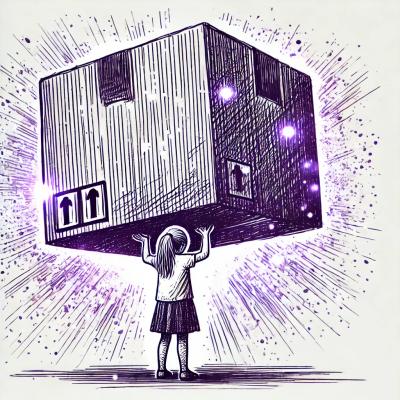
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
@hebcal/core
Advanced tools
Hebcal, a perpetual Jewish Calendar (ES6)
$ npm install @hebcal/core
import {hebcal, HDate, cities, Location, Event} from '@hebcal/core';
cities.init();
const options = {
year: 1981,
isHebrewYear: false,
candlelighting: true,
location: Location.newFromCity(cities.getCity('San Francisco')),
sedrot: true,
omer: true,
};
const events = hebcal.hebrewCalendar(options);
for (const ev of events) {
const hd = e.getDate();
const date = hd.greg();
console.log(date.toLocaleDateString(), e.render(), hd.toString());
}
Class representing a Hebrew date
Represents an Event with a title, date, and flags
Represents a day 1-49 of counting the Omer from Pesach to Shavuot
For a Daf Yomi, the name is already translated attrs.dafyomi.name contains the untranslated string
Havdalah after Shabbat or holiday
Candle lighting before Shabbat or holiday
Class representing Location
Represents Parashah HaShavua for an entire Hebrew year
Interface to lookup cities
boolean
Returns true if Hebrew year is a leap year
number
Number of months in Hebrew year
number
Number of days in Hebrew month in a given year
string
Returns an (untranslated) string name of Hebrew month in year
number
Returns the Hebrew month number
number
Days from sunday prior to start of Hebrew calendar to mean conjunction of Tishrei in Hebrew YEAR
number
Number of days in the hebrew YEAR
boolean
true if Cheshvan is long in Hebrew YEAR
boolean
true if Kislev is short in Hebrew YEAR
number
Converts Hebrew month string name to numeric
number
Note: Applying this function to d+6 gives us the DAYNAME on or after an absolute day d. Similarly, applying it to d+3 gives the DAYNAME nearest to absolute date d, applying it to d-1 gives the DAYNAME previous to absolute date d, and applying it to d+7 gives the DAYNAME following absolute date d.
Array.<number>
Returns an array from start to end
boolean
Returns true if the Gregorian year is a leap year
number
Number of days in the Gregorian month for given year
number
Returns number of days since January 1 of that year
number
Converts Gregorian date to Julian Day Count
Date
Converts from Julian Day Count to Gregorian date. See the footnote on page 384 of ``Calendrical Calculations, Part II: Three Historical Calendars'' by E. M. Reingold, N. Dershowitz, and S. M. Clamen, Software--Practice and Experience, Volume 23, Number 4 (April, 1993), pages 383-404 for an explanation.
HDate
number
Converts Hebrew date to absolute Julian days. The absolute date is the number of days elapsed since the (imaginary) Gregorian date Sunday, December 31, 1 BC.
SimpleHebrewDate
Converts Julian days to Hebrew date to absolute Julian days
Molad
Calculates the molad for a Hebrew month
HDate
Calculates a birthday or anniversary (non-yahrzeit). Year must be after original date of anniversary. Returns undefined when requested year preceeds or is same as original year.
HDate
Calculates yahrzeit. Year must be after original date of death. Returns undefined when requested year preceeds or is same as original year.
