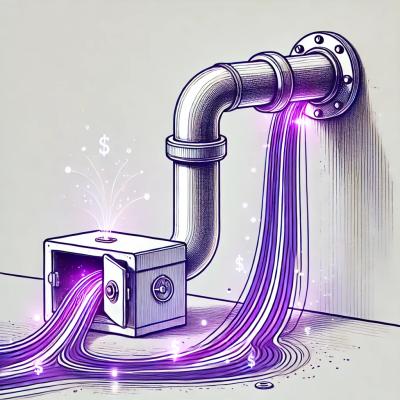
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@green-api/whatsapp-api-client
Advanced tools
Library to integrate with WhatsApp API. For details have look at https://green-api.com
This library helps you easily create a javascript application to connect the WhatsApp API using service green-api.com. You need to get ID_INSTANCE
and API_TOKEN_INSTANCE
in the control panel in order to use this library. It's free for developers.
The API corresponds with REST API from green-api since the library wraps own methods as https calls to the service. Therefore using these docs is highly encouraged.
Library supports both browser environment without package managers and Node/Webpack apps with package manager that can handle require
or import
module expressions.
For webpack and npm based apps:
npm i @green-api/whatsapp-api-client
For vanilla html-js website modify index.html:
<script src="https://unpkg.com/@green-api/whatsapp-api-client/lib/whatsapp-api-client.min.js"></script>
There are several ways to import the library in a project
Using common javascript
const whatsAppClient = require("@green-api/whatsapp-api-client");
Using ES6 javascript
import whatsAppClient from "@green-api/whatsapp-api-client";
Using typescript
import * as whatsAppClient from "@green-api/whatsapp-api-client";
Using browser javascript
<script src="https://unpkg.com/@green-api/whatsapp-api-client/lib/whatsapp-api-client.min.js"></script>
Sending WhatsApp message like any other call to the API requires account registered on green-api.com and authentication completed on mobile WhatsApp app. To register account you have to proceed to the control panel. After registering you wll get own unique pair of ID_INSTANCE
and API_TOKEN_INSTANCE
keys.
WhatsApp mobile app authentication may be achived by using control panel. You need to scan QR-code generated within the control panel.
Please do not use 'phoneNumber' parameter when calling methods. It is deprecated. Examples below are based on 'chatId' paramemeter
Use common javascript
const whatsAppClient = require("@green-api/whatsapp-api-client");
const restAPI = whatsAppClient.restAPI({
idInstance: "YOUR_ID_INSTANCE",
apiTokenInstance: "YOUR_API_TOKEN_INSTANCE",
});
restAPI.message.sendMessage("79999999999@c.us", null , "hello world").then((data) => {
console.log(data);
});
or use browser js script
<script src="https://unpkg.com/@green-api/whatsapp-api-client/lib/whatsapp-api-client.min.js"></script>
<script>
const restAPI = whatsAppClient.restAPI({
idInstance: "YOUR_ID_INSTANCE",
apiTokenInstance: "YOUR_API_TOKEN_INSTANCE",
});
restAPI.message
.sendMessage("79999999999@c.us", null, "hello world")
.then((data) => {
console.log(data);
})
.catch((reason) => {
console.error(reason);
});
</script>
Or use ES6 syntax. For node js app, you propably have to add in package.json
property "type": "module"
. Notice that all examples below are ES6 based.
import whatsAppClient from "@green-api/whatsapp-api-client";
(async () => {
const restAPI = whatsAppClient.restAPI({
idInstance: "YOUR_ID_INSTANCE",
apiTokenInstance: "YOUR_API_TOKEN_INSTANCE",
});
const response = await restAPI.message.sendMessage(
"79999999999@c.us",
null,
"hello world"
);
})();
ID_INSTANCE
and API_TOKEN_INSTANCE
keys (nodejs only!)You might want to store yours credentials separatedly from code. The library allow you to create a text file with preferred name and location with the format:
API_TOKEN_INSTANCE = "MY_API_TOKEN_INSTANCE"
ID_INSTANCE = "MY_ID_INSTANCE"
And then pass this file as described below:
const restAPI = whatsAppClient.restAPI({
credentialsPath: "examples\\credentials",
});
import whatsAppClient from "@green-api/whatsapp-api-client";
(async () => {
let restAPI = whatsAppClient.restAPI({
idInstance: "YOUR_ID_INSTANCE",
apiTokenInstance: "YOUR_API_TOKEN_INSTANCE",
});
try {
// Receive WhatsApp notifications.
console.log("Waiting incoming notifications...");
await restAPI.webhookService.startReceivingNotifications();
restAPI.webhookService.onReceivingMessageText((body) => {
console.log(body);
restAPI.webhookService.stopReceivingNotifications();
//console.log("Notifications is about to stop in 20 sec if no messages will be queued...")
});
restAPI.webhookService.onReceivingDeviceStatus((body) => {
console.log(body);
});
restAPI.webhookService.onReceivingAccountStatus((body) => {
console.log(body);
});
} catch (ex) {
console.error(ex.toString());
}
})();
import whatsAppClient from "@green-api/whatsapp-api-client";
(async () => {
const restAPI = whatsAppClient.restAPI({
idInstance: "YOUR_ID_INSTANCE",
apiTokenInstance: "YOUR_API_TOKEN_INSTANCE",
});
const response = await restAPI.file.sendFileByUrl(
"79999999999@c.us",
null,
"https://avatars.mds.yandex.net/get-pdb/477388/77f64197-87d2-42cf-9305-14f49c65f1da/s375",
"horse.png",
"horse"
);
})();
Webhooks are event-based callbacks invoked by green-api server as responses to client API calls. Webhooks support node js and express based apps only.
import whatsAppClient from "@green-api/whatsapp-api-client";
import express from "express";
import bodyParser from "body-parser";
(async () => {
try {
// Set http url, where webhooks are hosted.
// Url must have public domain address.
await restAPI.settings.setSettings({
webhookUrl: "MY_HTTP_SERVER:3000/webhooks",
});
const app = express();
app.use(bodyParser.json());
const webHookAPI = whatsAppClient.webhookAPI(app, "/webhooks");
// Subscribe to webhook happened when WhatsApp delivered a message
webHookAPI.onIncomingMessageText(
(data, idInstance, idMessage, sender, typeMessage, textMessage) => {
console.log(`outgoingMessageStatus data ${data.toString()}`);
}
);
// Run web server with public domain address
app.listen(3000, async () => {
console.log(`Started. App listening on port 3000!`);
const restAPI = whatsAppClient.restAPI({
idInstance: MY_ID_INSTANCE,
apiTokenInstance: MY_API_TOKEN_INSTANCE,
});
// Send test message that triggers webhook
const response = await restAPI.message.sendMessage(
"79999999999@c.us",
null,
"hello world"
);
});
} catch (error) {
console.error(error);
process.exit(1);
}
})();
There's some cool examples too.
Any help with development and bug fixing is appreciated. In order to deploy test-ready environment please make the steps:
git clone
npm install
rollup
for bundled builds.npm install express --save-dev
. Dont forget to delete it before making pull request"type": "module"
to the package.jsonCompile browser and node|webpack versions with single command:
npm run build
Publish to npm if you have access
npm publish
Licensed on MIT terms. For additional info have look at LICENSE
FAQs
Library to integrate with WhatsApp API. For details have look at https://green-api.com
The npm package @green-api/whatsapp-api-client receives a total of 293 weekly downloads. As such, @green-api/whatsapp-api-client popularity was classified as not popular.
We found that @green-api/whatsapp-api-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.