encryption. Make sure you use a stro
run npx git-secrets save
to create the encrypted version of the files
The first parameter is the SQL query to execute. Use @P1, @P2 for parameter values. the rest parameters are the values for the parameters (The first one will replace @P1, the second will replace @P2 and so on)
import { sql } from '@frangiskos/mssql';
sql.query('SELECT * FROM USERS WHERE name like @P1 AND isActive = @P2', 'John%', true)
.then((data) => console.log(data))
.catch((error) => console.error(error));
try {
const data = await sql.query('SELECT * FROM USERS WHERE name like @P1 AND isActive = @P2', 'John%', true);
} catch (error) {
console.log(error);
}
SQL Functions are special methods that make it easier to work with sql in some cases.
INSERT RECORD
await sql.q(
`INSERT INTO people (name, birthdate, childrenCount, salary, isMarried)
VALUES (@P1, @P2, @P3, @P4, @P5)`,
'Johnny',
new Date('2000-01-01'),
2,
2345.67,
true
};
INSERT AND GET ID
const id = await sql.ii(`INSERT INTO people (name) VALUES (@P1)`, 'Not Johnny');
UPDATE USING ISO DATE STRING
const id = await sql.q(`UPDATE people SET birthdate=@P1 WHERE id=@P2`, '2000-01-01', 2);
SELECT RECORDS FROM TABLE
const people = await sql.q(
`SELECT * FROM people WHERE name like @P1`,
'%Johnny')
); // returns an array with all matching records
SELECT FIRST RECORD FROM TABLE
const Johnny = await sql.q1(
`SELECT * FROM people WHERE id = @P1`,
1)
); // returns the first matching record or null
SELECT THE VALUE OF THE FIRST KEY OF THE FIRST RECORD
const JohnnyName = await sql.qv(
`SELECT name FROM people WHERE id = @P1`,
1)
); // returns the value of the first key of the first matching record or null
const totalPeople = await sql.qv(
`SELECT count(*) FROM people`)
); // returns the number of records in table
INSERT OBJECT FUNCTION
await sql.functions.insertObject('people', {
name: 'Mike',
birthdate: '2000-02-03',
childrenCount: 0,
salary: 3000,
isMarried: false,
});
BULK INSERT FUNCTION
const data = [];
for (let i = 0; i < 10000; i++) {
data.push({
name: 'Name',
birthdate: '2000-01-01',
childrenCount: 0,
salary: 2000,
isMarried: false,
});
}
const res = await sql.functions.bulkInsert('people', data);
console.log(`inserted ${res.rowsAffected} records in ${res.executionTime / 1000} secs.`);
See ./src/tests.ts for more examples.
FAQs
Save environment secrets or any other type of information encrypted in GitHub
We found that @frangiskos/git-secrets demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.