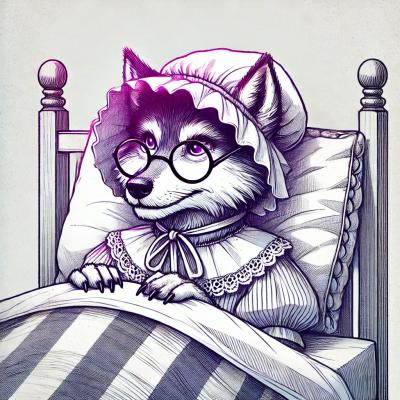
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@hapi/podium
Advanced tools
@hapi/podium is an event emitter with support for multiple event channels, filters, and event arguments. It is designed to be a more powerful and flexible alternative to the built-in Node.js EventEmitter.
Basic Event Emission
This feature allows you to emit and listen to events. The example demonstrates creating a Podium instance, registering an event listener, and emitting an event.
const Podium = require('@hapi/podium');
const podium = new Podium('event');
podium.on('event', (data) => {
console.log(data);
});
podium.emit('event', { message: 'Hello, world!' });
Multiple Event Channels
This feature allows you to manage multiple event channels. The example shows how to create a Podium instance with multiple events and handle them separately.
const Podium = require('@hapi/podium');
const podium = new Podium(['event1', 'event2']);
podium.on('event1', (data) => {
console.log('Event 1:', data);
});
podium.on('event2', (data) => {
console.log('Event 2:', data);
});
podium.emit('event1', { message: 'Hello from event 1!' });
podium.emit('event2', { message: 'Hello from event 2!' });
Event Filters
This feature allows you to filter events based on custom criteria. The example demonstrates setting up a filter that only processes events of a specific type.
const Podium = require('@hapi/podium');
const podium = new Podium('event');
podium.on('event', { filter: (data) => data.type === 'type1' }, (data) => {
console.log('Filtered event:', data);
});
podium.emit('event', { type: 'type1', message: 'This will be logged' });
podium.emit('event', { type: 'type2', message: 'This will not be logged' });
Event Arguments
This feature allows you to pass multiple arguments to event listeners. The example shows how to emit an event with multiple arguments and handle them in the listener.
const Podium = require('@hapi/podium');
const podium = new Podium('event');
podium.on('event', (arg1, arg2) => {
console.log('Arguments:', arg1, arg2);
});
podium.emit('event', 'arg1', 'arg2');
The 'events' module is the built-in Node.js EventEmitter. It provides basic event emission and handling capabilities. Compared to @hapi/podium, it lacks advanced features like multiple event channels, filters, and argument handling.
EventEmitter3 is a high-performance event emitter for Node.js and the browser. It offers a similar API to the built-in EventEmitter but with better performance. However, it does not provide the advanced features of @hapi/podium, such as multiple event channels and filters.
Mitt is a tiny (~200 bytes) functional event emitter. It is designed to be simple and fast, making it suitable for small projects or performance-critical applications. Unlike @hapi/podium, it does not support advanced features like event filters and multiple channels.
podium is part of the hapi ecosystem and was designed to work seamlessly with the hapi web framework and its other components (but works great on its own or with other frameworks). If you are using a different web framework and find this module useful, check out hapi – they work even better together.
FAQs
Node compatible event emitter with extra features
The npm package @hapi/podium receives a total of 0 weekly downloads. As such, @hapi/podium popularity was classified as not popular.
We found that @hapi/podium demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.