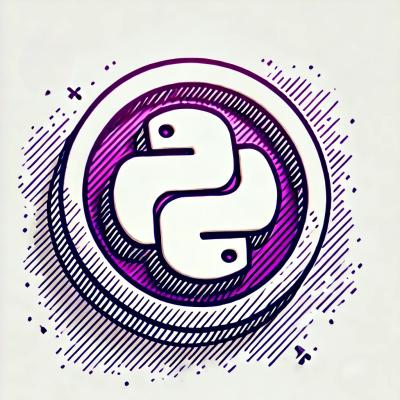
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
@hpke/core
Advanced tools
A Hybrid Public Key Encryption (HPKE) core module for various JavaScript runtimes
Documentation: deno.land | pages (only for the latest ver.)
Using npm:
npm install @hpke/core
Using yarn:
yarn add @hpke/core
Using deno.land:
// use a specific version
import * as hpke from "https://deno.land/x/hpke@1.2.7/core/mod.ts";
// use the latest stable version
import * as hpke from "https://deno.land/x/hpke/core/mod.ts";
Followings are how to use this module with typical CDNs. Other CDNs can be used as well.
Using esm.sh:
<!-- use a specific version -->
<script type="module">
import * as hpke from "https://esm.sh/@hpke/core@1.2.7";
// ...
</script>
<!-- use the latest stable version -->
<script type="module">
import * as hpke from "https://esm.sh/@hpke/core";
// ...
</script>
Using unpkg:
<!-- use a specific version -->
<script type="module">
import * as hpke from "https://unpkg.com/@hpke/core@1.2.7/esm/mod.js";
// ...
</script>
git clone git@github.com:dajiaji/hpke-js.git
cd hpke-js/core
npm install -g esbuild
deno task dnt
deno task minify > $YOUR_SRC_PATH/hpke-core.js
This section shows some typical usage examples.
import {
Aes128Gcm,
CipherSuite,
DhkemP256HkdfSha256,
HkdfSha256,
} from "@hpke/core";
// const {
// Aes128Gcm, CipherSuite, DhkemP256HkdfSha256, HkdfSha256,
// } = require("@hpke/core");
async function doHpke() {
// setup
const suite = new CipherSuite({
kem: new DhkemP256HkdfSha256(),
kdf: new HkdfSha256(),
aead: new Aes128Gcm(),
});
const rkp = await suite.kem.generateKeyPair();
const sender = await suite.createSenderContext({
recipientPublicKey: rkp.publicKey,
});
const recipient = await suite.createRecipientContext({
recipientKey: rkp.privateKey,
enc: sender.enc,
});
// encrypt
const ct = await sender.seal(new TextEncoder().encode("Hello world!"));
// decrypt
const pt = await recipient.open(ct);
// Hello world!
console.log(new TextDecoder().decode(pt));
}
try {
doHpke();
} catch (err) {
console.log("failed:", err.message);
}
import {
Aes128Gcm, CipherSuite, DhkemP256HkdfSha256, HkdfSha256,
} from "https://deno.land/x/hpke@1.2.7/core/mod.ts";
async function doHpke() {
// setup
const suite = new CipherSuite({
kem: new DhkemP256HkdfSha256(),
kdf: new HkdfSha256(),
aead: new Aes128Gcm(),
});
const rkp = await suite.kem.generateKeyPair();
const sender = await suite.createSenderContext({
recipientPublicKey: rkp.publicKey,
});
const recipient = await suite.createRecipientContext({
recipientKey: rkp.privateKey,
enc: sender.enc,
});
// encrypt
const ct = await sender.seal(new TextEncoder().encode("Hello world!"));
// decrypt
const pt = await recipient.open(ct);
// Hello world!
console.log(new TextDecoder().decode(pt));
}
try {
doHpke();
} catch (_err: unknown) {
console.log("doHPKE() failed.");
}
<html>
<head></head>
<body>
<script type="module">
// import * as hpke from "https://esm.sh/hpke-js@1.2.7";
import {
Aes128Gcm, CipherSuite, DhkemP256HkdfSha256, HkdfSha256,
} from "https://esm.sh/@hpke/core@1.2.7";
globalThis.doHpke = async () => {
const suite = new CipherSuite({
kem: new DhkemP256HkdfSha256(),
kdf: new HkdfSha256(),
aead: new Aes128Gcm(),
});
const rkp = await suite.kem.generateKeyPair();
const sender = await suite.createSenderContext({
recipientPublicKey: rkp.publicKey
});
const recipient = await suite.createRecipientContext({
recipientKey: rkp.privateKey, // rkp (CryptoKeyPair) is also acceptable.
enc: sender.enc,
});
// encrypt
const ct = await sender.seal(new TextEncoder().encode("hello world!"));
// decrypt
try {
const pt = await recipient.open(ct);
// hello world!
alert(new TextDecoder().decode(pt));
} catch (err) {
alert("failed to decrypt.");
}
}
</script>
<button type="button" onclick="doHpke()">do HPKE</button>
</body>
</html>
We welcome all kind of contributions, filing issues, suggesting new features or sending PRs.
FAQs
A Hybrid Public Key Encryption (HPKE) core module for various JavaScript runtimes
The npm package @hpke/core receives a total of 4,338 weekly downloads. As such, @hpke/core popularity was classified as popular.
We found that @hpke/core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.