
A Hyperapp higher-order app
that logs state updates and action information to the console.
Getting Started
This example shows a counter that can be incremented or decremented. Go ahead and try it online with your browser console open to see the log messages.
import { h, app } from "hyperapp"
import { withLogger } from "@hyperapp/logger"
const state = {
count: 0
}
const actions = {
down: () => state => ({ count: state.count - 1 }),
up: () => state => ({ count: state.count + 1 })
}
const view = (state, actions) => (
<main>
<h1>{state.count}</h1>
<button onclick={actions.down} disabled={state.count <= 0}>
ー
</button>
<button onclick={actions.up}>+</button>
</main>
)
withLogger(app)(state, actions, view, document.body)
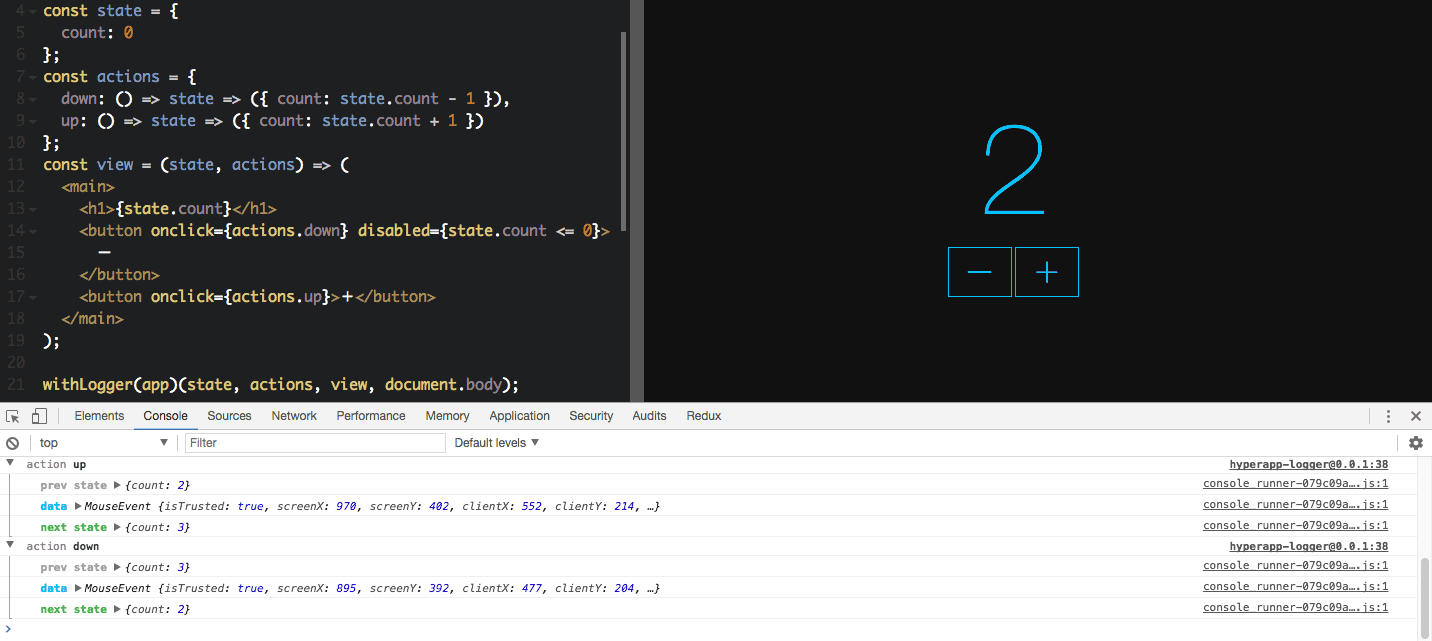
Installation
Node.js
Install with npm / Yarn.
npm i @hyperapp/logger
Then with a module bundler like rollup or webpack use as you would anything else.
import { withLogger } from "@hyperapp/logger"
Browser
Download the minified library from the CDN.
<script src="https://unpkg.com/@hyperapp/logger"></script>
You can find the library in window.hyperappLogger
.
const { withLogger } = hyperappLogger
Usage
Compose the withLogger
function with your app
before calling it with the usual arguments.
import { withLogger } from "@hyperapp/logger"
withLogger(app)(state, actions, view, document.body)
withLogger(options)(app)(state, actions, view, document.body)
Options
options.log
Use it to customize the log function.
withLogger({
log(prevState, action, nextState) {
}
})(app)(state, actions, view, document.body)
License
Hyperapp Logger is MIT licensed. See LICENSE.