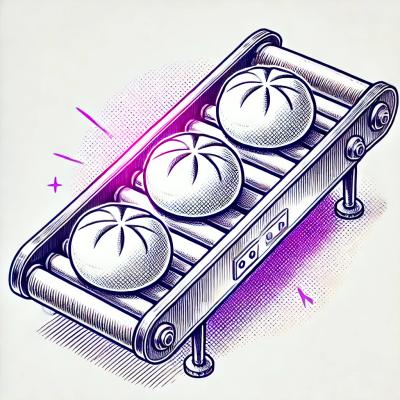
Security News
Bun 1.2 Released with 90% Node.js Compatibility and Built-in S3 Object Support
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
@fxjs/handbag
Advanced tools
fibjs >= 0.26.0
npm install @fxjs/handbag
register one compiler for .pug
file
const fxHandbag = require('@fxjs/handbag')
const fpug = require('fib-pug')
function registerPugAsHtml (vbox) {
const compilerOptions = {
filters: {
typescript: require('jstransformer-typescript').render,
stylus: require('jstransformer-stylus').render,
}
}
fxHandbag.vboxUtils.setCompilerForVbox(vbox, {
suffix: '.pug',
compiler: (buf, info) => fxHandbag.vboxUtils.wrapAsString(
fpug.renderFile(info.filename, compilerOptions)
)
})
}
const vm = require('vm')
vbox = new vm.SandBox(moduleHash)
registerPugAsHtml(vbox)
// just require one pug as rendered html string
const renderedHtml = vbox.require('./test.pug', __dirname)
you can also do like this:
const compilerOptions = {
filters: {
typescript: require('jstransformer-typescript').render,
stylus: require('jstransformer-stylus').render,
}
}
const vbox = new vm.SandBox(moduleHash)
fxHandbag.registers.pug.registerPugAsHtml(vbox, { compilerOptions })
const renderedHtml = vbox.require('./test.pug', __dirname)
vboxUtils
is some basic operation for one vbox generated by new vm.SandBox(...)
setCompilerForVbox(vbox: Class_SandBox, options: SetCompilerForVBoxOptions)
set one compiler
function for vbox
, the compiler
is used for vbox.setModuleCompiler
.
interface SetVboxOptions {
suffix: string|string[],
compiler: Function,
compile_to_iife_script?: boolean
}
register functions support options below:
{
// compilerOptions passed to render of register.
compilerOptions: {...},
// timeout that module keep required file id,
// - if it is 0, keep module always
// - if it is lower than 0, it would NIRVANA after remove it, that means module required would be updated after burnout_timeout.
burnout_timeout: {...}
}
plain.registerAsPlain(vbox, options)
['.txt']
register compiler to require file as its plain text
pug.registerPugAsRender(vbox, options)
['.pug', '.jade']
register compiler to require pug file as pug renderer
pug.registerPugAsHtml(vbox, options)
['.pug', '.jade']
register compiler to require pug file as rendered html
stylus.registerStylusAsCss(vbox, options)
['.styl', '.stylus']
register compiler to require stylus file as rendered html
typescript.registerTypescriptAsModule(vbox, options)
['.ts']
register compiler to require typescript file as one valid module
typescript.registerTypescriptAsPlainJavascript(vbox, options)
['.ts']
register compiler to require typescript file as plain javascript string.
rollup.registerAsModule(vbox, options)
['.ts', '.tsx']
register compiler to specified js-preprocessor file as module.
extra dependencies
rollup.registerAsPlainJavascript(vbox, options)
['.ts', '.tsx']
register compiler to specified js-preprocessor file as rolluped plain javascript string.
extra dependencies
vue.registerVueAsRollupedJavascript(vbox, options)
['.vue']
{}
, options passwd to rollup-plugin-vue'babel'
false
: no transpileregister compiler to require vue file as rolluped plain javascript string
NOTICE it's not recommend use async/await
in vue component, if you do so, the transpiled vue component's size would be large.
it would compile vue component js to 'es5' by default. If <script lang="ts">
set,
it always transpile component js with typescript
extra dependencies
rollup-plugin-buble (if use options.transpileLib="buble")
rollup-plugin-typescript (if use lang="ts")
tslib
typescript
react.registerReactAsRollupedJavascript(vbox, options)
started from 0.2.7
['.ts', '.jsx', '.tsx']
'babel'
false
: no transpileregister compiler to require react file as rolluped plain javascript string
NOTICE it's not recommend use async/await
in react component, if you do so, the transpiled vue component's size would be large.
extra dependencies
rollup-plugin-buble (if use options.transpileLib="buble")
rollup-plugin-typescript (if support suffix .ts
, .tsx
)
tslib
typescript
riot.registerRiotAsJs(vbox, options)
started from 0.2.3
['.tag', '.tsx']
true
register compiler to require .tag
file as compiled javascript string starting with riot.tag2(...)
