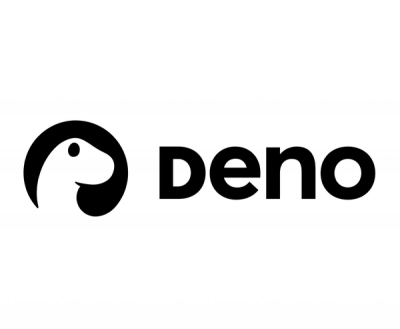
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@jaybirdgroup/jb-file-storage
Advanced tools
A storage interface to work with local file storage, s3, youtube and vimeo
A file storage interface to work with Local File Storage and S3.
Additionally, video files can bу uploaded to the Youtube or Vimeo
Install package.
$ npm i jb-file-storage --save
Work with Local storage.
const Storage = require('jb-file-storage');
const Fs = require('fs');
const LocalStorage = new Storage('local', {
basePath: './public/uploads/',
baseUrl: 'http://localhost:8080/public/uploads/'
});
// Path to file
let input = '/path/to/source/file.txt';
// OR read stream
input = Fs.createReadStream(input);
LocalStorage.saveFile(input, 'path/to/dest/file.txt', 'text/plain', (err, result) => {
if (err) {
return console.log(err);
}
console.log(result);
// Example result
// {
// url: 'http://localhost:8080/public/uploads/path/to/dest/file.txt',
// path: 'path/to/dest/file.txt'
// }
});
// Note: the same arguments and response parameters for method LocalStorage.saveFileOnce().
// Note: basePath will be prepended to the file path
LocalStorage.deleteFile('path/to/file.txt', (err) => {
if (err) {
return console.log(err);
}
console.log('success!')
});
// Note: Work only for S3 and Local storage
// Note: basePath will be prepended to the folder path
LocalStorage.deleteDir('path/to/folder', (err) => {
if (err) {
return console.log(err);
}
console.log('success!')
});
You need to create S3 Bucket and get Access Key and Secret Access Key. See Managing Access Keys for Your AWS Account guide.
const Storage = require('jb-file-storage');
const S3Storage = new Storage('s3', {
basePath: 'project/uploads/',
baseUrl: 'https://{bucket}.s3.amazonaws.com/project/uploads/',
s3config: {
accessKeyId: '{access-key}',
secretAccessKey: '{secret-access-key}',
params: {
Bucket: '{bucket}',
ACL: 'public-read'
}
}
});
// use the same api for all storages
// Also for youtube available different behaviour for saveFileOnce().
// Method prevent re-uploading in case of file existence
Read Obtaining authorization credentials to get Client Id and App Secret.
To make Youtube upload work, we need to authorize API requests with oAuth Token.
Add your domain URI to the Authorized JavaScript origins. For example: http://localhost:8080
.
Add callback URI http://localhost:8080/api/settings/youtubeoauth/callback
to the Authorized redirect URIs.
TODO: Add description on how to retrieve Youtube Access Token and routes configuration
const Storage = require('jb-file-storage');
const YoutubeStorage = new Storage('youtube', {
baseUrl: 'https://www.youtube.com',
type: 'oauth',
auth: {
accessToken: '{oauth-access-token}'
},
apiConfig: {
clientId: '{client-id}',
appSecret: '{app-secret}'
}
});
// use the same api for all storages
Read Getting Started guide to get API Config values.
const Storage = require('jb-file-storage');
const VimeoStorage = new Storage('vimeo', {
baseUrl: 'https://player.vimeo.com/video/',
apiConfig: {
clientId: '{client-id}',
appSecret: '{app-secret}',
accessToken: '{access-token}'
}
});
// use the same api for all storages
FAQs
A storage interface to work with local file storage, s3, youtube and vimeo
The npm package @jaybirdgroup/jb-file-storage receives a total of 19 weekly downloads. As such, @jaybirdgroup/jb-file-storage popularity was classified as not popular.
We found that @jaybirdgroup/jb-file-storage demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.