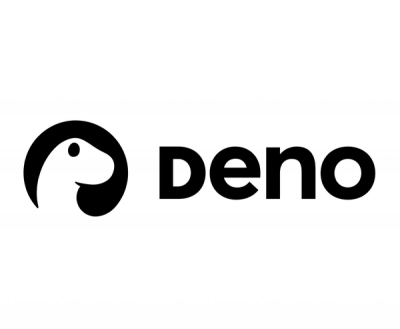
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@google-cloud/container
Advanced tools
Node.js idiomatic client for Kubernetes Engine cluster management.
Kubernetes Engine is used for building and managing container based applications, powered by the open source Kubernetes technology.
Read more about the client libraries for Cloud APIs, including the older Google APIs Client Libraries, in Client Libraries Explained.
Table of contents:
npm install @google-cloud/container
const container = require('@google-cloud/container');
// Create the Cluster Manager Client
const client = new container.v1.ClusterManagerClient();
async function quickstart() {
const zone = 'us-central1-a';
const projectId = await client.getProjectId();
const request = {
projectId: projectId,
zone: zone,
};
const [response] = await client.listClusters(request);
console.log('Clusters:');
console.log(response);
}
quickstart();
@google-cloud/container
provides a high level API for creating and managing
Google Kubernetes Engine clusters on Google Cloud.
To run commands against the clusters created, you will need to use the Kubernetes API (and the associated kubectl command-line interface).
Samples are in the samples/
directory. The samples' README.md
has instructions for running the samples.
Sample | Source Code | Try it |
---|---|---|
Quickstart | source code | ![]() |
The Kubernetes Engine Cluster Manager API Node.js Client API Reference documentation also contains samples.
This library follows Semantic Versioning.
This library is considered to be in alpha. This means it is still a work-in-progress and under active development. Any release is subject to backwards-incompatible changes at any time.
More Information: Google Cloud Platform Launch Stages
Contributions welcome! See the Contributing Guide.
Apache Version 2.0
See LICENSE
FAQs
Google Container Engine API client for Node.js
The npm package @google-cloud/container receives a total of 118,427 weekly downloads. As such, @google-cloud/container popularity was classified as popular.
We found that @google-cloud/container demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.