DafYomiResult
Returns the Daf Yomi for given date
string
Formats (with translation) the dafyomi result as a string like "Pesachim 34"
number
parsha doubler/undoubler
Object
Returns an object describing the parsha on the first Saturday on or after absdate
string
Map.<string, Array.<Event>>
Returns a Map for the year indexed by HDate.toString()
Array.<Event>
Returns an array of Events on this date (or undefined if no events)
string
Array.<Object>
Array.<Object>
Event
number
Array.<number>
Parse options object to determine start & end days
Array.<number>
number
Mask to filter Holiday array
Array.<Event>
Generates a list of holidays
Object
A simple Hebrew date
Object
Represents an Molad
Object
A City result
Object
A Daf Yomi result
Object
Options to configure which events are returned
Class representing a Hebrew date
Kind: global class
number
boolean
number
number
number
number
number
HDate
HDate
HDate
HDate
Date
number
string
string
HDate
HDate
HDate
HDate
HDate
HDate
HDate
boolean
Create a Hebrew date.
Param | Type | Description |
---|---|---|
[day] | number | Date | HDate | Day of month (1-30) |
[month] | number | Hebrew month of year (1=NISAN, 7=TISHREI) |
[year] | number | Hebrew year |
number
Gets the Hebrew year of this Hebrew date
Kind: instance method of HDate
boolean
Tests if this date occurs during a leap year
Kind: instance method of HDate
number
Gets the Hebrew month (1=NISAN, 7=TISHREI) of this Hebrew date
Kind: instance method of HDate
number
Kind: instance method of HDate
number
Number of days in the month of this Hebrew date
Kind: instance method of HDate
number
Gets the day within the month (1-30)
Kind: instance method of HDate
number
Gets the day of the week, using local time.
Kind: instance method of HDate
HDate
Kind: instance method of HDate
Param | Type |
---|---|
year | number |
HDate
Kind: instance method of HDate
Param | Type |
---|---|
month | number |
HDate
Kind: instance method of HDate
Param | Type |
---|---|
month | number |
HDate
Kind: instance method of HDate
Param | Type |
---|---|
date | number |
Date
Converts to Gregorian date
Kind: instance method of HDate
number
Returns Julian absolute days
Kind: instance method of HDate
string
Returns untranslated Hebrew month name
Kind: instance method of HDate
string
Returns translated/transliterated Hebrew date
Kind: instance method of HDate
HDate
Kind: instance method of HDate
Param | Type | Description |
---|---|---|
day | number | day of week |
HDate
Kind: instance method of HDate
Param | Type | Description |
---|---|---|
day | number | day of week |
HDate
Kind: instance method of HDate
Param | Type | Description |
---|---|---|
day | number | day of week |
HDate
Kind: instance method of HDate
Param | Type | Description |
---|---|---|
day | number | day of week |
HDate
Kind: instance method of HDate
Param | Type | Description |
---|---|---|
day | number | day of week |
HDate
Kind: instance method of HDate
HDate
Kind: instance method of HDate
boolean
Kind: instance method of HDate
Param | Type | Description |
---|---|---|
other | HDate | Hebrew date to compare |
Represents an Event with a title, date, and flags
Kind: global class
number
Object
boolean
boolean
string
string
HDate
Constructs Event
Param | Type | Default | Description |
---|---|---|---|
date | HDate | Hebrew date event occurs | |
desc | string | Description (not translated) | |
[mask] | number | 0 | optional holiday flags |
[attrs] | Object | {} |
number
Kind: instance method of Event
Object
Kind: instance method of Event
boolean
Is this event observed in Israel?
Kind: instance method of Event
boolean
Is this event observed in the Diaspora?
