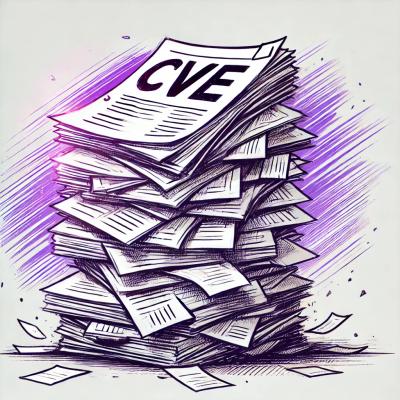
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@homeappcorporate/entity-manager
Advanced tools
Description
$ yarn add @homeappcorporate/entity-manager
or
$ npm install @homeappcorporate/entity-manager --save
For initialization, you need to create an instance of the EntityManager
and implement reducer from instance
Example:
import { combineReducers, applyMiddleware, createStore } from 'redux';
import { composeWithDevTools } from 'redux-devtools-extension';
import thunkMiddleware from 'redux-thunk';
import { EntityManager } from '@homeapp/entity-manager';
const entities = new EntityManager({ id: 'entities', props: {}, config: [] });
const rootReducer = combineReducers({ [entities.getId()]: entities.getReducer() });
const middleware = composeWithDevTools(applyMiddleware(thunkMiddleware));
const makeStore = () => createStore(rootReducer, {}, middleware);
Parameter | Type | Default | Required | Description |
---|---|---|---|---|
id | string | entities | no | Store key |
props | Props | no | Common properties for all entities | |
config | Array<Entity> | no | Config for all entities |
Method | Description |
---|---|
getId() | Returns a store key |
getReducer() | Returns a reducer |
getEntity(entityId) | Returns an instance of entity — entityId (required): string Inner entity id |
cloneEntity(sourceEntityId, entityId) | Returns a cloned copy of source entity — sourceEntityId (required): string Source inner entity id— entityId (required): string Inner entity id for cloned instance |
setParams(params) | Updates the entity params — params (required): object Common headers for api todo: params type |
After initialization you can use getEntity
method to return an entity instance
Example:
const entity = entities.getEntity('ENTITY_ID');
entity.fetch();
for cloneEntity
const firstEntity = entities.cloneEntity('SOURCE_ENTITY_ID', 'INNER_ENTITY_ID_1');
const secondEntity = entities.cloneEntity('SOURCE_ENTITY_ID', 'INNER_ENTITY_ID_2');
Method | Description |
---|---|
clean() | Returns DispatchAction Cleans state for current entity |
getIsLoading(state) | Returns loading status boolean — state (required): Store |
getIsSuccess(state) | Returns isSuccess status boolean — state (required): Store |
getTimestamp(state) | Returns timestamp of last completed request number — state (required): Store |
getErrors(state) | Returns array of errors `Array |
getHasErrors(state) | Returns hasErrors status boolean — state (required): Store |
create(data, successCallback, failCallback) | Returns DispatchAction — data (required): Object — successCallback (not required): SuccessCallback — failCallback (not required): FailCallback Send POST to baseUrl with body = data |
update(id, data, successCallback, failCallback) | Returns DispatchAction — id (required): string — data (required): Object — successCallback (not required): SuccessCallback — failCallback (not required): FailCallback Send PUT to baseUrl + /[id] with body = data |
patch(id, data, successCallback, failCallback) | Returns DispatchAction — id (required): string — data (required): Object — successCallback (not required): SuccessCallback — failCallback (not required): FailCallback Send PATCH to baseUrl + /[id] with body = data . |
delete(id, successCallback, failCallback) | Returns DispatchAction — id (required): string — successCallback (not required): SuccessCallback — failCallback (not required): FailCallback Send DELETE to baseUrl + /[id] with body = data . |
Method | Description |
---|---|
getOne(state, id) | Returns data by id Object — state (required): Store — id (required): string id of data you want to get |
getUniqueData(state) | Returns array of unique data Array<Object> — state (required): Store |
initialServerFetch(id) | Returns DispatchAction — id (required): string fetch initial data (method for server side) |
initialClientFetch(id) | Returns DispatchAction — id (required): string fetch initial data (method for client side) |
fetch() | Returns DispatchAction Send GET to base url |
fetchOne(id) | Returns DispatchAction — id (required): string Send GET to baseUrl + /[id] |
fetchOneByUrl(url) | Returns DispatchAction — url (required): string Send GET to baseUrl + /[url] |
forceFetch() | Returns DispatchAction Same as fetch , ignores shouldCache prop |
(data: ResponseData) => void
(errors: Array<string>) => void
Method | Arguments |
---|---|
init() | Returns DispatchAction set initial state |
initialServerFetch(query) | Returns DispatchAction — query (required): ParsedUrlQueryReturns fetch initial data (method for server side) |
initialClientFetch() | Returns DispatchAction fetch initial data (method for client side) |
fetch() | Returns DispatchAction Send GET to base url |
loadMore() | Returns DispatchAction set page as currentPage + 1 and fetch() with isAppend flag, only for paginationType===page |
loadMoreOffset(limit) | Returns DispatchAction — limit: number set offset as currentOffset + limit and fetch() with isAppend flag, only for paginationType===offset |
setOffset(offset) | Returns DispatchAction — offset (required): number set offset param and fetch() , only for paginationType===offset |
setLimit(limit) | Returns DispatchAction — limit (required): number set limit param and fetch() , only for paginationType===offset |
setOffsetAndLimit(offset, limit) | Returns DispatchAction — offset (required): number — limit (required): number set offset and limit params and fetch() , only for paginationType===offset |
setPage(page) | Returns DispatchAction — page (required): number set page param and fetch() , only for paginationType===page |
