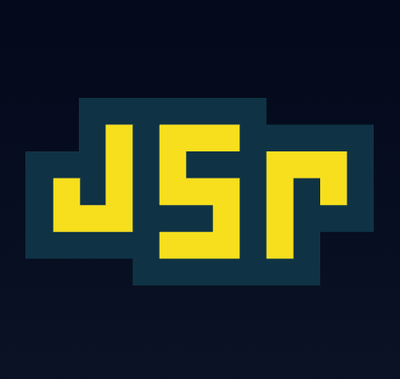
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@grpc/grpc-js
Advanced tools
The @grpc/grpc-js package is a pure JavaScript implementation of gRPC, which stands for gRPC Remote Procedure Calls. It allows you to define service methods and their input/output types using Protocol Buffers, and it handles the networking layer transparently. This package is used to build client and server applications that communicate over the gRPC protocol, which is particularly suited for microservices and other distributed systems where performance and scalability are important.
Defining a gRPC Service
This code demonstrates how to define a gRPC service using a .proto file. The service definition includes the RPC methods that can be called remotely.
const grpc = require('@grpc/grpc-js');
const protoLoader = require('@grpc/proto-loader');
const packageDefinition = protoLoader.loadSync('your_proto_file.proto', {});
const protoDescriptor = grpc.loadPackageDefinition(packageDefinition);
const yourService = protoDescriptor.your_package.YourService;
Creating a gRPC Server
This code sample shows how to create a gRPC server, add a service to it, and start listening for client requests on a specified port.
const grpc = require('@grpc/grpc-js');
const server = new grpc.Server();
server.addService(yourService.service, {
yourMethod: (call, callback) => {
// Implement your method logic here
callback(null, response);
}
});
server.bindAsync('0.0.0.0:50051', grpc.ServerCredentials.createInsecure(), () => {
server.start();
});
Creating a gRPC Client
This code snippet illustrates how to create a gRPC client that can call methods on a gRPC server. The client uses the service definition from a .proto file to make properly structured calls.
const grpc = require('@grpc/grpc-js');
const protoLoader = require('@grpc/proto-loader');
const packageDefinition = protoLoader.loadSync('your_proto_file.proto', {});
const protoDescriptor = grpc.loadPackageDefinition(packageDefinition);
const client = new protoDescriptor.your_package.YourService('localhost:50051', grpc.credentials.createInsecure());
client.yourMethod({ yourRequestField: 'yourValue' }, (error, response) => {
// Handle the response or error
});
The 'grpc' package is the original Node.js gRPC library, which relies on the C-based gRPC core library. It provides similar functionality to @grpc/grpc-js but requires native dependencies. The @grpc/grpc-js package was created as a pure JavaScript alternative, eliminating the need for native compilation and simplifying deployment, especially in serverless environments.
While 'protobufjs' is not a direct alternative to @grpc/grpc-js, it is a related package that provides tools for working with Protocol Buffers, which are an integral part of gRPC. It allows you to encode and decode Protocol Buffers messages but does not handle the gRPC networking layer.
Apache Thrift is another RPC framework that can be used as an alternative to gRPC. It supports multiple programming languages and has its own interface definition language (IDL). The 'thrift' package for Node.js allows you to build clients and servers using the Thrift protocol, which, like gRPC, is designed for efficient communication between services.
Node 12 is recommended. The exact set of compatible Node versions can be found in the engines
field of the package.json
file.
npm install @grpc/grpc-js
Documentation specifically for the @grpc/grpc-js
package is currently not available. However, documentation is available for the grpc
package, and the two packages contain mostly the same interface. There are a few notable differences, however, and these differences are noted in the "Migrating from grpc" section below.
If you need a feature from the grpc
package that is not provided by the @grpc/grpc-js
, please file a feature request with that information.
This library does not directly handle .proto
files. To use .proto
files with this library we recommend using the @grpc/proto-loader
package.
grpc
@grpc/grpc-js
is almost a drop-in replacement for grpc
, but you may need to make a few code changes to use it:
.proto
files using grpc.load
, that function is not available in this library. You should instead load your .proto
files using @grpc/proto-loader
and load the resulting package definition objects into @grpc/grpc-js
using grpc.loadPackageDefinition
.grpc-tools
, you should instead generate your files using the generate_package_definition
option in grpc-tools
, then load the object exported by the generated file into @grpc/grpc-js
using grpc.loadPackageDefinition
.Server#bind
to bind ports, you will need to use Server#bindAsync
instead.grpc
but not supported in @grpc/grpc-js
, you may need to adjust your code to handle the different behavior. Refer to the list of supported options below.grpc
and @grpc/grpc-js
.Many channel arguments supported in grpc
are not supported in @grpc/grpc-js
. The channel arguments supported by @grpc/grpc-js
are:
grpc.ssl_target_name_override
grpc.primary_user_agent
grpc.secondary_user_agent
grpc.default_authority
grpc.keepalive_time_ms
grpc.keepalive_timeout_ms
grpc.keepalive_permit_without_calls
grpc.service_config
grpc.max_concurrent_streams
grpc.initial_reconnect_backoff_ms
grpc.max_reconnect_backoff_ms
grpc.use_local_subchannel_pool
grpc.max_send_message_length
grpc.max_receive_message_length
grpc.enable_http_proxy
grpc.default_compression_algorithm
grpc.enable_channelz
grpc.dns_min_time_between_resolutions_ms
grpc.enable_retries
grpc.per_rpc_retry_buffer_size
grpc.retry_buffer_size
grpc.service_config_disable_resolution
grpc.client_idle_timeout_ms
grpc-node.max_session_memory
grpc-node.tls_enable_trace
channelOverride
channelFactoryOverride
The public API of this library follows semantic versioning, with some caveats:
Call
is only exposed due to limitations of TypeScript. It should not be considered part of the public API.grpc
library is likely an error and should not be considered part of the public API.grpc.experimental
namespace contains APIs that have not stabilized. Any API in that namespace may break in any minor version update.FAQs
gRPC Library for Node - pure JS implementation
The npm package @grpc/grpc-js receives a total of 8,799,057 weekly downloads. As such, @grpc/grpc-js popularity was classified as popular.
We found that @grpc/grpc-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.