Kind: instance method of Event
string
Returns (translated) description of this event
Kind: instance method of Event
string
Returns untranslated description of this event
Kind: instance method of Event
HDate
Returns Hebrew date of this event
Kind: instance method of Event
Represents a day 1-49 of counting the Omer from Pesach to Shavuot
Kind: global class
Param | Type |
---|---|
date | HDate |
omerDay | number |
string
Kind: instance method of OmerEvent
Todo
For a Daf Yomi, the name is already translated attrs.dafyomi.name contains the untranslated string
Kind: global class
Param | Type |
---|---|
date | HDate |
desc | string |
attrs | Object |
string
Kind: instance method of DafYomiEvent
Havdalah after Shabbat or holiday
Kind: global class
Param | Type |
---|---|
date | HDate |
mask | number |
attrs | Object |
[havdalahMins] | number |
string
Kind: instance method of HavdalahEvent
Candle lighting before Shabbat or holiday
Kind: global class
Param | Type |
---|---|
date | HDate |
mask | number |
attrs | Object |
string
Kind: instance method of CandleLightingEvent
Class representing Location
Kind: global class
suncalc.GetTimesResult
Date
Date
number
number
Date
number
number
Date
Date
Date
Date
Date
Date
Date
Date
Date
Date
Date
Date
Date
Date
Initialize a Location instance
Param | Type | Description |
---|---|---|
latitude | number | Latitude as a decimal, valid range -90 thru +90 (e.g. 41.85003) |
longitude | number | Longitude as a decimal, valid range -180 thru +180 (e.g. -87.65005) |
il | boolean | in Israel (true) or Diaspora (false) |
tzid | string | Olson timezone ID, e.g. "America/Chicago" |
cityName | string | optional descriptive city name |
countryCode | string | ISO 3166 alpha-2 country code (e.g. "FR") |
geoid | number | optional numeric geographic ID |
suncalc.GetTimesResult
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
number
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
number
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
number
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
number
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
hours | number |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Date
Kind: instance method of Location
Param | Type |
---|---|
hdate | HDate |
Location
Kind: static method of Location
Param | Type |
---|---|
city | Object |
Represents Parashah HaShavua for an entire Hebrew year
Kind: global class
Array.<string>
string
Object
boolean
Array.<Object>
number
string
Caculates the Parashah HaShavua for an entire Hebrew year
Param | Type | Description |
---|---|---|
hebYr | number | Hebrew year (e.g. 5749) |
il | boolean | Use Israel sedra schedule (false for Diaspora) |
Array.<string>
Returns the parsha (or parshiyot) read on Hebrew date
Kind: instance method of Sedra
Param | Type | Description |
---|---|---|
hDate | HDate | number | Hebrew date or absolute days |
string
Looks up parsha for the date, then returns a (translated) string
Kind: instance method of Sedra
Param | Type | Description |
---|---|---|
hDate | HDate | number | Hebrew date or absolute days |
Object
Returns an object describing the parsha on the first Saturday on or after absdate
Kind: instance method of Sedra
Param | Type | Description |
---|---|---|
hDate | HDate | number | Hebrew date or absolute days |
boolean
Checks to see if this day would be a regular parasha HaShavua Torah reading or special holiday reading
Kind: instance method of Sedra
Param | Type | Description |
---|---|---|
hDate | HDate | number | Hebrew date or absolute days |
Array.<Object>
Kind: instance method of Sedra
number
Kind: instance method of Sedra
string
Translates object describing the parsha to a string
Kind: static method of Sedra
Param | Type |
---|---|
parsha | Array.<string> |
enum
Hebrew months of the year (NISAN=1, TISHREI=7)
Kind: global enum
Read only: true
Properties
Name | Type | Default | Description |
