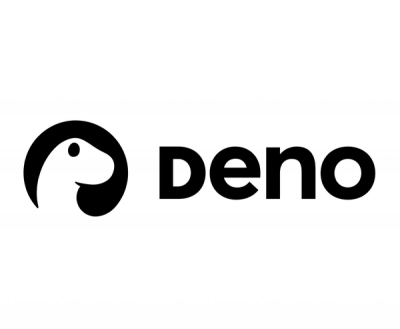
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@googlemaps/react-wrapper
Advanced tools
React component that wraps the loading of Google Maps JavaScript API.
@googlemaps/react-wrapper is an npm package that provides a simple way to integrate Google Maps into React applications. It acts as a wrapper around the Google Maps JavaScript API, making it easier to use within the React ecosystem.
Basic Map Rendering
This code demonstrates how to render a basic Google Map using the @googlemaps/react-wrapper package. The Wrapper component takes an API key and a render function to handle different loading states.
import React from 'react';
import { Wrapper, Status } from '@googlemaps/react-wrapper';
const render = (status) => {
if (status === Status.LOADING) return <p>Loading...</p>;
if (status === Status.FAILURE) return <p>Error loading map</p>;
return null;
};
const MyMapComponent = () => {
return (
<Wrapper apiKey={"YOUR_API_KEY"} render={render}>
<div id="map" style={{ height: '400px', width: '100%' }}></div>
</Wrapper>
);
};
export default MyMapComponent;
Adding Markers
This code demonstrates how to add a marker to a Google Map using the @googlemaps/react-wrapper package. The useEffect hook initializes the map and adds a marker to it.
import React, { useEffect, useRef } from 'react';
import { Wrapper, Status } from '@googlemaps/react-wrapper';
const render = (status) => {
if (status === Status.LOADING) return <p>Loading...</p>;
if (status === Status.FAILURE) return <p>Error loading map</p>;
return null;
};
const MyMapComponent = () => {
const mapRef = useRef(null);
useEffect(() => {
if (mapRef.current) {
const map = new window.google.maps.Map(mapRef.current, {
center: { lat: -34.397, lng: 150.644 },
zoom: 8,
});
new window.google.maps.Marker({
position: { lat: -34.397, lng: 150.644 },
map,
title: 'Hello World!',
});
}
}, []);
return (
<Wrapper apiKey={"YOUR_API_KEY"} render={render}>
<div id="map" ref={mapRef} style={{ height: '400px', width: '100%' }}></div>
</Wrapper>
);
};
export default MyMapComponent;
Handling Map Events
This code demonstrates how to handle map events, such as clicks, using the @googlemaps/react-wrapper package. The useEffect hook initializes the map and sets up an event listener for map clicks.
import React, { useEffect, useRef } from 'react';
import { Wrapper, Status } from '@googlemaps/react-wrapper';
const render = (status) => {
if (status === Status.LOADING) return <p>Loading...</p>;
if (status === Status.FAILURE) return <p>Error loading map</p>;
return null;
};
const MyMapComponent = () => {
const mapRef = useRef(null);
useEffect(() => {
if (mapRef.current) {
const map = new window.google.maps.Map(mapRef.current, {
center: { lat: -34.397, lng: 150.644 },
zoom: 8,
});
map.addListener('click', (e) => {
alert(`Clicked at: ${e.latLng}`);
});
}
}, []);
return (
<Wrapper apiKey={"YOUR_API_KEY"} render={render}>
<div id="map" ref={mapRef} style={{ height: '400px', width: '100%' }}></div>
</Wrapper>
);
};
export default MyMapComponent;
react-google-maps is a popular package for integrating Google Maps into React applications. It provides a set of React components that wrap the Google Maps JavaScript API, making it easier to work with maps in a React context. Compared to @googlemaps/react-wrapper, react-google-maps offers more built-in components and higher-level abstractions, but it may be less flexible for custom implementations.
google-maps-react is another package for integrating Google Maps into React applications. It provides a set of React components and utilities for working with the Google Maps JavaScript API. Compared to @googlemaps/react-wrapper, google-maps-react offers a more comprehensive set of features and components, but it may have a steeper learning curve for beginners.
react-map-gl is a package for integrating Mapbox maps into React applications. While it does not use the Google Maps API, it offers similar functionality for working with interactive maps. Compared to @googlemaps/react-wrapper, react-map-gl provides a different set of features and is focused on Mapbox rather than Google Maps, making it a good alternative for those who prefer Mapbox.
Wrap React components with this library to load the Google Maps JavaScript API.
import { Wrapper } from "@googlemaps/react-wrapper";
const MyApp = () => (
<Wrapper apiKey={"YOUR_API_KEY"}>
<MyMapComponent />
</Wrapper>
);
The preceding example will not render any elements unless the Google Maps JavaScript API is successfully loaded. To handle error cases and the time until load is complete, it is recommended to provide render props.
import { Wrapper, Status } from "@googlemaps/react-wrapper";
const render = (status) => {
switch (status) {
case Status.LOADING:
return <Spinner />;
case Status.FAILURE:
return <ErrorComponent />;
case Status.SUCCESS:
return <MyMapComponent />;
}
};
const MyApp = () => <Wrapper apiKey={"YOUR_API_KEY"} render={render} />;
When combining children and render props, the children will render on success and the render prop will be executed for other status values.
import { Wrapper, Status } from "@googlemaps/react-wrapper";
const render = (status: Status): ReactElement => {
if (status === Status.FAILURE) return <ErrorComponent />;
return <Spinner />;
};
const MyApp = () => (
<Wrapper apiKey={"YOUR_API_KEY"} render={render}>
<MyMapComponent />
</Wrapper>
);
This wrapper uses @googlemaps/js-api-loader to load the Google Maps JavaScript API. This library uses a singleton pattern and will not attempt to load the library more than once. All options accepted by @googlemaps/js-api-loader are also accepted as props to the wrapper component.
The following snippets demonstrates the usage of useRef
and useEffect
hooks with Google Maps.
function MyMapComponent({
center,
zoom,
}: {
center: google.maps.LatLngLiteral;
zoom: number;
}) {
const ref = useRef();
useEffect(() => {
new window.google.maps.Map(ref.current, {
center,
zoom,
});
});
return <div ref={ref} id="map" />;
}
See the examples folder for additional usage patterns.
Available via npm as the package @googlemaps/react-wrapper.
npm i @googlemaps/react-wrapper
or
yarn add @googlemaps/react-wrapper
For TypeScript support additionally install type definitions.
npm i -D @types/google.maps
or
yarn add -D @types/google.maps
The reference documentation can be found at this link.
This library is community supported. We're comfortable enough with the stability and features of the library that we want you to build real production applications on it.
If you find a bug, or have a feature suggestion, please log an issue. If you'd like to contribute, please read How to Contribute.
FAQs
React component that wraps the loading of Google Maps JavaScript API.
The npm package @googlemaps/react-wrapper receives a total of 150,246 weekly downloads. As such, @googlemaps/react-wrapper popularity was classified as popular.
We found that @googlemaps/react-wrapper demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.