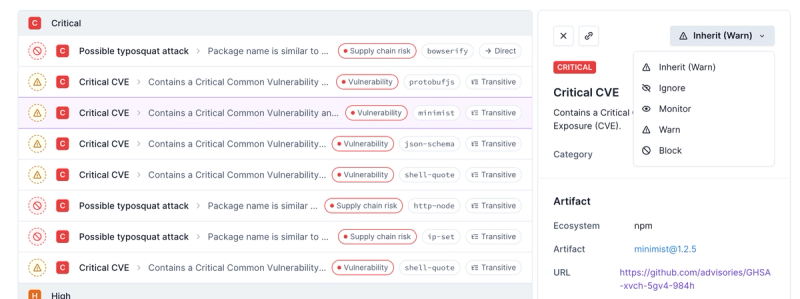
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
@ideal-postcodes/core-browser
Advanced tools
Changelog
Readme
JavaScript browser client for api.ideal-postcodes.co.uk
@ideal-postcodes/core-browser
is the JavaScript browser client for api.ideal-postcodes.co.uk
Our JavaScript client implements a common interface defined at @ideal-postcodes/core-interface
. In depth client documentation can be found at core-interface.ideal-postcodes.dev.
Tested against a suite of modern and legacy, mobile and desktop browsers.
This library is transpiled to modern ES6 and modules. It should be consumed by a bundler or transpiler (e.g. webpack, parcel, rollup) for minification, module resolution and specific browser support.
To support legacy browsers, you will also need to include fetch
polyfill and ES3 transpilation.
npm install @ideal-postcodes/core-browser
import { Client } from "@ideal-postcodes/core-browser"
const client = new Client({ api_key: "iddqd" });
const addresses = await client.lookupPostcode({ postcode: "SW1A2AA" });
const { IdpcRequestFailedError } = Client.errors;
try {
await client.lookupAddress({ query: "10 downing street" });
} catch (error) {
if (error instanceof IdpcRequestFailedError) {
// IdpcRequestFailedError indicates a 402 response code
// Possibly the key balance has been depleted
}
}
core-browser
uses the Fetch API under the hood to facilitate HTTP requests.
A configuration object can be passed to the Client
constructor as a second argument to override certain attributes on the Request of all subsequent fetch requests. Request configuration is documented here.
If you need to support older browsers, please include a fetch polyfill and transpile this library down to ES3. Alternatively use one of our pre-transpiled outputs at ideal-postcodes/core-browser-bundled.
The client exposes a number of simple methods to get at the most common tasks when interacting with the API. Below is a (incomplete) list of commonly used methods.
For a complete list of client methods, including low level resource methods, please see the core-interface documentation
Return addresses associated with a given postcode
const postcode = "id11qd";
const addresses = await client.lookupPostcode({ postcode });
Return addresses associated with a given query
const query = "10 downing street sw1a";
const addresses = await client.lookupAddress({ query });
Return address for a given udprn
Invalid UDPRN will return null
const udprn = 23747771;
const address = await client.lookupUdprn({ udprn });
npm test
MIT
FAQs
Browser javascript client for api.ideal-postcodes.co.uk
The npm package @ideal-postcodes/core-browser receives a total of 3,671 weekly downloads. As such, @ideal-postcodes/core-browser popularity was classified as popular.
We found that @ideal-postcodes/core-browser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.