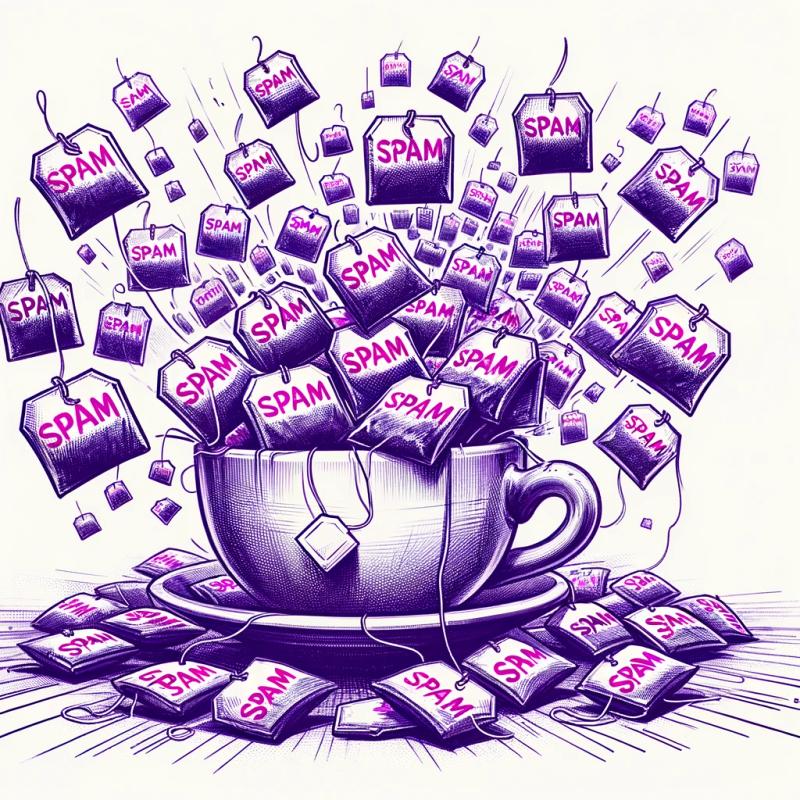
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@frangiskos/mssql
Advanced tools
Readme
A simple way to run SQL queries using Async/Await and Promises. This is not an ORM.
It uses node-mssql under the hood
npm install @frangiskos/mssql
import { sql, SqlConfig } from '@frangiskos/mssql';
const sqlConfig: SqlConfig = {
user: 'my_db_user',
password: 'my_super_secret_password',
database: 'my_database_name',
server: 'the_sql_server',
};
await sql.init(sqlConfig);
The first parameter is the SQL query to execute. Use @P1, @P2 for parameter values. the rest parameters are the values for the parameters (The first one will replace @P1, the second will replace @P2 and so on)
import { sql } from '@frangiskos/mssql';
sql.query('SELECT * FROM USERS WHERE name like @P1 AND isActive = @P2', 'John%', true)
.then((data) => console.log(data))
.catch((error) => console.error(error));
try {
const data = await sql.query('SELECT * FROM USERS WHERE name like @P1 AND isActive = @P2', 'John%', true);
} catch (error) {
console.log(error);
}
SQL Functions are special methods that make it easier to work with sql.
tmp_merge_${targetTable}
and insert the objects in bulk. Then it will merge the tmp_merge_${targetTable}
table with the target table.INSERT RECORD
await sql.q(
`INSERT INTO people (name, birthdate, childrenCount, salary, isMarried)
VALUES (@P1, @P2, @P3, @P4, @P5)`,
'Johnny',
new Date('2000-01-01'),
2,
2345.67,
true
};
INSERT AND GET ID
const id = await sql.ii(`INSERT INTO people (name) VALUES (@P1)`, 'Not Johnny');
UPDATE USING ISO DATE STRING
const id = await sql.q(`UPDATE people SET birthdate=@P1 WHERE id=@P2`, '2000-01-01', 2);
SELECT RECORDS FROM TABLE
const people = await sql.q(
`SELECT * FROM people WHERE name like @P1`,
'%Johnny')
); // returns an array with all matching records
SELECT FIRST RECORD FROM TABLE
const Johnny = await sql.q1(
`SELECT * FROM people WHERE id = @P1`,
1)
); // returns the first matching record or null
SELECT THE VALUE OF THE FIRST KEY OF THE FIRST RECORD
const JohnnyName = await sql.qv(
`SELECT name FROM people WHERE id = @P1`,
1)
); // returns the value of the first key of the first matching record or null
const totalPeople = await sql.qv(
`SELECT count(*) FROM people`)
); // returns the number of records in table
INSERT OBJECT FUNCTION
await sql.functions.insertObject('people', {
name: 'Mike',
birthdate: '2000-02-03',
childrenCount: 0,
salary: 3000,
isMarried: false,
});
BULK INSERT FUNCTION
await sql.functions.bulkInsert('people', [
{
name: 'Mike',
birthdate: '2000-02-03',
childrenCount: 0,
salary: 3000,
isMarried: false,
},
]);
MERGE TABLES FUNCTION
await sql.functions.mergeTables('srcTable', 'destTable', {
matchFields: ['InvoiceNumber'],
insertFields: ['InvoiceDate', 'amount'],
updateFields: ['amount'],
deleteNotMatching: true,
});
MERGE VALUES FUNCTION
await sql.functions.mergeValues(
[
{ InvoiceNumber: 1000, InvoiceDate: new Date(), amount: 5000 },
{ InvoiceNumber: 1001, InvoiceDate: new Date(), amount: 10000 },
],
'destTable',
{
matchFields: ['InvoiceNumber'],
insertFields: ['InvoiceDate', 'amount'],
updateFields: ['amount'],
deleteNotMatching: false,
}
);
See ./src/tests.ts for more examples.
FAQs
Connect to an MS-SQL server and run queries using a simple API
The npm package @frangiskos/mssql receives a total of 17 weekly downloads. As such, @frangiskos/mssql popularity was classified as not popular.
We found that @frangiskos/mssql demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.