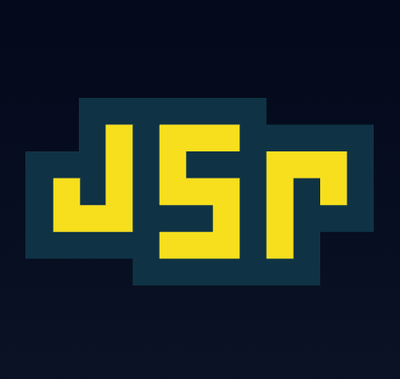
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@jimp/plugin-resize
Advanced tools
@jimp/plugin-resize is a plugin for the Jimp image processing library that provides functionality for resizing images. It allows you to scale images up or down, fit them within specific dimensions, and apply various resizing algorithms.
Resize to specific dimensions
This feature allows you to resize an image to specific dimensions. In this example, the image is resized to 256x256 pixels.
const Jimp = require('jimp');
Jimp.read('path/to/image.jpg')
.then(image => {
return image.resize(256, 256) // resize to 256x256
.write('path/to/resized_image.jpg'); // save
})
.catch(err => {
console.error(err);
});
Scale image proportionally
This feature allows you to scale an image proportionally. In this example, the image is scaled to 50% of its original size.
const Jimp = require('jimp');
Jimp.read('path/to/image.jpg')
.then(image => {
return image.scale(0.5) // scale image to 50%
.write('path/to/scaled_image.jpg'); // save
})
.catch(err => {
console.error(err);
});
Contain image within specific dimensions
This feature allows you to resize an image to fit within specific dimensions while maintaining the aspect ratio. In this example, the image is resized to fit within a 300x300 pixel box.
const Jimp = require('jimp');
Jimp.read('path/to/image.jpg')
.then(image => {
return image.contain(300, 300) // contain within 300x300
.write('path/to/contained_image.jpg'); // save
})
.catch(err => {
console.error(err);
});
Sharp is a high-performance image processing library that supports resizing, cropping, and various other transformations. It is known for its speed and efficiency, especially with large images. Compared to @jimp/plugin-resize, Sharp offers more advanced features and better performance.
GraphicsMagick (gm) is a powerful image processing library that provides a wide range of image manipulation capabilities, including resizing. It is a wrapper around the GraphicsMagick and ImageMagick tools. While it offers extensive functionality, it requires the installation of external dependencies, unlike @jimp/plugin-resize which is a pure JavaScript solution.
Lightweight Image Processor (lwip) is a simple and efficient image processing library that supports resizing, cropping, and other basic transformations. It is lightweight and easy to use, but it may not offer as many features or the same level of performance as @jimp/plugin-resize or Sharp.
Resize an image.
Resizes the image to a set width and height using a 2-pass bilinear algorithm/
import jimp from 'jimp';
async function main() {
// Read the image.
const image = await jimp.read('test/image.png');
// Resize the image to width 150 and auto height.
await image.resize(150, jimp.AUTO);
// Save and overwrite the image
await image.writeAsync('test/image.png');
}
main();
Jimp.AUTO
can be passes to either the height or width and jimp will scale the image accordingly. Jimp.AUTO
cannot be both height and width.
// resize the height to 250 and scale the width accordingly
image.resize(Jimp.AUTO, 250);
// resize the width to 250 and scale the height accordingly
image.resize(250, Jimp.AUTO);
The default resizing algorithm uses a bilinear method.
Optionally, the following constants can be passed to choose a particular resizing algorithm:
Jimp.RESIZE_NEAREST_NEIGHBOR;
Jimp.RESIZE_BILINEAR;
Jimp.RESIZE_BICUBIC;
Jimp.RESIZE_HERMITE;
Jimp.RESIZE_BEZIER;
image.resize(250, 250, Jimp.RESIZE_BEZIER);
FAQs
Unknown package
The npm package @jimp/plugin-resize receives a total of 1,029,100 weekly downloads. As such, @jimp/plugin-resize popularity was classified as popular.
We found that @jimp/plugin-resize demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.