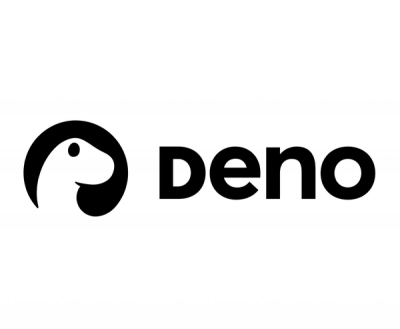
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@glue42/electron
Advanced tools
This package allows you to Glue42 enable your Electron application.
npm i @glue42/electron
import * as glue42Electron from '@glue42/electron';
...
const config = {
appDefinition: {
name: 'desktop-electron-app',
title: 'Desktop Electron App'
}
}
const glue = await glue42Electron.initialize(config);
const gdMainWindow = glue.registerStartupWindow(this.mainWindow, {allowChannels: true, channelId: "Red"});
When you do this and you run yor app, the package will:
By default the lib will inject Glue42 API into any window - if you want to change this and inject FDC3 you need to set inject option to "fdc3" when initializing the lib
const glue = await glue42Electron.initialize({ inject: "fdc3" });
const options = glue.startupOptions;
const context = options.context
const windowOptions = options.windowOptions;
You can also disable any injection by setting the option to none.
You can register your app as factory for certain window types, e.g. if you have a Chat Window in your app, you might want to allow other apps to create this window with a given context. To do so you can register an appFactory
glue.registerAppFactory(
{
name: "child-app-electron",
title: "Glue42 Electron Child Application"
}, function(appDefinition, context, glue42electron) {
// if you want some window options to override - you can use this
// this.title = "my special title"
// the first parameter is the app definition
// the second one is the context - when restoring, starting with context
// the third one is the service object which should be inject in the renderer, when preload: false
// should return the newly created BrowserWindow
const bw = new BrowserWindow();
return bw;
})
const bw = new BrowserWindow();
const glueWindow = glue.registerChildWindow(bw, {
name: "child-app-electron-2",
title: "Glue42 Electron Child Application"
}, {
mode: "tab",
left: 100,
top: 100,
width: 400,
height: 400
}
})
FAQs
Glue42 Electron library
The npm package @glue42/electron receives a total of 3 weekly downloads. As such, @glue42/electron popularity was classified as not popular.
We found that @glue42/electron demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.