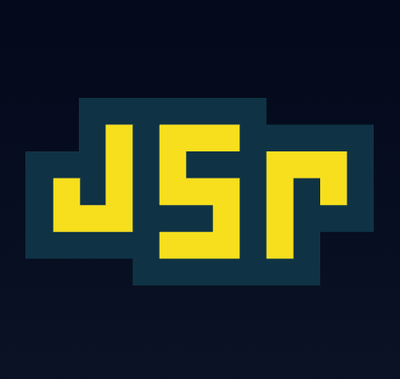
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@ionic/utils-subprocess
Advanced tools
@ionic/utils-subprocess is a utility package designed to facilitate the execution and management of subprocesses in Node.js applications. It provides a set of tools to spawn, manage, and interact with child processes, making it easier to handle tasks that require running external commands or scripts.
Spawning a Subprocess
This feature allows you to spawn a new subprocess to run a command. In this example, the 'ls -la' command is executed, and the output is logged to the console.
const { spawn } = require('@ionic/utils-subprocess');
async function runCommand() {
const subprocess = spawn('ls', ['-la']);
subprocess.stdout.on('data', (data) => {
console.log(`stdout: ${data}`);
});
subprocess.stderr.on('data', (data) => {
console.error(`stderr: ${data}`);
});
const code = await subprocess;
console.log(`Child process exited with code ${code}`);
}
runCommand();
Handling Subprocess Output
This feature demonstrates how to handle the output of a subprocess. In this example, the 'node -v' command is executed to get the Node.js version, and the output is captured and logged.
const { spawn } = require('@ionic/utils-subprocess');
async function runCommand() {
const subprocess = spawn('node', ['-v']);
let output = '';
subprocess.stdout.on('data', (data) => {
output += data;
});
const code = await subprocess;
console.log(`Node.js version: ${output.trim()}`);
}
runCommand();
Error Handling in Subprocess
This feature shows how to handle errors when spawning a subprocess. In this example, an attempt is made to run a nonexistent command, and the error is caught and logged.
const { spawn } = require('@ionic/utils-subprocess');
async function runCommand() {
try {
const subprocess = spawn('nonexistent-command');
subprocess.stdout.on('data', (data) => {
console.log(`stdout: ${data}`);
});
subprocess.stderr.on('data', (data) => {
console.error(`stderr: ${data}`);
});
const code = await subprocess;
console.log(`Child process exited with code ${code}`);
} catch (error) {
console.error(`Failed to start subprocess: ${error.message}`);
}
}
runCommand();
The 'child_process' module is a core Node.js module that provides the ability to spawn child processes. It offers similar functionalities to @ionic/utils-subprocess, such as spawning subprocesses, handling their output, and managing errors. However, @ionic/utils-subprocess provides a more user-friendly API and additional utilities for managing subprocesses.
Execa is a popular npm package for executing shell commands in Node.js. It provides a promise-based API and additional features like improved error handling and better support for cross-platform compatibility. Compared to @ionic/utils-subprocess, Execa offers a more modern and feature-rich API for managing subprocesses.
Cross-spawn is an npm package that provides a cross-platform solution for spawning child processes. It addresses issues with the default 'child_process' module on Windows and offers a consistent API for spawning subprocesses across different operating systems. While @ionic/utils-subprocess also aims to simplify subprocess management, cross-spawn focuses specifically on cross-platform compatibility.
FAQs
Subprocess utils for NodeJS
The npm package @ionic/utils-subprocess receives a total of 283,829 weekly downloads. As such, @ionic/utils-subprocess popularity was classified as popular.
We found that @ionic/utils-subprocess demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.