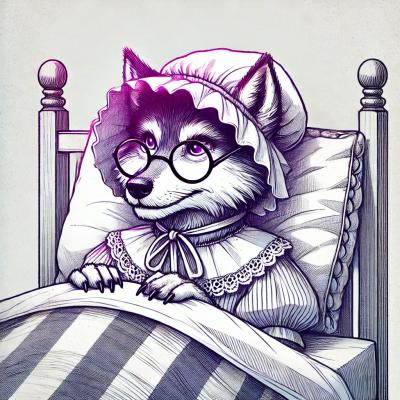
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@gaiads/telia-react-component-library
Advanced tools
A library of React-components to enable fast and consistent development of React-applications in Telia.
Gaia design system (@gaiads/telia-react-component-library) is now in maintenance mode, and DS team focuses fully on Global DS.
You are free to ask questions and help each other in #design-system Slack channel! Please let us know if you have any questions about this.
~ DS team
This is a react component library and living styleguide, showing the components which should be used in Telia Finland's web applications to achieve common look & feel, and therefore user experience.
You can install component library as any node package
npm install @gaiads/telia-react-component-library --save
In case the artifactory for some reason is a no go for you, you can install the library using Github.
npm install --save https://{USER}:{ACCESS_TOKEN}@github.com/TeliaSoneraFinland/telia-react-component-library/archive/{VERSION}.tar.gz
Read how to create an access token
Component library supports three types of module format
If you need to import UMD modules you need to target the UMD file explicity
import { button } from '@gaiads/telia-react-component-library/build/index.umd.js'
In case your project is typscript codebase currently UMD build doesn't support typescript. Exporting multiple type declaration files are not supported in npm package so far and because of this typescript will give error that corresponding type declaration file is missing. To fix this issue do the following
types
in same path as file which is importing component from component libraryindex.umd.d.ts
in the types
folderAdd following contents inside the file:
declare module '@gaiads/telia-react-component-library/build/index.umd.js';
You should add the styles into your bundle. Usually this is done by importing them in your index.js
like so:
import '@gaiads/telia-react-component-library/build/index.css';
The component library supports Typescript by exposing the d.ts file with component type declarations. Projects don't have to make any settings for taking this feature in use, but it works automatically for typescript projects.
The component library supports design tokens by exposing the css and scss variables files with. These files can be imported from build/tokens directory.
import '@gaiads/telia-react-component-library/build/tokens/css/_variables.css';
.my-class {
background-color: var(--gaia-color-black);
}
import '@gaiads/telia-react-component-library/build/tokens/scss/_variables.scss';
With growing numnber of UI elements it is challenging to manage z-index. Defining set of rules for z-index makes them more manageable. Z index token can be used as follows
import '@gaiads/telia-react-component-library/build/tokens/css/_variables.css';
.my-class {
z-index: var(--gaia-z-index-default);
}
import '@gaiads/telia-react-component-library/build/tokens/scss/_variables.scss';
.my-class {
z-index: $gaia-z-index-default;
}
It is also possible to import z-index scss function file and use this function with z-index name.
import '@gaiads/telia-react-component-library/build/styles/zindex.scss';
.my-class {
z-index: z('default');
}
git remote add upstream git@github.com:TeliaSoneraFinland/telia-react-component-library.git
git pull --rebase upstream master
git push --force-with-lease origin master
npm install
npm run storybook
npm run test
http://localhost:6007
It's very important that you read the coding conventions which have been documented in here. See also our code review checklist
To create new files, you can use the 'plop' script included in devDependencies.
npm run plop
You can also manually add the files. Make sure to follow coding conventions of the repo!
YourComponentName
in src/components
YourComponentName.test.js
and YourComponentName.js
YourComponentName.stories.js
file under src/styleguide
YourComponentName.module.scss
to same directory@gaiads/telia-react-component-library.d.ts
fileWhen developing, make sure to:
src/index.js
and add test to src/index.test.js
@gaiads/telia-react-component-library.d.ts
fileFor this, we have to utilize npm link
command. You have two options: either use the static build of the project or develop against running component library. When using the running component library you can test changes in command library's end and see the changes in our application immediately.
