What is @js-sdsl/ordered-map?
@js-sdsl/ordered-map is a JavaScript library that provides an ordered map data structure. This data structure maintains the order of elements based on their keys, allowing for efficient insertion, deletion, and access operations. It is particularly useful for scenarios where the order of elements is important and you need to perform frequent updates.
What are @js-sdsl/ordered-map's main functionalities?
Insertion
This feature allows you to insert key-value pairs into the ordered map. The elements are stored in the order they are inserted.
const { OrderedMap } = require('@js-sdsl/ordered-map');
const map = new OrderedMap();
map.set('a', 1);
map.set('b', 2);
map.set('c', 3);
console.log(map);
Access
This feature allows you to access the value associated with a specific key in the ordered map.
const { OrderedMap } = require('@js-sdsl/ordered-map');
const map = new OrderedMap();
map.set('a', 1);
map.set('b', 2);
map.set('c', 3);
console.log(map.get('b')); // Output: 2
Deletion
This feature allows you to delete a key-value pair from the ordered map based on the key.
const { OrderedMap } = require('@js-sdsl/ordered-map');
const map = new OrderedMap();
map.set('a', 1);
map.set('b', 2);
map.set('c', 3);
map.delete('b');
console.log(map);
Iteration
This feature allows you to iterate over the key-value pairs in the ordered map in the order they were inserted.
const { OrderedMap } = require('@js-sdsl/ordered-map');
const map = new OrderedMap();
map.set('a', 1);
map.set('b', 2);
map.set('c', 3);
for (const [key, value] of map) {
console.log(key, value);
}
Other packages similar to @js-sdsl/ordered-map
immutable
The 'immutable' package provides immutable data structures, including OrderedMap. It ensures that data cannot be changed once created, which can help prevent bugs and improve performance in certain scenarios. Compared to @js-sdsl/ordered-map, 'immutable' focuses on immutability and offers a broader range of data structures.
sorted-map
The 'sorted-map' package provides a sorted map data structure that maintains the order of elements based on their keys. It offers similar functionality to @js-sdsl/ordered-map but focuses on sorting elements by key rather than maintaining insertion order.
bintrees
The 'bintrees' package provides various binary tree data structures, including a Red-Black Tree, which can be used to implement ordered maps. It offers more control over the underlying tree structure compared to @js-sdsl/ordered-map, which abstracts these details away.
A javascript standard data structure library which benchmark against C++ STL
English | 简体中文
✨ Included data structures
- Stack - first in first out stack.
- Queue - first in last out queue.
- PriorityQueue - heap-implemented priority queue.
- Vector - protected array, cannot to operate properties like
length
directly. - LinkList - linked list of non-contiguous memory addresses.
- Deque - double-ended-queue, O(1) time complexity to
unshift
or getting elements by index. - OrderedSet - sorted set which implemented by red black tree.
- OrderedMap - sorted map which implemented by red black tree.
- HashSet - refer to the polyfill of ES6 Set.
- HashMap - refer to the polyfill of ES6 Map.
⚔️ Benchmark
We are benchmarking against other popular data structure libraries. In some ways we're better than the best library. See benchmark.
🖥 Supported platforms
IE / Edge
|
Firefox
|
Chrome
|
Safari
|
Opera
|
NodeJs
|
Edge 12 | 31 | 49 | 10 | 36 | 10 |
📦 Download
Download directly by cdn:
Or install js-sdsl using npm:
npm install js-sdsl
Or you can download the isolation packages containing only the containers you want:
🪒 Usage
You can visit our official website to get more information.
To help you have a better use, we also provide this API document.
For previous versions of the documentation, please visit:
https://js-sdsl.org/js-sdsl/previous/v${version}/index.html
E.g.
https://js-sdsl.org/js-sdsl/previous/v4.1.5/index.html
For browser
<script src="https://unpkg.com/js-sdsl/dist/umd/js-sdsl.min.js"></script>
<script>
const {
Vector,
Stack,
Queue,
LinkList,
Deque,
PriorityQueue,
OrderedSet,
OrderedMap,
HashSet,
HashMap
} = sdsl;
const myOrderedMap = new OrderedMap();
myOrderedMap.setElement(1, 2);
console.log(myOrderedMap.getElementByKey(1));
</script>
For npm
import { OrderedMap } from 'js-sdsl';
const { OrderedMap } = require('js-sdsl');
const myOrderedMap = new OrderedMap();
myOrderedMap.setElement(1, 2);
console.log(myOrderedMap.getElementByKey(1));
🛠 Test
Unit test
We use jest library to write unit tests, you can see test coverage on coveralls. You can run yarn test:unit
command to reproduce it.
For performance
We tested most of the functions for efficiency. You can go to gh-pages/performance.md
to see our running results or reproduce it with yarn test:performance
command.
You can also visit here to get the result.
⌨️ Development
Use Gitpod, a free online dev environment for GitHub.

Or clone locally:
$ git clone https://github.com/js-sdsl/js-sdl.git
$ cd js-sdsl
$ npm install
$ npm run dev
Then you can see the output in dist/cjs
folder.
🤝 Contributing
Feel free to dive in! Open an issue or submit PRs. It may be helpful to read the Contributor Guide.
Contributors
Thanks goes to these wonderful people:
This project follows the all-contributors specification. Contributions of any kind welcome!
❤️ Sponsors and Backers
The special thanks to these sponsors or backers because they provided support at a very early stage:
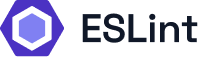
Thanks also give to these sponsors or backers:


🪪 License
MIT © ZLY201