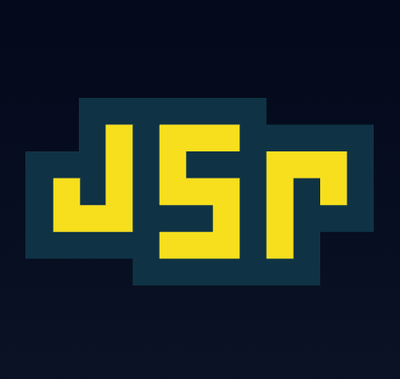
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@jimp/plugin-color
Advanced tools
@jimp/plugin-color is a plugin for the Jimp image processing library that provides various color manipulation functionalities. It allows users to perform operations such as adjusting brightness, contrast, and applying color filters to images.
Adjust Brightness
This feature allows you to adjust the brightness of an image. The value can range from -1 (darken) to 1 (brighten).
const Jimp = require('jimp');
Jimp.read('path/to/image.jpg').then(image => {
image.brightness(0.5) // increase brightness by 50%
.write('path/to/output.jpg');
});
Adjust Contrast
This feature allows you to adjust the contrast of an image. The value can range from -1 (decrease contrast) to 1 (increase contrast).
const Jimp = require('jimp');
Jimp.read('path/to/image.jpg').then(image => {
image.contrast(0.5) // increase contrast by 50%
.write('path/to/output.jpg');
});
Apply Color Filter
This feature allows you to apply a color filter to an image. You can specify the color and the intensity of the filter.
const Jimp = require('jimp');
Jimp.read('path/to/image.jpg').then(image => {
image.color([{ apply: 'red', params: [100] }]) // apply red filter
.write('path/to/output.jpg');
});
Sharp is a high-performance image processing library that supports various color manipulation functionalities such as brightness, contrast, and color filters. It is known for its speed and efficiency compared to Jimp.
GraphicsMagick (gm) is a powerful image processing library that provides extensive color manipulation features. It is more feature-rich and can handle a wider range of image formats compared to Jimp.
Image-js is a versatile image processing library that offers various color manipulation functionalities. It is designed to be easy to use and integrates well with other JavaScript libraries.
Utils for jimp extensions.
Apply multiple color modification rules
import jimp from "jimp";
async function main() {
const image = await jimp.read("test/image.png");
image.color([{ apply: "red", params: [100] }]);
}
main();
Jimp supports advanced colour manipulation using a single method as follows:
image.color([
{ apply: "hue", params: [-90] },
{ apply: "lighten", params: [50] },
{ apply: "xor", params: ["#06D"] },
]);
The method supports the following modifiers:
Modifier | Description |
---|---|
lighten {amount} | Lighten the color a given amount, from 0 to 100. Providing 100 will always return white (works through TinyColor) |
brighten {amount} | Brighten the color a given amount, from 0 to 100 (works through TinyColor) |
darken {amount} | Darken the color a given amount, from 0 to 100. Providing 100 will always return black (works through TinyColor) |
desaturate {amount} | Desaturate the color a given amount, from 0 to 100. Providing 100 will is the same as calling greyscale (works through TinyColor) |
saturate {amount} | Saturate the color a given amount, from 0 to 100 (works through TinyColor) |
greyscale {amount} | Completely desaturates a color into greyscale (works through TinyColor) |
spin {degree} | Spin the hue a given amount, from -360 to 360. Calling with 0, 360, or -360 will do nothing - since it sets the hue back to what it was before. (works through TinyColor) |
hue {degree} | Alias for spin |
mix {color, amount} | Mixes colors by their RGB component values. Amount is opacity of overlaying color |
tint {amount} | Same as applying mix with white color |
shade {amount} | Same as applying mix with black color |
xor {color} | Treats the two colors as bitfields and applies an XOR operation to the red, green, and blue components |
red {amount} | Modify Red component by a given amount |
green {amount} | Modify Green component by a given amount |
blue {amount} | Modify Blue component by a given amount |
Adjusts the brightness of the image
import jimp from "jimp";
async function main() {
const image = await jimp.read("test/image.png");
image.brightness(20);
}
main();
Adjusts the contrast of the image
import jimp from "jimp";
async function main() {
const image = await jimp.read("test/image.png");
image.contrast(70);
}
main();
Apply a posterize effect
import jimp from "jimp";
async function main() {
const image = await jimp.read("test/image.png");
image.posterize(5);
}
main();
Multiplies the opacity of each pixel by a factor between 0 and 1
import jimp from "jimp";
async function main() {
const image = await jimp.read("test/image.png");
image.opacity(80);
}
main();
Applies a sepia tone to the image
import jimp from "jimp";
async function main() {
const image = await jimp.read("test/image.png");
image.sepia();
}
main();
Fades each pixel by a factor between 0 and 1
import jimp from "jimp";
async function main() {
const image = await jimp.read("test/image.png");
image.fade(0.7);
}
main();
Sum neighbor pixels weighted by the kernel matrix. You can find a nice explanation with examples at GIMP's Convolution Matrix plugin
import jimp from "jimp";
async function main() {
const image = await jimp.read("test/image.png");
image.convolution(
[
[-1, -1, -1],
[-1, 8, -1],
[-1, -1, -1],
],
jimp.EDGE_EXTEND
);
}
main();
Set the alpha channel on every pixel to fully opaque
import jimp from "jimp";
async function main() {
const image = await jimp.read("test/image.png");
image.opaque();
}
main();
Pixelates the image or a region
import jimp from "jimp";
async function main() {
const image = await jimp.read("test/image.png");
image.pixelate(10);
}
main();
Applies a convolution kernel to the image or a region
import jimp from "jimp";
async function main() {
const image = await jimp.read("test/image.png");
// make me better
image.pixelate(kernal);
}
main();
import jimp from "jimp";
async function main() {
const image = await jimp.read("test/image.png");
image.normalize();
}
main();
import jimp from "jimp";
async function main() {
const image = await jimp.read("test/image.png");
image.invert();
}
main();
FAQs
Unknown package
The npm package @jimp/plugin-color receives a total of 967,881 weekly downloads. As such, @jimp/plugin-color popularity was classified as popular.
We found that @jimp/plugin-color demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.