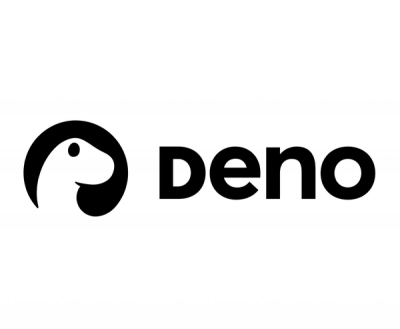
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@graphql-toolkit/graphql-tag-pluck
Advanced tools
Pluck graphql-tag template literals
@graphql-toolkit/graphql-tag-pluck
will take JavaScript code as an input and will pluck all template literals provided to graphql-tag
.
Input:
import gql from 'graphql-tag';
const fragment = gql`
fragment Foo on FooType {
id
}
`;
const doc = gql`
query foo {
foo {
...Foo
}
}
${fragment}
`;
Output:
fragment Foo on FooType {
id
}
query foo {
foo {
...Foo
}
}
Originally created because of https://graphql-code-generator.com/.
@graphql-toolkit/graphql-tag-pluck
is installable via NPM (or Yarn):
$ npm install @graphql-toolkit/graphql-tag-pluck
Once installed you can pluck GraphQL template literals using one of the following methods:
import gqlPluck, { gqlPluckFromFile, gqlPluckFromCodeString } from '@graphql-toolkit/graphql-tag-pluck';
// Returns promise
gqlPluck.fromFile(filePath, {
useSync: true, // Optional, will return string if so
});
// Returns string
gqlPluck.fromFile.sync(filePath);
// Returns string
gqlPluck.fromCodeString(codeString, {
fileExt: '.ts', // Optional, defaults to '.js'
});
Template literals leaded by magic comments will also be extracted :-)
/* GraphQL */ `
enum MessageTypes {
text
media
draftjs
}
`;
supported file extensions are: .js
, .jsx
, .ts
, .tsx
, .flow
, .flow.js
, .flow.jsx
, .graphqls
, .graphql
, .gqls
, .gql
.
I recommend you to look at the source code for a clearer understanding of the transformation options.
gqlMagicComment
The magic comment anchor to look for when parsing GraphQL strings. Defaults to graphql
, which may be translated into /* GraphQL */
in code.
globalGqlIdentifierName
Allows to use a global identifier instead of a module import.
// `graphql` is a global function
export const usersQuery = graphql`
{
users {
id
name
}
}
`;
modules
An array of packages that are responsible for exporting the GraphQL string parser function. The following modules are supported by default:
{
modules: [
{
// import gql from 'graphql-tag'
name: 'graphql-tag',
},
{
name: 'graphql-tag.macro',
},
{
// import { graphql } from 'gatsby'
name: 'gatsby',
identifier: 'graphql',
},
{
name: 'apollo-server-express',
identifier: 'gql',
},
{
name: 'apollo-server',
identifier: 'gql',
},
{
name: 'react-relay',
identifier: 'graphql',
},
{
name: 'apollo-boost',
identifier: 'gql',
},
{
name: 'apollo-server-koa',
identifier: 'gql',
},
{
name: 'apollo-server-hapi',
identifier: 'gql',
},
{
name: 'apollo-server-fastify',
identifier: 'gql',
},
{
name: ' apollo-server-lambda',
identifier: 'gql',
},
{
name: 'apollo-server-micro',
identifier: 'gql',
},
{
name: 'apollo-server-azure-functions',
identifier: 'gql',
},
{
name: 'apollo-server-cloud-functions',
identifier: 'gql',
},
{
name: 'apollo-server-cloudflare',
identifier: 'gql',
},
];
}
MIT
FAQs
Pluck graphql-tag template literals
The npm package @graphql-toolkit/graphql-tag-pluck receives a total of 11,966 weekly downloads. As such, @graphql-toolkit/graphql-tag-pluck popularity was classified as popular.
We found that @graphql-toolkit/graphql-tag-pluck demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.