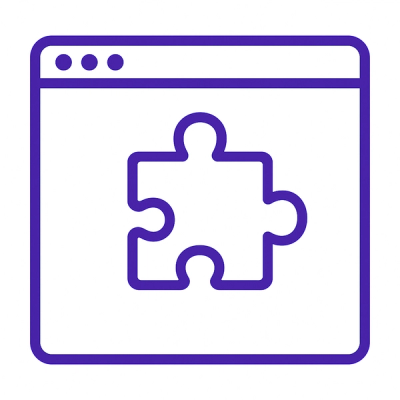
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
@grpc/proto-loader
Advanced tools
The @grpc/proto-loader package is used to load .proto files and generate JavaScript objects. It is primarily used in conjunction with gRPC, a high-performance, open-source universal RPC framework, to dynamically load protocol buffer definitions at runtime.
Loading .proto files
This feature allows you to load .proto files and create a package definition that can be used to make RPC calls.
const grpc = require('@grpc/grpc-js');
const protoLoader = require('@grpc/proto-loader');
const packageDefinition = protoLoader.loadSync('your_proto_file.proto', {});
const protoDescriptor = grpc.loadPackageDefinition(packageDefinition);
const yourService = protoDescriptor.your_package.YourService;
Customizing the loader options
This feature allows you to customize how the .proto files are loaded by specifying options such as whether to keep the original field casing or convert enums to strings.
const options = {
keepCase: true,
longs: String,
enums: String,
defaults: true,
oneofs: true
};
const packageDefinition = protoLoader.loadSync('your_proto_file.proto', options);
Asynchronous loading of .proto files
This feature allows you to load .proto files asynchronously, which can be useful if you want to handle the loading process without blocking the event loop.
const protoLoader = require('@grpc/proto-loader');
protoLoader.load('your_proto_file.proto', {}).then(packageDefinition => {
const protoDescriptor = grpc.loadPackageDefinition(packageDefinition);
// Use protoDescriptor to create gRPC service client
}).catch(err => {
console.error('Error loading .proto file', err);
});
Protobufjs is a pure JavaScript implementation of Protocol Buffers. It provides similar functionality to @grpc/proto-loader but with additional features like creating .proto files programmatically and a more complex API for handling protocol buffers.
grpc-tools is a package that includes protocol buffer compiler (protoc) and a plugin for generating gRPC service code. It is used for generating static service code from .proto files, as opposed to the dynamic loading approach of @grpc/proto-loader.
grpc-web provides a JavaScript library that lets browser clients access a gRPC service. It is similar to @grpc/proto-loader in that it deals with gRPC and protocol buffers, but it is specifically designed for web clients.
A utility package for loading .proto
files for use with gRPC, using the latest Protobuf.js package.
Please refer to protobuf.js' documentation
to understands its features and limitations.
npm install @grpc/proto-loader
const protoLoader = require('@grpc/proto-loader');
const grpcLibrary = require('grpc');
// OR
const grpcLibrary = require('@grpc/grpc-js');
protoLoader.load(protoFileName, options).then(packageDefinition => {
const packageObject = grpcLibrary.loadPackageDefinition(packageDefinition);
});
// OR
const packageDefinition = protoLoader.loadSync(protoFileName, options);
const packageObject = grpcLibrary.loadPackageDefinition(packageDefinition);
The options parameter is an object that can have the following optional properties:
Field name | Valid values | Description |
---|---|---|
keepCase | true or false | Preserve field names. The default is to change them to camel case. |
longs | String or Number | The type to use to represent long values. Defaults to a Long object type. |
enums | String | The type to use to represent enum values. Defaults to the numeric value. |
bytes | Array or String | The type to use to represent bytes values. Defaults to Buffer . |
defaults | true or false | Set default values on output objects. Defaults to false . |
arrays | true or false | Set empty arrays for missing array values even if defaults is false Defaults to false . |
objects | true or false | Set empty objects for missing object values even if defaults is false Defaults to false . |
oneofs | true or false | Set virtual oneof properties to the present field's name. Defaults to false . |
json | true or false | Represent Infinity and NaN as strings in float fields, and automatically decode google.protobuf.Any values. Defaults to false |
includeDirs | An array of strings | A list of search paths for imported .proto files. |
The following options object closely approximates the existing behavior of grpc.load
:
const options = {
keepCase: true,
longs: String,
enums: String,
defaults: true,
oneofs: true
}
The proto-loader-gen-types
script distributed with this package can be used to generate TypeScript type information for the objects loaded at runtime. More information about how to use it can be found in the @grpc/proto-loader TypeScript Type Generator CLI Tool proposal document. The arguments mostly match the load
function's options; the full usage information is as follows:
proto-loader-gen-types.js [options] filenames...
Options:
--help Show help [boolean]
--version Show version number [boolean]
--keepCase Preserve the case of field names
[boolean] [default: false]
--longs The type that should be used to output 64 bit
integer values. Can be String, Number
[string] [default: "Long"]
--enums The type that should be used to output enum fields.
Can be String [string] [default: "number"]
--bytes The type that should be used to output bytes
fields. Can be String, Array
[string] [default: "Buffer"]
--defaults Output default values for omitted fields
[boolean] [default: false]
--arrays Output default values for omitted repeated fields
even if --defaults is not set
[boolean] [default: false]
--objects Output default values for omitted message fields
even if --defaults is not set
[boolean] [default: false]
--oneofs Output virtual oneof fields set to the present
field's name [boolean] [default: false]
--json Represent Infinity and NaN as strings in float
fields. Also decode google.protobuf.Any
automatically [boolean] [default: false]
--includeComments Generate doc comments from comments in the original
files [boolean] [default: false]
-I, --includeDirs Directories to search for included files [array]
-O, --outDir Directory in which to output files
[string] [required]
--grpcLib The gRPC implementation library that these types
will be used with. If not provided, some types will
not be generated [string]
--inputTemplate Template for mapping input or "permissive" type
names [string] [default: "%s"]
--outputTemplate Template for mapping output or "restricted" type
names [string] [default: "%s__Output"]
--inputBranded Output property for branded type for "permissive"
types with fullName of the Message as its value
[boolean] [default: false]
--outputBranded Output property for branded type for "restricted"
types with fullName of the Message as its value
[boolean] [default: false]
--targetFileExtension File extension for generated files.
[string] [default: ".ts"]
--importFileExtension File extension for import specifiers in generated
code. [string] [default: ""]
Generate the types:
$(npm bin)/proto-loader-gen-types --longs=String --enums=String --defaults --oneofs --grpcLib=@grpc/grpc-js --outDir=proto/ proto/*.proto
Consume the types:
import * as grpc from '@grpc/grpc-js';
import * as protoLoader from '@grpc/proto-loader';
import type { ProtoGrpcType } from './proto/example.ts';
import type { ExampleHandlers } from './proto/example_package/Example.ts';
const exampleServer: ExampleHandlers = {
// server handlers implementation...
};
const packageDefinition = protoLoader.loadSync('./proto/example.proto');
const proto = (grpc.loadPackageDefinition(
packageDefinition
) as unknown) as ProtoGrpcType;
const server = new grpc.Server();
server.addService(proto.example_package.Example.service, exampleServer);
FAQs
gRPC utility library for loading .proto files
The npm package @grpc/proto-loader receives a total of 11,715,638 weekly downloads. As such, @grpc/proto-loader popularity was classified as popular.
We found that @grpc/proto-loader demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.