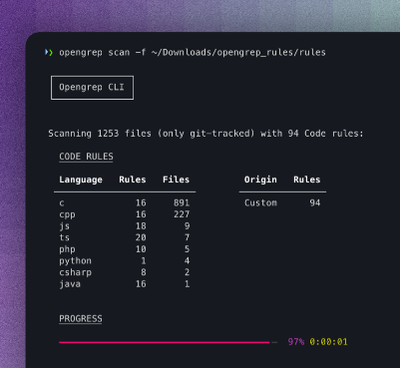
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
@housinganywhere/safe-redux
Advanced tools
NOTE: this library is based on @martin_hotell's rex-tils library and his article Improved Redux type safety with TypeScript 2.8.
Instead of telling the program what types it should use, types are inferred from the implementation, so type checker gets out of our way!
yarn add @housinganywhere/safe-redux
npm i @housinganywhere/safe-redux
Define the actions:
// src/pages/MyPage/actions.ts
import { ActionsUnion, createAction } from '@housinganywhere/safe-redux';
export const INC = '[counter] increment';
export const DEC = '[counter] decrement';
export const INC_BY = '[counter] increment_by';
export const WITH_META = '[counter] with_meta';
export const Actions = {
inc: () => createAction(INC),
dec: () => createAction(DEC),
incBY: (by: number) => createAction(INC_BY, by),
withMeta: (by: number, meta: string) => createAction(WITH_META, by, meta),
};
export type Actions = ActionsUnion<typeof Actions>;
export type ActionTypes =
| typeof INC
| typeof DEC
| typeof INC_BY
| typeof WITH_META;
Handle the actions:
// src/pages/MyPage/reducer.ts
import { handleActions, Handler } from '@housinganywhere/safe-redux';
import { User } from '../types';
import { INC, DEC, INC_BY, WITH_META, Actions, ActionTypes } from './actions';
interface State {
count: number;
}
const initialState: State = {
count: 0,
};
// `Handler` type can be used when you don't want to define the handlers inline
const handleIncBy: Handler<State, typeof INC_BY, Actions> = (
{ count },
{ payload },
) => ({ count: count + payload });
const reducer = handleActions<State, ActionTypes, Actions>(
{
[INC]: ({ count }) => ({ count: count + 1 }),
[DEC]: ({ count }) => ({ count: count - 1 }),
[INC_BY]: handleIncBy,
[WITH_META]: ({ count }, { payload }) => ({ count: count + payload }),
},
initialState,
);
export default reducer;
safe-redux
also provides some type utils to work with Redux.
BindAction
Changes the return type of an action creator to void
. In the context of a
component the only important part of an action is the types of it's arguments.
We don't rely on the return type.
// src/pages/MyPage/actions.ts
import { ActionsUnion, createAction } from '@housinganywhere/safe-redux';
export const INC = '[counter] increment';
export const DEC = '[counter] decrement';
export const INC_BY = '[counter] increment_BY';
export const Actions = {
incBy: (by: number) => createAction(INC_BY, { by }),
};
export type Actions = ActionsUnion<typeof Actions>;
// src/pages/MyPage/MyPage.container.ts
import { connect } from 'react-redux';
import { BindAction } from '@housinganywhere/safe-redux';
import { Actions } from './actions';
import MyPage from './MyPage';
interface StateProps {
count: number;
}
interface DispatchProps {
incBy: BindAction<typeof Actions.incBy>; // (arg: number) => void
}
type MyPageProps = StateProps & DispatchProps;
export default connect<StateProps, DispatchProps>(
(s) => ({ count: s.count }),
{ incBy: Actions.incBy },
)(MyPage);
rex-tils
createAction
are compliant with
flux-standard-actions
,
meaning they have an error
property set to true
when the payload is
instanceof Error
and might have a meta
property.handleActions
to create type safe reducers.safe-redux
only exports a few functions and types:
createAction
and handleActions
.Action
, ActionsUnion
, ActionsOfType
, Handler
and
BindAction
.FAQs
Create and handle safely typed actions
We found that @housinganywhere/safe-redux demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.