Remember that this is only for development and when you are deploying your own project then you should always use npm install
to get the latest version of component library
sudo npm link ../<your_project_name>/node_modules/react
npm run start
@gaiads/telia-react-component-library
as a dependencynpm link @gaiads/telia-react-component-library
npm install npm-link-shared
and npm-link-shared ./node_modules/@gaiads/telia-react-component-library/node_modules . react
from your project directory. npm-link-shared will enable your project to use react version from the telia-react-component.library package in order to avoid errors related to different React version.npm i
will break the linking.npm link
npm run build
@gaiads/telia-react-component-library
as a dependencynpm link @gaiads/telia-react-component-library
npm run build
it should be automatically updated in your project as wellnpm install npm-link-shared
and npm-link-shared ./node_modules/@gaiads/telia-react-component-library/node_modules . react
from your project directory. npm-link-shared will enable your project to use react version from the telia-react-component.library pacakge in order to avoid errors related to different React version.npm i
will break the linking.Component library uses Semantic versioning.
Run npm login
and when the command line asks, enter your deveo credentials and suiting email. After this you'll be logged in to artifactory and you are able to make actions such publishing into the artifactory.
Include .npmrc file into the root of your project. File should include:
registry=https://artifactory.verso.sonera.fi:443/api/npm/npm-registry-virtual/
always-auth = true
Then login to the artifactory by running npm login
. When the command line asks, enter your deveo credentials and suiting email. After this you'll be logged in to artifactory and you are able to install the dependencies.
Remember to include the credentials also to your CI!
npm run release-prep
to patch a versionSEMVER=minor npm run release-prep
SEMVER=major npm run release-prep
npm run visual-test
@gaiads/telia-react-component-library
repository with commit message Release preparations for <VERSION>
, for example Release preparations for v0.0.11
git push --set-upstream origin release/<VERSION>
git push --set-upstream origin release/v6.14.1
release/v<VERSION>
branch.WARNING
!!Before going further make sure that you pull request is approved by your collegue and merged into master!!
Once your release preparations are in the master go to the GitHub UI.
package.json
git fetch
and rebase git rebase origin/master
, with your local master
branchnpm publish
in order to publish the package into the artifactory@gaiads/telia-react-component-library
keyword to check if the latest version was indeed published.
npm run process-icons
in order to add icon into allowed icons list. Parser takes the name from the icon's file namenpm run build
or npm run storybook
You can use colors, baseline, breakpoints, spacing and scalableTypography sass helpers.
Import colors from the library and refer them with color(color_name).
@import '@gaiads/telia-react-component-library/build/styles/colors.scss';
.myview {
color: color(purple500);
background-color: color(gray100);
}
Import breakpoint from the library and refer them with breakpoint name such "md".
@import '@gaiads/telia-react-component-library/build/styles/breakpoints.scss';
.myview {
width: 100%;
@include from('md') {
width: 80%
}
}
Import baseline from the library and refer it as $baseline or $typographyBaseline.
@import '@gaiads/telia-react-component-library/build/styles/baseline.scss';
.myview {
height: 10 * $baseline;
font-size: 3 * $typographyBaseline;
}
Import fluidTypographyMixins from the library and define the font size scaling @include fluid-type(minSize, maxSize)
;
.
@import '@gaiads/telia-react-component-library/build/styles/fluidTypographyMixins.scss';
.myview {
@include fluid-type(10px, 20px);
}
FAQs
A library of React-components to enable fast and consistent development of React-applications in Telia.
The npm package @gaiads/telia-react-component-library receives a total of 60 weekly downloads. As such, @gaiads/telia-react-component-library popularity was classified as not popular.
We found that @gaiads/telia-react-component-library demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.