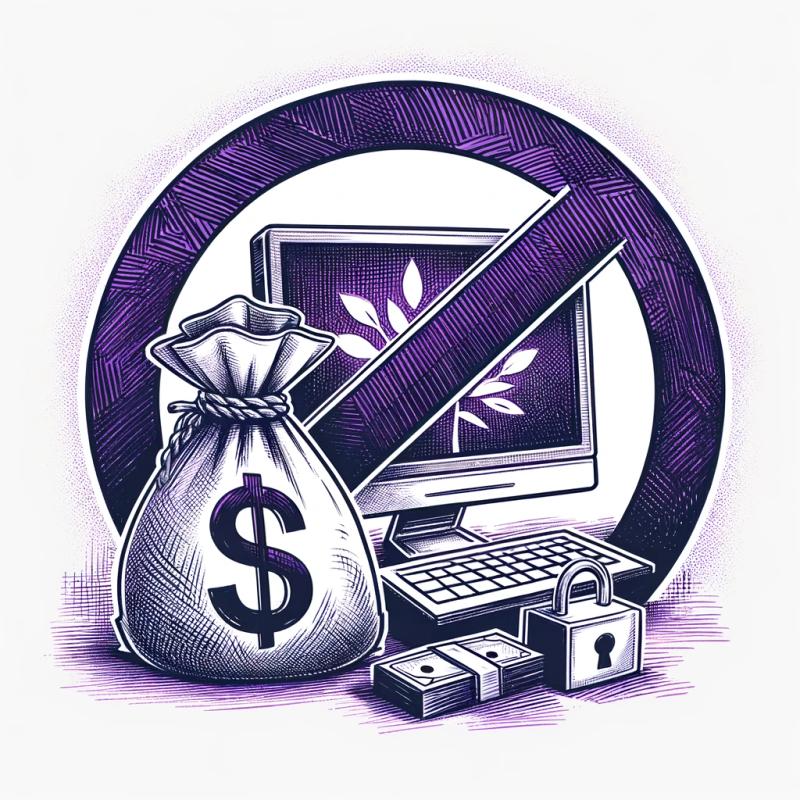
Security News
The Push to Ban Ransom Payments Is Gaining Momentum
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
@jsenv/eslint-config
Advanced tools
Readme
Create ESLint configuration for any project.
Exports dumb ESLint config parts that can be composed together to obtain the eslint config you need. It is used by all jsenv repositories to configure ESLint.
composeEslintConfig
is a function returning an eslint config object being the composition of eslint config objects passed in arguments.
This function helps to create groups while configuring ESLint.
const { composeEslintConfig, eslintConfigBase } = require("@jsenv/eslint-config")
const eslintConfig = composeEslintConfig(
eslintConfigBase,
// first "group": enable html plugin
{
plugins: ["html"],
settings: {
extensions: [".html"],
},
},
// second "group": enable react plugin
{
plugins: ["react"],
settings: {
extensions: [".jsx"],
},
},
)
module.exports = eslintConfig
eslintConfigBase
is an eslint config object declared in src/eslintConfigBase.js. It is a basic ESLint configuration meant to enable es6 features like top level await.
eslintConfigForPrettier
is an eslint config object declared in src/eslintConfigForPrettier.js.
Use this object if your project uses prettier, it will disable all eslint rules already handled by prettier.
Important: This object is forcing a list of eslint rules to "off"
so it must be used after eslint rules are configured. In other words, keep it low, ideally last, in the eslint config composition.
const {
composeEslintConfig,
eslintConfigBase,
eslintConfigForPrettier,
} = require("@jsenv/eslint-config")
const eslintConfig = composeEslintConfig(
eslintConfigBase,
{
rules: {
semi: ["error", "never"],
},
},
eslintConfigForPrettier, // will disable "semi" rule because prettier is handling semi colon during formatting
)
module.exports = eslintConfig
eslintConfigToPreferExplicitGlobals
is an eslint config object declared in src/eslintConfigToPreferExplicitGlobals.js.