---|---|---|---|
NISAN | number | 1 | Nissan / ניסן |
IYYAR | number | 2 | Iyyar / אייר |
SIVAN | number | 3 | Sivan / סיון |
TAMUZ | number | 4 | Tamuz (sometimes Tammuz) / תמוז |
AV | number | 5 | Av / אב |
ELUL | number | 6 | Elul / אלול |
TISHREI | number | 7 | Tishrei / תִשְׁרֵי |
CHESHVAN | number | 8 | Cheshvan / חשון |
KISLEV | number | 9 | Kislev / כסלו |
TEVET | number | 10 | Tevet / טבת |
SHVAT | number | 11 | Sh'vat / שבט |
ADAR_I | number | 12 | Adar or Adar Rishon / אדר |
ADAR_II | number | 13 | Adar Sheini (only on leap years) / אדר ב׳ |
enum
Days of the week (SUN=0, SAT=6)
Kind: global enum
Read only: true
Properties
Name | Type | Default | Description |
---|---|---|---|
SUN | number | 0 | Sunday |
MON | number | 1 | Monday |
TUE | number | 2 | Tuesday |
WED | number | 3 | Wednesday |
THU | number | 4 | Thursday |
FRI | number | 5 | Friday |
SAT | number | 6 | Saturday |
enum
Holiday flags for Event
Kind: global enum
Read only: true
Properties
Name | Type | Default | Description |
---|---|---|---|
CHAG | number | CHAG | Chag, yontiff, yom tov |
LIGHT_CANDLES | number | LIGHT_CANDLES | Light candles before sundown |
YOM_TOV_ENDS | number | YOM_TOV_ENDS | End of holiday (end of Yom Tov) |
CHUL_ONLY | number | CHUL_ONLY | |
IL_ONLY | number | IL_ONLY | |
LIGHT_CANDLES_TZEIS | number | LIGHT_CANDLES_TZEIS | |
CHANUKAH_CANDLES | number | CHANUKAH_CANDLES | |
ROSH_CHODESH | number | ROSH_CHODESH | |
MINOR_FAST | number | MINOR_FAST | |
SPECIAL_SHABBAT | number | SPECIAL_SHABBAT | |
PARSHA_HASHAVUA | number | PARSHA_HASHAVUA | |
DAF_YOMI | number | DAF_YOMI | |
OMER_COUNT | number | OMER_COUNT | |
MODERN_HOLIDAY | number | MODERN_HOLIDAY | |
MAJOR_FAST | number | MAJOR_FAST | |
SHABBAT_MEVARCHIM | number | SHABBAT_MEVARCHIM | |
MOLAD | number | MOLAD | |
USER_EVENT | number | USER_EVENT |
Interface to lookup cities
CityResult
Looks up a city
Kind: static method of cities
Param | Type | Description |
---|---|---|
str | string | city name |
boolean
Returns true if Hebrew year is a leap year
Kind: global function
Param | Type | Description |
---|---|---|
x | number | Hebrew year |
number
Number of months in Hebrew year
Kind: global function
Param | Type | Description |
---|---|---|
x | number | Hebrew year |
number
Number of days in Hebrew month in a given year
Kind: global function
Param | Type | Description |
---|---|---|
month | number | Hebrew month (e.g. months.TISHREI) |
year | number | Hebrew year |
string
Returns an (untranslated) string name of Hebrew month in year
Kind: global function
Param | Type | Description |
---|---|---|
month | number | Hebrew month (e.g. months.TISHREI) |
year | number | Hebrew year |
number
Returns the Hebrew month number
Kind: global function
Param | Type | Description |
---|---|---|
month | number | string | A number, or Hebrew month name string |
number
Days from sunday prior to start of Hebrew calendar to mean conjunction of Tishrei in Hebrew YEAR
Kind: global function
Param | Type | Description |
---|---|---|
hYear | number | Hebrew year |
number
Number of days in the hebrew YEAR
Kind: global function
Param | Type | Description |
---|---|---|
year | number | Hebrew year |
boolean
true if Cheshvan is long in Hebrew YEAR
Kind: global function
Param | Type | Description |
---|---|---|
year | number | Hebrew year |
boolean
true if Kislev is short in Hebrew YEAR
Kind: global function
Param | Type | Description |
---|---|---|
year | number | Hebrew year |
number
Converts Hebrew month string name to numeric
Kind: global function
Param | Type | Description |
---|---|---|
c | string | monthName |
number
Note: Applying this function to d+6 gives us the DAYNAME on or after an absolute day d. Similarly, applying it to d+3 gives the DAYNAME nearest to absolute date d, applying it to d-1 gives the DAYNAME previous to absolute date d, and applying it to d+7 gives the DAYNAME following absolute date d.
