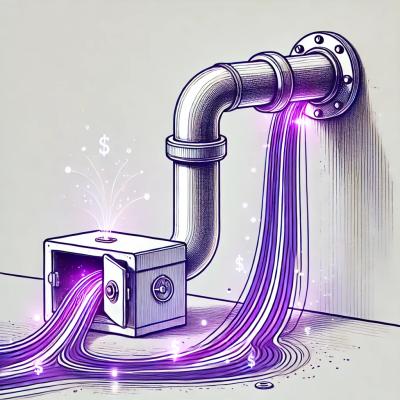
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@fugle/trade
Advanced tools
FugleTrade API SDK for Node.js
$ npm install --save @fugle/trade
// Using Node.js `require()`
const { FugleTrade, Order } = require('@fugle/trade');
// Using ES6 imports
import { FugleTrade, Order } from '@fugle/trade';
import { FugleTrade, Order } from '@fugle/trade';
const fugle = new FugleTrade({ configPath: '/path/to/config.ini' });
fugle.login().then(() => {
fugle.streamer.connect();
fugle.streamer.on('connect', () => {
console.log('streamer connected');
const order = new Order({
buySell: Order.Side.Buy,
price: 25.00,
stockNo: '2884',
quantity: 1,
apCode: Order.ApCode.Common,
priceFlag: Order.PriceFlag.Limit,
bsFlag: Order.BsFlag.ROD,
trade: Order.Trade.Cash,
});
// place order
fugle.placeOrder(order);
});
fugle.streamer.on('disconnect', () => {
console.log('streamer disconnected');
});
fugle.streamer.on('order', (data) => {
console.log('order confirmation:');
console.log(data);
});
fugle.streamer.on('trade', (data) => {
console.log('execution report:');
console.log(data);
});
});
Make sure you have applied to use Fugle trading API, downloaded the configuration file and certificate to your local machine.
First, create a FugleTrade
client with configPath
option:
const fugle = new FugleTrade({ configPath: '/path/to/config.ini' });
After creating the client, you need to log in to the remote server to obtain a valid api token:
await fugle.login();
When you log in for the first time, you need to set your account password and certificate password:
? Enter esun account password ********
? Enter cert password ********
Once logged in, you can start placing orders and viewing account information:
// Retrieve existing orders
const orders = await fugle.getOrders();
// Retrieve transaction details within three months
const transactions = await fugle.getTransactions({ duration: '3m' });
// Retrieve all inventories in your account
const inventories = await fugle.getInventories();
// Retrieve incoming settlements
const settlements = await fugle.getSettlements();
// Retrieve bank account balance
const balance = await fugle.getBalance();
// Retrieve trading quota and margin transaction information
const tradeStatus = await fugle.getTradeStatus();
// Retrieve market open status
const marketStatus = await fugle.getMarketStatus();
When you want to log in with another account, or need to reset your certificate password, please log out first:
await fugle.logout();
In order to place an order, you need to import Order
from @fugle/trade
.
import { Order } from '@fugle/trade';
Then, create an Order
instance that represents the order to place:
const order = new Order({
buySell: Order.Side.Buy,
price: 25.00,
stockNo: '2884',
quantity: 3,
apCode: Order.ApCode.Common,
priceFlag: Order.PriceFlag.Limit,
bsFlag: Order.BsFlag.ROD,
trade: Order.Trade.Cash,
});
// place the order
await fugle.placeOrder(order);
See /doc/fugle-trade.md
for details on available options.
If you want to replace or cancel an order, you need to get your all existing orders first.
const orders = await fugle.getOrders();
After that, you can choose one of the orders to replace or cancel it.
const [ order, ...others ] = orders;
// Replace the order to change price
await fugle.replacePrice(order, 24.5);
// Replace the order to change quantity
await fugle.replaceQuantity(order, 1);
// Cancel the order
await fugle.cancelOrder(order);
Streamer is an application that serves streaming data to clients of Fugle. If you want to receive order confirmation or execution report in real time, you need to connect the streamer.
Make sure you are logged in, then connect to the streamer:
fugle.streamer.connect();
The fugle.streamer
inherits from EventEmitter
that lets you register event listeners to listen for events:
fugle.streamer.on('connect', () => {
// streamer connected
});
fugle.streamer.on('disconnect', () => {
// streamer disconnected
});
fugle.streamer.on('order', (data) => {
// receive order confirmation
});
fugle.streamer.on('trade', (data) => {
// receive execution report
});
fugle.streamer.on('message', (data) => {
// receive message from streamer
});
fugle.streamer.on('error', (err) => {
// handle error
});
If you want to disconnect the streamer, just inovke:
fugle.streamer.disconnect();
See /doc/fugle-trade.md
for Node.js-like documentation of @fugle/trade
classes.
FAQs
FugleTrade API SDK for Node.js
We found that @fugle/trade demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.