If your code uses a variable named close
, eslint consider it as defined by default because there is a window.close
function. This behaviour prevents you to catch bugs because most of the time you didn't want to use window.*
. Adding eslintConfigToPreferExplicitGlobals
to your eslint config means close
can be reported as not defined and forces to write explicitely write window.close
if that's what you want to use.
const {
composeEslintConfig,
eslintConfigBase,
eslintConfigToPreferExplicitGlobals,
} = require("@jsenv/eslint-config")
const eslintConfig = composeEslintConfig(eslintConfigBase, eslintConfigToPreferExplicitGlobals)
module.exports = eslintConfig
jsenvEslintRules
is a list of eslint rules declared in src/jsenvEslintRules.js.
Use jsenvEslintRules
to reuse the eslint configuration used in jsenv codebase. You can still modify some rules to you convenience as shown below:
const { composeEslintConfig, jsenvEslintRules } = require("@jsenv/eslint-config")
const eslintConfig = composeEslintConfig(eslintConfigBase, {
rules: {
...jsenvEslintRules,
semi: ["error", "always"],
},
})
module.exports = eslintConfig
jsenvEslintRulesForImport
is a list of eslint rules declared in src/jsenvEslintRulesForImport.js.
It can be used to configure eslint-plugin-import with jsenv rules.
npm install --save-dev eslint-plugin-import
const { composeEslintConfig, jsenvEslintRulesForImport } = require("@jsenv/eslint-config")
const eslintConfig = composeEslintConfig(eslintConfigBase, {
plugins: ["import"],
settings: {
"import/resolver": {
node: {},
},
},
rules: jsenvEslintRulesForImport,
})
module.exports = eslintConfig
jsenvEslintRulesForReact
is a list of eslint rules declared in src/jsenvEslintRulesForReact.js.
It can be used to configure eslint-plugin-react with jsenv rules.
npm install --save-dev eslint-plugin-react
const { composeEslintConfig, jsenvEslintRulesForReact } = require("@jsenv/eslint-config")
const eslintConfig = composeEslintConfig(eslintConfigBase, {
plugins: ["react"],
rules: jsenvEslintRulesForReact,
})
module.exports = eslintConfig
The following ESLint configuration does the following:
const {
composeEslintConfig,
eslintConfigBase,
eslintConfigForPrettier,
jsenvEslintRules,
jsenvEslintRulesForImport,
} = require("@jsenv/eslint-config")
const eslintConfig = composeEslintConfig(
eslintConfigBase,
{
rules: jsenvEslintRules,
},
eslintConfigForPrettier,
// import plugin
{
plugins: ["import"],
settings: {
"import/resolver": {
["@jsenv/importmap-eslint-resolver"]: {
projectDirectoryUrl: __dirname,
importMapFileRelativeUrl: "./import-map.importmap",
},
},
},
rules: jsenvEslintRulesForImport,
},
// html plugin
{
plugins: ["html"],
settings: {
extensions: [".html"],
},
},
// files are written for browsers by default
{
env: {
browser: true,
},
},
// and some files are written for node as es modules
{
overrides: [
{
files: [".github/**/*.js", "script/**/*.js", "jsenv.config.js"],
env: {
browser: false,
node: true,
},
globals: {
__filename: "off",
__dirname: "off",
require: "off",
},
settings: {
"import/resolver": {
[importResolverPath]: {
node: true,
},
},
},
},
],
},
// and some are written for node as commonjs modules
{
overrides: [
{
files: ["**/*.cjs"],
env: {
browser: false,
node: true,
},
globals: {
__filename: true,
__dirname: true,
require: true,
},
settings: {
"import/resolver": {
[importResolverPath]: {
node: true,
},
},
},
},
],
},
)
module.exports = eslintConfig
Add
"eslint.validate": ["javascript", "html"]
In .vscode/settings.json
FAQs
Create ESLint configuration for any project
We found that @jsenv/eslint-config demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
Application Security
New SEC disclosure rules aim to enforce timely cyber incident reporting, but fear of job loss and inadequate resources lead to significant underreporting.
Security News
The Python Software Foundation has secured a 5-year sponsorship from Fastly that supports PSF's activities and events, most notably the security and reliability of the Python Package Index (PyPI).