Kind: global function
Param | Type |
---|---|
day_of_week | number |
absdate | number |
Array.<number>
Returns an array from start to end
Kind: global function
Param | Type | Default | Description |
---|---|---|---|
start | number | beginning number, inclusive | |
end | number | ending number, inclusive | |
[step] | number | 1 |
boolean
Returns true if the Gregorian year is a leap year
Kind: global function
Param | Type | Description |
---|---|---|
year | number | Gregorian year |
number
Number of days in the Gregorian month for given year
Kind: global function
Param | Type | Description |
---|---|---|
month | number | Gregorian month (1=January, 12=December) |
year | number | Gregorian year |
number
Returns number of days since January 1 of that year
Kind: global function
Param | Type | Description |
---|---|---|
date | Date | Gregorian date |
number
Converts Gregorian date to Julian Day Count
Kind: global function
Param | Type | Description |
---|---|---|
date | Date | Gregorian date |
Date
Converts from Julian Day Count to Gregorian date. See the footnote on page 384 of ``Calendrical Calculations, Part II: Three Historical Calendars'' by E. M. Reingold, N. Dershowitz, and S. M. Clamen, Software--Practice and Experience, Volume 23, Number 4 (April, 1993), pages 383-404 for an explanation.
Kind: global function
Param | Type | Description |
---|---|---|
theDate | number | absolute Julian days |
HDate
Kind: global function
Param | Type |
---|---|
day | number |
t | HDate |
offset | number |
number
Converts Hebrew date to absolute Julian days. The absolute date is the number of days elapsed since the (imaginary) Gregorian date Sunday, December 31, 1 BC.
Kind: global function
Param | Type | Description |
---|---|---|
d | HDate | SimpleHebrewDate | Hebrew Date |
SimpleHebrewDate
Converts Julian days to Hebrew date to absolute Julian days
Kind: global function
Param | Type | Description |
---|---|---|
d | number | absolute Julian days |
Molad
Calculates the molad for a Hebrew month
Kind: global function
Param | Type |
---|---|
year | number |
month | number |
HDate
Calculates a birthday or anniversary (non-yahrzeit). Year must be after original date of anniversary. Returns undefined when requested year preceeds or is same as original year.
Kind: global function
Returns: HDate
- anniversary occurring in hyear
Param | Type | Description |
---|---|---|
hyear | number | Hebrew year |
gdate | Date | HDate | Gregorian or Hebrew date of event |
HDate
Calculates yahrzeit. Year must be after original date of death. Returns undefined when requested year preceeds or is same as original year.
Kind: global function
Returns: HDate
- anniversary occurring in hyear
Param | Type | Description |
---|---|---|
hyear | number | Hebrew year |
gdate | Date | HDate | Gregorian or Hebrew date of death |
DafYomiResult
Returns the Daf Yomi for given date
Kind: global function
Returns: DafYomiResult
- Tractact name and page number
Param | Type | Description |
---|---|---|
gregdate | Date | Gregorian date |
string
Formats (with translation) the dafyomi result as a string like "Pesachim 34"
Kind: global function
Param | Type | Description |
---|---|---|
daf | DafYomiResult | the Daf Yomi |
number
parsha doubler/undoubler
Kind: global function
Param | Type |
---|---|
p | number |
Object
Returns an object describing the parsha on the first Saturday on or after absdate
Kind: global function
Param | Type |
---|---|
year | number |
absDate | number |
string
Kind: global function
Param | Type |
---|---|
day | number |
Map.<string, Array.<Event>>
Returns a Map for the year indexed by HDate.toString()
Kind: global function
Param | Type | Description |
---|---|---|
year | number | Hebrew year |
Kind: inner method of getHolidaysForYear
Param | Type |
---|---|
year | number |
arr | Array.<Object> |
Array.<Event>
Returns an array of Events on this date (or undefined if no events)
Kind: global function
Param | Type | Description |
---|---|---|
date | HDate | Date | number | Hebrew Date, Gregorian date, or absolute Julian date |
string
Kind: global function
Param | Type |
---|---|
timeFormat | Intl.DateTimeFormat |
dt | Date |
Array.<Object>