setPageSize(pageSize) | Returns DispatchAction — pageSize (required): number set pageSize param and fetch() , only for paginationType===page |
setPagination(page, pageSize) | Returns DispatchAction — page (required): number — pageSize (required): number set page and pageSize params and fetch() , only for paginationType===page |
setFilters(filters) | Returns DispatchAction — filters (required): Object set filters param and fetch() |
setFiltersAndResetPage(filters) | Returns DispatchAction — filters (required): Object set params filters and page to 1 and fetch() , only for paginationType===page |
setFiltersAndResetOffset(filters) | Returns DispatchAction — filters (required): Object set params filters and offset to 0 and fetch() , only for paginationType===offset |
setSorting(sortType, sortDirection) | Returns DispatchAction — sortType (required): string — sortDirection (required): ascend | descend set sortType and sortDirection params and fetch() |
reset() | Returns DispatchAction set params to initialParams and fetch() |
getUniqueData(state) | Returns array of unique data Array<Object> — state (required): Store |
getData(state) | Returns array of data (can be with repetitions) Array<Object> — state (required): Store |
getListState(state) | Returns listState Params — state (required): Store |
getFilters(state) | Returns filters Filters — state (required): Store |
getIsDirtyFilters(state) | Returns is filters changes with initialFilters boolean — state (required): Store |
getPage(state) | Returns page number — state (required): Store |
getPageSize(state) | Returns pageSize number — state (required): Store |
getOffset(state) | Returns offset number — state (required): Store |
getLimit(state) | Returns limit number — state (required): Store |
getSortType(state) | Returns sortType string — state (required): Store |
getSortDirection(state) | Returns sortDirection ascend | descend — state (required): Store |
getTotal(state) | Returns total number — state (required): Store |
getCanLoadMore(state) | Returns if there are more items to be loaded boolean — state (required): Store |
object
Parameter | Type | Default | Required | Description |
---|---|---|---|---|
headers | Object | {} | no | initial headers for api requests |
syncUrlHandler | Function | (query) => history.pushState(null, '', location.pathname + query) | no | Handler will be fired after setting new list state if the shouldSyncUrl flag is true |
defaultParamsSerializer | Function | no | paramsSerializer that would be used during request. Can be over overwritten by paramsSerialzer from Entity Config |
object
Parameter | Type | Default | Required | Description |
---|---|---|---|---|
id | string | yes | id of entity, unique throughout application | |
type | single | list | yes | Type of entity | |
baseUrl | string | yes | baseUrl for api requests | |
headers | Object | no | headers for api requests | |
formatters | Formatters | {} | no | see below |
dependencies | Array<EntityDependency> | [] | no | Dependencies list - entities which will be fetched with the main entity |
shouldCache | boolean | false | no | If that flag is true - only the first fetch will be dispatched, next will be skipped |
shouldSyncUrl | boolean | false | no | Flag for type===list , if true - initialState will be merged with query, all state changes will affect query. |
initialState | ListState | no | Flag for type===list , initial state for list params. | |
paginationType | page | offset | page | no | Flag for type===list , pagination type for list |
paramsSerializer | Function | no | paramsSerializer that would be used during request |
object
Parameter | Type | Default | Required | Description |
---|---|---|---|---|
responseToState | ResponseToState | data => data | no | Transform response data format to state data format. Result will be merged with the result of headersToState |
headersToState | HeadersToState | no | Transform headers to state data format. Result will be merged with the result of responseToState | |
stateToRequestParams | StateToRequestParams | no | Transform list state params to request params | |
stateToRequestBody | StateToRequestBody | no | Transform state data format to request data format | |
queryToState | QueryToState | no | Transform query to list state params | |
stateToQuery | StateToQuery | no | Transform list state params to query |
{
isSuccess: boolean,
errors?: Array<string>,
total?: number,
data: {
ids: Array<string>,
byId: {
[id: string]: Object,
}
}
}
Object => ResponseData
Object => ResponseData
ListState => Object
Object => Object
Object => ListState
ListState => Object
object
Parameter | Type | Default | Required | Description |
---|---|---|---|---|
page | number | 1 | no | only for paginationType===page |
pageSize | number | 20 | no | only for paginationType===page |
offset | number | 0 | no | only for paginationType===offset |
limit | number | 20 | no | only for paginationType===offset |
sortType | string | no | --//-- | |
sortDirection | ascend | descend | ascend | no | --//-- |
filters | Object | {} | no | --//-- |
object
Parameter | Type | Default | Required | Description |
---|---|---|---|---|
id | string | yes | --//-- | |
shouldPrefetch | boolean | yes | if the flag is true main entity will await dependency request to resolve |
Package exports utils for creating formatters:
Used for creating formatters.
type Config = Array<{ from: string, to: string, hadler?: (currentValue: any, data: Object, from: string, to: string) => any}>
type CreateFormater = (Config) => (Object => Object)
FAQs
Description
We found that @homeappcorporate/entity-manager demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.