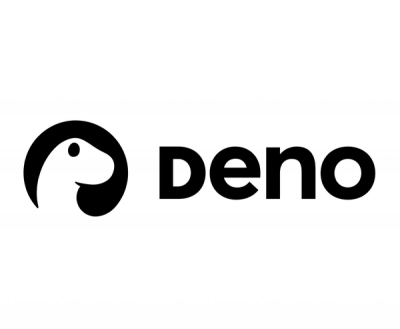
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@gloojs/core-ui
Advanced tools
core-ui
NavigationNavigation
is a shared, consistent navbar component for all new Gloo applications.
Mobile | Desktop |
---|---|
![]() | ![]() |
$ npm i @gloojs/core-ui --save
# or
$ yarn add @gloojs/core-ui
# Install peerDependencies
npx install-peerdeps @gloojs/core-ui --auth $NPM_TOKEN
The Navigation
component initiates REST API calls using the config passed via the client
prop. For these API calls to succeed, your application server needs to proxy those request to the backend services. It is recommended to use the glooApiProxyMiddleware for this purpose.
Due to design wishes, Navigation is always fixed at the top of the page. Because of this, Navigation exports it's height as a static property, Navigation.height
.
Navigation
There are cases when you may not need to control current location because your application is working only on organization level, in these cases you can pass in only your organizationId
and the organization/location dropdown changes to only display organizations. When you need to be able to switch between locations it's sufficient to pass in only the locationId
prop. We can derive the current organization from the location.
If no valid location/organization is passed to Navigation
, the dropdown will be hidden.
import { Navigation, createClient } from "@gloojs/core-ui";
import { useRouter } from "next/router";
const client = createClient({ baseURL: "/gloo-api" });
function App(props) {
const router = useRouter();
const isAdmin = router.query.page === "admin";
const changeRoute = (e, id) => {
e.preventDefault();
router.push(`${router.pathname}?id=${id}`);
};
const links = [
{
text: "People",
href: "#people",
onClick: () => {}
},
{
text: "Dashboards",
href: "#dashboards"
}
];
const config = {
onLocationChange: (e, { locId, orgId }) => changeRoute(e, locId),
onOrganizationChange: (e, { orgId, locId }) => changeRoute(e, orgId),
getLocationHref: locId => `/app?id=${locId}`,
getOrganizationHref: orgId => `/app?id=${orgId}`,
signOutLink: "/logout",
onSignOutClick: () => console.log("Logged out"),
applicationContext: {
name: "Home",
onClick: () => console.log("clicked home"),
href: "/",
logoSrc: "https://assets.gloo.us/design/some-cool-logo.png"
},
links,
appDomain: process.env.APP_DOMAIN
};
const organization = useMemo(() => ({ id: router.query.id }), [
router.query.id
]);
const orgProps = isAdmin
? { organizationId: organization.id }
: { locationId: organization.id };
return (
<div styles={{paddingTop: `${Navigation.height}px`}}>
<Navigation config={config} {...orgProps} />
</div>
);
}
export default App;
organizationId
string
| default:undefined
Used to control currently selected organization.
locationId
string
| default:undefined
Used to control currently selected location.
client
function(options:
RequestConfig) | Required
Used to configure axios
client instance.
config
object
| Required
More detailed description of all props within config is detailed under here.
disableOrgSwitching
boolean
| default:false
Switch off/enable organization switching dropdown.
onLocationChange
function(e: Event, {locId: string, orgId: string})
| default:() => {/* noop */}
Called when location is changed in the locations dropdown, can be used to run side-effects such as programmatic route changes or logging. Returns the click event and the new current location and organization.
onOrganizationChange
function(e: Event, {locId: string, orgId: string})
| default:() => {/* noop */}
Called when organization is changed in the organizations dropdown, can be used to run side-effects such as programmatic route changes or logging. Returns the click event and the new current location and organization. Location defaults to the first location of the current organization.
getLocationHref
function(locationId: string)
| Required
Used to construct a link for the location list items.
getOrganizationHref
function(organizationId: string)
| Required
Used to construct a link for the organization list items.
signOutLink
string
| Required
Link for sign out page.
onSignOutClick
function()
| default:() => {/* noop */}
Can be used to perform side effects when signing out.
applicationContext
object
| Required
Configure information about the current gloo application.
Prop | Type | Description |
---|---|---|
name | string, required | Name of the current application. I.e Home, Admin, etc. |
href | string, required | Link to the index page of the current application. |
onClick | function() | Perform side-effects when clicking link to application index. |
logoSrc | string, required | Link to logo used for application. |
links
array
| default:undefined
Links to be shown on the navigation bar.
Prop | Type | Description |
---|---|---|
text | string, required | Text on link |
href | string, required | Link href . |
onClick | function() | Perform side-effects when clicking link. |
appDomain
string
| Required
Example
- dev.gloo.us / gloo.us
Used to configure environment on links, make sure you're using production env for deployed applications.
environmentBaseUrl
string
Used to configure environment on links, make sure you're using production env for deployed applications.
allowedOrganizationTypes
array
ofstring
| default:undefined
Restrict the organization dropdown to only organizations of the specified types, e.g. NETWORK
.
accountMenuLinks
array
| default:undefined
Links to be shown in the Account Dropdown between the Administration and Sign Out links.
Prop | Type | Description |
---|---|---|
text | string, required | Text on link |
href | string, required | Link href . |
FAQs
Shared components for internal and external gloo projects
The npm package @gloojs/core-ui receives a total of 2 weekly downloads. As such, @gloojs/core-ui popularity was classified as not popular.
We found that @gloojs/core-ui demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 47 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.