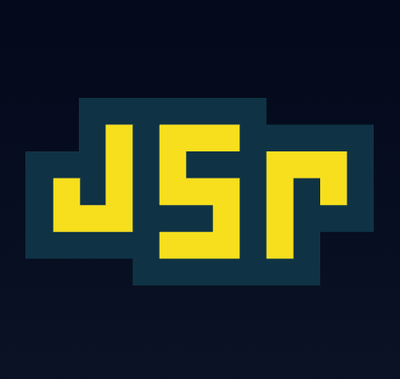
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@grpc/grpc-js
Advanced tools
The @grpc/grpc-js package is a pure JavaScript implementation of gRPC, which stands for gRPC Remote Procedure Calls. It allows you to define service methods and their input/output types using Protocol Buffers, and it handles the networking layer transparently. This package is used to build client and server applications that communicate over the gRPC protocol, which is particularly suited for microservices and other distributed systems where performance and scalability are important.
Defining a gRPC Service
This code demonstrates how to define a gRPC service using a .proto file. The service definition includes the RPC methods that can be called remotely.
const grpc = require('@grpc/grpc-js');
const protoLoader = require('@grpc/proto-loader');
const packageDefinition = protoLoader.loadSync('your_proto_file.proto', {});
const protoDescriptor = grpc.loadPackageDefinition(packageDefinition);
const yourService = protoDescriptor.your_package.YourService;
Creating a gRPC Server
This code sample shows how to create a gRPC server, add a service to it, and start listening for client requests on a specified port.
const grpc = require('@grpc/grpc-js');
const server = new grpc.Server();
server.addService(yourService.service, {
yourMethod: (call, callback) => {
// Implement your method logic here
callback(null, response);
}
});
server.bindAsync('0.0.0.0:50051', grpc.ServerCredentials.createInsecure(), () => {
server.start();
});
Creating a gRPC Client
This code snippet illustrates how to create a gRPC client that can call methods on a gRPC server. The client uses the service definition from a .proto file to make properly structured calls.
const grpc = require('@grpc/grpc-js');
const protoLoader = require('@grpc/proto-loader');
const packageDefinition = protoLoader.loadSync('your_proto_file.proto', {});
const protoDescriptor = grpc.loadPackageDefinition(packageDefinition);
const client = new protoDescriptor.your_package.YourService('localhost:50051', grpc.credentials.createInsecure());
client.yourMethod({ yourRequestField: 'yourValue' }, (error, response) => {
// Handle the response or error
});
The 'grpc' package is the original Node.js gRPC library, which relies on the C-based gRPC core library. It provides similar functionality to @grpc/grpc-js but requires native dependencies. The @grpc/grpc-js package was created as a pure JavaScript alternative, eliminating the need for native compilation and simplifying deployment, especially in serverless environments.
While 'protobufjs' is not a direct alternative to @grpc/grpc-js, it is a related package that provides tools for working with Protocol Buffers, which are an integral part of gRPC. It allows you to encode and decode Protocol Buffers messages but does not handle the gRPC networking layer.
Apache Thrift is another RPC framework that can be used as an alternative to gRPC. It supports multiple programming languages and has its own interface definition language (IDL). The 'thrift' package for Node.js allows you to build clients and servers using the Thrift protocol, which, like gRPC, is designed for efficient communication between services.
Note: This is an alpha-level release. Some APIs may not yet be present and there may be bugs. Please report any that you encounter
Node 9.x or greater is required.
npm install @grpc/grpc-js
This library does not directly handle .proto
files. To use .proto
files with this library we recommend using the @grpc/proto-loader
package.
FAQs
gRPC Library for Node - pure JS implementation
The npm package @grpc/grpc-js receives a total of 8,799,057 weekly downloads. As such, @grpc/grpc-js popularity was classified as popular.
We found that @grpc/grpc-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.