Kind: global function
Param | Type |
---|---|
hd | HDate |
location | Location |
timeFormat | Intl.DateTimeFormat |
offset | number |
Array.<Object>
Kind: global function
Param | Type |
---|---|
hd | HDate |
location | Location |
timeFormat | Intl.DateTimeFormat |
Event
Kind: global function
Param | Type |
---|---|
e | Event |
hd | HDate |
dow | number |
location | Location |
timeFormat | Intl.DateTimeFormat |
candlesOffset | number |
havdalahOffset | number |
number
Kind: global function
Param | Type |
---|---|
options | HebcalOptions |
Array.<number>
Parse options object to determine start & end days
Kind: global function
Param | Type |
---|---|
options | HebcalOptions |
Array.<number>
Kind: global function
Param | Type |
---|---|
hyear | number |
number
Mask to filter Holiday array
Kind: global function
Param | Type |
---|---|
options | HebcalOptions |
Array.<Event>
Generates a list of holidays
Kind: global function
Param | Type |
---|---|
options | HebcalOptions |
Object
A simple Hebrew date
Kind: global typedef
Properties
Name | Type | Description |
---|---|---|
yy | number | Hebrew year |
mm | number | Hebrew month of year (1=NISAN, 7=TISHREI) |
dd | number | Day of month (1-30) |
Object
Represents an Molad
Kind: global typedef
Properties
Name | Type | Description |
---|---|---|
dow | number | Day of Week (0=Sunday, 6=Saturday) |
hour | number | hour of day (0-23) |
minutes | number | minutes past hour (0-59) |
chalakim | number | parts of a minute (0-17) |
Object
A City result
Kind: global typedef
Properties
Name | Type | Description |
---|---|---|
name | string | Short city name |
latitude | number | |
longitude | number | |
tzid | string | Timezone Identifier (for tzdata/Olson tzdb) |
cc | string | ISO 3166 two-letter country code |
cityName | string | longer city name with US State or country code |
[state] | string | U.S. State name (only if cc='US') |
[geoid] | number | optional numerical geoid |
Object
A Daf Yomi result
Kind: global typedef
Properties
Name | Type | Description |
---|---|---|
name | string | Tractate name |
blatt | number | Page number |
Object
Options to configure which events are returned
Kind: global typedef
Properties
Name | Type | Description |
---|---|---|
location | Location | latitude/longitude/tzid used for candle-lighting |
year | number | Gregorian or Hebrew year |
isHebrewYear | boolean | to interpret year as Hebrew year |
month | number | Gregorian or Hebrew month (to filter results to a single month) |
numYears | number | generate calendar for multiple years (default 1) |
candlelighting | boolean | calculate candle-lighting and havdalah times |
candleLightingMins | number | minutes before sundown to light candles (default 18) |
havdalahMins | number | minutes after sundown for Havdalah (typical values are 42, 50, or 72) |
havdalahTzeit | boolean | calculate Havdalah according to Tzeit Hakochavim - Nightfall (the point when 3 small stars are observable in the night time sky with the naked eye). Defaults to true unless havdalahMins is specified |
sedrot | boolean | calculate parashah hashavua on Saturdays |
il | boolean | Israeli holiday and sedra schedule |
noMinorFast | boolean | suppress minor fasts |
noModern | boolean | suppress modern holidays |
noRoshChodesh | boolean | suppress Rosh Chodesh & Shabbat Mevarchim |
noSpecialShabbat | boolean | suppress Special Shabbat |
noHolidays | boolean | suppress regular holidays |
dafyomi | boolean | include Daf Yomi |
omer | boolean | include Days of the Omer |
molad | boolean | include event announcing the molad |
ashkenazi | boolean | use Ashkenazi transliterations for event titles (default Sephardi transliterations) |
locale | string | translate event titles according to a locale (one of fi , fr , he , hu , pl , ru , ashkenazi , ashkenazi_litvish , ashkenazi_poylish , ashkenazi_standard ) |
hour12 | boolean | use 12-hour time (1-12) instead of default 24-hour time (0-23) |
FAQs
A perpetual Jewish Calendar API
The npm package @hebcal/core receives a total of 1,952 weekly downloads. As such, @hebcal/core popularity was classified as popular.
We found that @hebcal/core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.