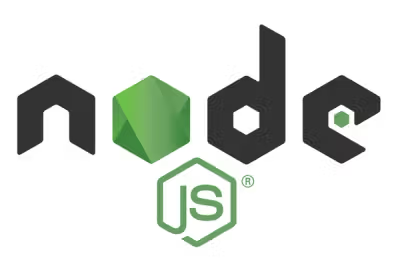
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
@iguilhermeluis/sharepoint-orm-mod
Advanced tools
# NPM
npm install --save @jorgejr568/sharepoint-orm
# Yarn
yarn add @jorgejr568/sharepoint-orm
Create an plugins/sharepoint-orm.js file to place your configuration. We'll export two factories, creating our Auth and Builder services.
import { AuthFactory, BuilderFactory} from '@jorgejr568/sharepoint-orm'
const config = {
apiUrl: process.env.API_URL,
spUrl: process.env.SHAREPOINT_URL,
tokenApi: process.env.API_TOKEN,
application: process.env.APP_NAME, //'[uppercase and only letters portal name]',
environment: process.env.APP_ENVIRONMENT //'DEV or PRD'
}
export const spAuth = AuthFactory(config)
export const spBuilder = BuilderFactory(config)
import { spAuth } from '@/plugins/sharepoint-orm'
// sync
spAuth
.authorize()
.then(token => {
// Token is automatically stored on spAuth
console.log({ token })
spAuth.current().then(user => {
// Get current sharepoint user
console.log({ user })
})
})
.catch(e => {
// Authorization error
console.error({ e })
})
// async
try{
const token = await spAuth.authorize()
console.log({ token })
const user = await spAuth.current()
console.log({ user })
}
catch(e){
console.error({ e })
}
import { spAuth } from '@/plugins/sharepoint-orm'
try{
const token = await spAuth.authorize()
console.log({ token })
setInterval(async () => {
const newToken = await spAuth.refreshToken()
// Token is automatically updated on spAuth
console.log({ newToken })
}, 2 * 60 * 1000) // Each 2 minutes
}
catch(e){
// Authorization error
console.error({ e })
}
import { spAuth, spBuilder } from '@/plugins/sharepoint-orm'
await spAuth.authorize()
const items = await spBuilder.table('ListName').select('*').get()
console.log({ items })
import { spAuth, spBuilder } from '@/plugins/sharepoint-orm'
await spAuth.authorize()
const items = await spBuilder
.table('ListName')
.select('*')
.where('Id', 'eq', 1)
.get()
console.log({ items })
// And condition
const items = await spBuilder
.table('ListName')
.select('*')
.where('Id', 'eq', 1)
.where('Title', 'eq', 'Player name')
.get()
console.log({ items })
import { spAuth, spBuilder } from '@/plugins/sharepoint-orm'
await spAuth.authorize()
const items = await spBuilder
.table('ListName')
.select('*')
.where('Id', 'eq', 1)
.orWhere('Id', 'eq', 2)
.get()
console.log({ items })
import { spAuth, spBuilder } from '@/plugins/sharepoint-orm'
await spAuth.authorize()
const items = await spBuilder
.table('ListName')
.select('*')
.whereNot('Id', 'eq', 1)
.get()
console.log({ items })
import { spAuth, spBuilder } from '@/plugins/sharepoint-orm'
await spAuth.authorize()
const items = await spBuilder
.table('ListName')
.select('*')
.whereNot('Id', 'eq', 1)
.orWhereNot('Title', 'eq', 'Player name')
.get()
console.log({ items })
import { spAuth, spBuilder } from '@/plugins/sharepoint-orm'
await spAuth.authorize()
const items = await spBuilder
.table('ListName')
.select('*')
.whereRaw('Id eq 1')
.get()
console.log({ items })
import { spAuth, spBuilder } from '@/plugins/sharepoint-orm'
await spAuth.authorize()
const items = await spBuilder
.table('ListName')
.select('*')
.whereRaw('Id eq 1')
.orWhereRaw(`Title eq 'Player name'`)
.get()
console.log({ items })
// List all items
import { spAuth, spBuilder } from '@/plugins/sharepoint-orm'
await spAuth.authorize()
const items = await spBuilder.table('ListName').select('*').get()
console.log({ items })
// Get only first item or undefined
import { spAuth, spBuilder } from '@/plugins/sharepoint-orm'
await spAuth.authorize()
const item = await spBuilder
.table('ListName')
.select('*')
.where('Id', 'eq', 1)
.first()
console.log({ item })
import { spAuth, spBuilder } from '@/plugins/sharepoint-orm'
await spAuth.authorize()
const insertedId = await spBuilder
.table('ListName')
.insertGetId({
Title: 'Player name'
})
console.log({ insertedId })
import { spAuth, spBuilder } from '@/plugins/sharepoint-orm'
await spAuth.authorize()
// Void return
await spBuilder
.table('ListName')
.where('Id', 'eq', 1)
.update({
Title: 'New player name'
})
import { spAuth, spBuilder } from '@/plugins/sharepoint-orm'
await spAuth.authorize()
// Void return
await spBuilder
.table('ListName')
.where('Id', 'eq', 1)
.delete()
FAQs
Lib to consume sharepoint using axios
The npm package @iguilhermeluis/sharepoint-orm-mod receives a total of 1 weekly downloads. As such, @iguilhermeluis/sharepoint-orm-mod popularity was classified as not popular.
We found that @iguilhermeluis/sharepoint-orm-mod demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.