.
image.registerImageAsBase64(vbox, options)
['.png', '.jpg', '.jpeg', '.gif', '.bmp']
register compiler to require image file as base64 string
graphql.registerGraphQLParser(vbox, options)
started from 0.2.4
['.gql', '.graphql']
register compiler to require graphql file as parsed graphql-js object.
extra dependencies
graphql.registerGraphQLAsQueryBuilder(vbox, options)
started from 0.2.4
['.gql', '.graphql']
register compiler to require graphql file as string builder, it's just simple text replacor.
// index.js
graphql.registerGraphQLAsQueryBuilder(vbox)
vbox.require('./foo.gql')({foo: 'bar'}) // result is { q1(foo: 'bar'){} }
// foo.gql
{ q1(foo: $foo){} }
registerOptions.compilerOptions
options passed to register's compiler/transpilor/renderer, view details in specified register
registerOptions.hooks
there's one emitter
option with EventEmitter
type in registerOptions
, all key
in registerOptions.hooks
would be registered as emitter's event_name with handler from registerOptions.hooks[key]
, e.g:
{
...
hooks: {
before_transpile: (payload) => {
// print required file's information
console.log(payload.info)
},
generated: (payload) => {
// change content from every file required by this Sandbox to 'always online'
payload.result = 'always online'
}
}
...
}
supported hooks
before_transpile
payload.raw
: original content's bufferpayload.info
: file informationgenerated
: payload: {result}
payload.result
: transpiled contentregisterOptions.rollup
There's register based on rollup, its registerOptions has those options:
options.rollup.bundleConfig
: default {}
, config passed to
const bundle = rollup.rollup({...})
options.rollup.writeConfig
: default {}
, config passed to
bundle.write({...})
bundle.generate({...})
options.rollup.onGenerateUmdName
: default (buf, info) => 's'
. generate name for rollup's umd
/iife
moderegisterOptions.burnout_timeout
In some cases, we want vbox to remove required module by ID after burnout_timeout
.
For example, you are developing one website's user profile page, which rendered from path/user-profile.pug
, you serve it with http.Server
and mq.Routing
, and require the pug file as require('path/user-profile.pug', __dirname)
with special vbox generated by registers in @fxjs/handbag
or by your own manual registering. You change the pug file, then you wish the next calling to require('path/user-profile.pug', __dirname)
would return the latest file resource. So, you need remove the required content every other times(such as 300ms), which is burnout_timeout
.
All reigsters's option in @fxjs/handbag
supports burnout_timeout
option.
nirvana
if burnout_timeout
set as Integer lower than 0, after one module(with moduleId mid
) removed from Sandbox(that would happend after burnout_timeout
), it would be require right away. e.g.
non-nirvana mode:
burnout_timeout = 200
sandbox.require(mid)
burnout_timeout
gone...sandbox.remove(mid)
sandbox.require(mid)
nirvana mode:
burnout_timeout = -200
sandbox.require(mid)
burnout_timeout
gone...sandbox.remove(mid)
and sandbox.require(mid)
20ms
In general, there's time-range about lower than 20ms
(it's tested) when you require one file as plain via registered box in @fxjs/handbag
, but it would over 20ms
in these cases:
If you develop app based on this feature(burnout_time
), notice the time-range
Copyright (c) 2018-present, Richard
FAQs
require resources directly 👝
The npm package @fxjs/handbag receives a total of 21 weekly downloads. As such, @fxjs/handbag popularity was classified as not popular.
We found that @fxjs/handbag demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.
Security News
Biden's executive order pushes for AI-driven cybersecurity, software supply chain transparency, and stronger protections for federal and open source systems.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.