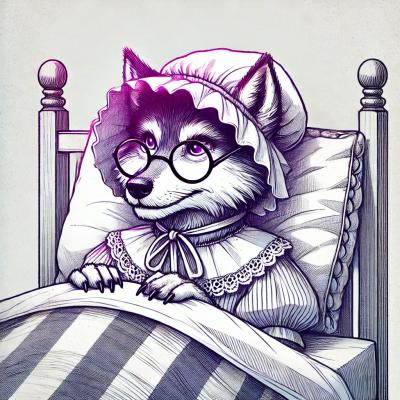
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@googlemaps/markerclusterer
Advanced tools
Creates and manages per-zoom-level clusters for large amounts of markers.
@googlemaps/markerclusterer is an npm package that provides functionality for clustering a large number of markers on a Google Map. This helps in improving the performance and user experience by grouping nearby markers into clusters, which can be expanded to show individual markers as the user zooms in.
Basic Marker Clustering
This feature allows you to create a basic marker clusterer on a Google Map. You initialize the map, create an array of markers, and then use the MarkerClusterer to cluster these markers.
const map = new google.maps.Map(document.getElementById('map'), {
center: { lat: -28.024, lng: 140.887 },
zoom: 4,
});
const markers = locations.map((location, i) => {
return new google.maps.Marker({
position: location,
});
});
new MarkerClusterer({
markers,
map
});
Custom Cluster Icons
This feature allows you to customize the icons used for clusters. You can specify a custom renderer function that returns a marker with a custom icon and label.
const map = new google.maps.Map(document.getElementById('map'), {
center: { lat: -28.024, lng: 140.887 },
zoom: 4,
});
const markers = locations.map((location, i) => {
return new google.maps.Marker({
position: location,
});
});
new MarkerClusterer({
markers,
map,
renderer: {
render: ({ count, position }) => {
return new google.maps.Marker({
position,
label: String(count),
icon: {
url: 'path/to/custom/icon.png',
scaledSize: new google.maps.Size(50, 50)
}
});
}
}
});
Cluster Click Event
This feature allows you to add an event listener for cluster clicks. When a cluster is clicked, you can access the markers within that cluster and perform custom actions.
const map = new google.maps.Map(document.getElementById('map'), {
center: { lat: -28.024, lng: 140.887 },
zoom: 4,
});
const markers = locations.map((location, i) => {
return new google.maps.Marker({
position: location,
});
});
const markerCluster = new MarkerClusterer({
markers,
map
});
markerCluster.addListener('clusterclick', (cluster) => {
const markers = cluster.getMarkers();
console.log('Cluster clicked:', markers);
});
leaflet.markercluster is a popular plugin for the Leaflet library that provides similar functionality for clustering markers on a map. It offers a variety of customization options and is widely used in the Leaflet community. Compared to @googlemaps/markerclusterer, it is designed specifically for Leaflet maps rather than Google Maps.
supercluster is a fast, geospatial point clustering library for browsers and Node. It is highly efficient and can handle large datasets. Unlike @googlemaps/markerclusterer, supercluster is not tied to any specific mapping library and can be used with various mapping solutions.
The library creates and manages per-zoom-level clusters for large amounts of markers.
See the history section and migration section for how this library relates to @google/markerclusterer and @googlemaps/markerclustererplus.
Available via npm as the package @googlemaps/markerclusterer.
npm i @googlemaps/markerclusterer
Alternativly you may add the umd package directly to the html document using the unpkg link.
<script src="https://unpkg.com/@googlemaps/markerclusterer/dist/index.min.js"></script>
When adding via unpkg, the MarkerClusterer
can be accessed at markerClusterer.MarkerClusterer
.
This library uses the official TypeScript typings for Google Maps Platform, @types/google.maps.
npm i -D @types/google.maps
The reference documentation is generated from the TypeScript definitions.
import { MarkerClusterer } from "@googlemaps/markerclusterer";
// use default algorithm and renderer
const markerCluster = new MarkerClusterer({ map, markers });
View the package in action:
Algorithm Comparisons - This example demonstrates the different algorithms. Please note that spacing and many other options can be changed for each algorithm.
Renderer Usage - This example demonstrates different renderers similar to the image below.
This library has a heritage in @google/markerclusterer and @googlemaps/markerclustererplus, originally made available on code.google.com and then transferred to GitHub at https://github.com/googlemaps/v3-utility-library. The following is an approximate timeline.
The API of @googlemaps/markerclusterer has changed in a number of ways from @googlemaps/markerclustererplus.
MarkerClusterer
class now accepts an algorithm
and renderer
parameter to allow for more flexibility. The interface looks like the following:{
algorithm?: Algorithm;
map?: google.maps.Map;
markers?: google.maps.Marker[];
renderer?: Renderer;
onClusterClick?: onClusterClickHandler;
}
MarkerClusterer
accepts a single options argument instead of positional parameters.GridAlgorithm
is still supported, but is not the default. The default is supercluster which uses k-d trees for improved performance.MarkerClusterer
class is still an instance of google.maps.OverlayView
, but uses google.maps.Marker
s instead of google.maps.Overlay
to render the clusters. This solves issues related to the usage of map panes and click handlers.FAQs
Creates and manages per-zoom-level clusters for large amounts of markers.
The npm package @googlemaps/markerclusterer receives a total of 0 weekly downloads. As such, @googlemaps/markerclusterer popularity was classified as not popular.
We found that @googlemaps/markerclusterer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.