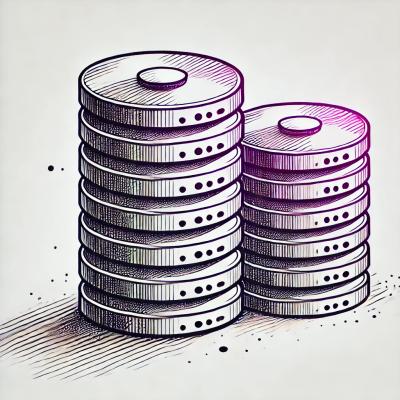
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
@graphql-typed-document-node/core
Advanced tools
The @graphql-typed-document-node/core package is designed to enhance the development experience with GraphQL by providing strongly typed document nodes. This package allows developers to use TypeScript to generate and utilize types directly from GraphQL queries, mutations, and subscriptions, ensuring type safety and reducing the likelihood of runtime errors.
Type-safe GraphQL queries
This feature allows developers to create GraphQL queries with embedded TypeScript types. The example shows how to define a query to fetch a user by ID, with the expected fields and types automatically inferred.
import { gql } from '@graphql-typed-document-node/core';
const GET_USER = gql`
query GetUser($id: ID!) {
user(id: $id) {
id
name
email
}
}
`;
// Usage in a TypeScript file ensures that `id` is required and the returned object is typed.
fetchUser(GET_USER, { id: '123' }).then(result => console.log(result.data.user.email));
Type-safe GraphQL mutations
This feature enables developers to write mutations with complete type safety. The example demonstrates updating a user's email, with TypeScript ensuring that all required variables are provided and correctly typed.
import { gql } from '@graphql-typed-document-node/core';
const UPDATE_USER_EMAIL = gql`
mutation UpdateUserEmail($id: ID!, $email: String!) {
updateUser(id: $id, email: $email) {
id
email
}
}
`;
// This mutation is fully typed, errors and variables are checked against the schema.
updateUserEmail(UPDATE_USER_EMAIL, { id: '123', email: 'newemail@example.com' }).then(result => console.log('Email updated:', result.data.updateUser.email));
Similar to @graphql-typed-document-node/core, this package also generates typed document nodes from GraphQL queries. However, it integrates with the GraphQL Code Generator tool, offering a broader range of code generation options and plugins for different frontend and backend frameworks.
While primarily a comprehensive state management library for JavaScript applications using GraphQL, Apollo Client also supports type generation through integration with tools like Apollo Tooling. It differs in that it provides a more extensive set of features for managing GraphQL data beyond just type safety.
FAQs
Unknown package
The npm package @graphql-typed-document-node/core receives a total of 3,897,964 weekly downloads. As such, @graphql-typed-document-node/core popularity was classified as popular.
We found that @graphql-typed-document-node/core demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.