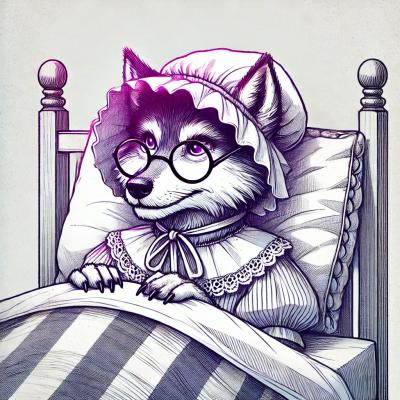
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@hapi/shot
Advanced tools
@hapi/shot is a Node.js library used for testing HTTP servers. It allows you to inject requests into a server and inspect the responses, making it useful for unit testing server-side code without the need to actually start the server.
Injecting Requests
This feature allows you to inject HTTP requests into a server and inspect the responses. The example demonstrates how to create a simple HTTP server and use Shot to inject a GET request, then log the status code and payload of the response.
const Shot = require('@hapi/shot');
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, world!');
});
Shot.inject(server, { method: 'get', url: '/' }, (res) => {
console.log(res.statusCode); // 200
console.log(res.payload); // 'Hello, world!'
});
Testing with Assertions
This feature allows you to perform assertions on the responses to ensure they meet expected criteria. The example shows how to create a server that responds with JSON and use Shot to inject a GET request, then assert the status code, content type, and payload.
const Shot = require('@hapi/shot');
const http = require('http');
const assert = require('assert');
const server = http.createServer((req, res) => {
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ message: 'Hello, world!' }));
});
Shot.inject(server, { method: 'get', url: '/' }, (res) => {
assert.strictEqual(res.statusCode, 200);
assert.strictEqual(res.headers['content-type'], 'application/json');
assert.deepStrictEqual(JSON.parse(res.payload), { message: 'Hello, world!' });
console.log('All assertions passed');
});
Supertest is a popular library for testing Node.js HTTP servers. It provides a high-level abstraction for testing HTTP, making it easy to write tests for your server. Compared to @hapi/shot, Supertest offers a more expressive API and integrates well with testing frameworks like Mocha and Jest.
Nock is a library for HTTP mocking and expectations. It allows you to intercept HTTP requests and provide predefined responses, making it useful for testing HTTP clients. While @hapi/shot is focused on injecting requests into servers, Nock is more about mocking external HTTP requests.
Axios-mock-adapter is a library for mocking axios requests. It allows you to define how axios should behave when making HTTP requests, which is useful for testing client-side code. Unlike @hapi/shot, which is server-focused, axios-mock-adapter is specifically designed for mocking HTTP requests made with axios.
shot is part of the hapi ecosystem and was designed to work seamlessly with the hapi web framework and its other components (but works great on its own or with other frameworks). If you are using a different web framework and find this module useful, check out hapi – they work even better together.
FAQs
Injects a fake HTTP request/response into a node HTTP server
The npm package @hapi/shot receives a total of 379,624 weekly downloads. As such, @hapi/shot popularity was classified as popular.
We found that @hapi/shot demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.