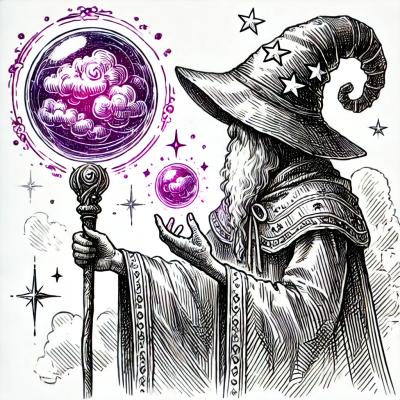
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@jsenv/import-map
Advanced tools
Helpers to implement importmaps.
@jsenv/import-map
can be used to implement the behaviour of importMap as described in the specification. It is written using es modules and is compatible with Node.js.
— see importMap spec
npm install --save-dev @jsenv/import-map@6.7.2
@jsenv/import-map
exports are documented in this section.
composeTwoImportMaps
takes two importMap
and return a single importMap
being the composition of the two.
import { composeTwoImportMaps } from "@jsenv/import-map"
const importMap = composeTwoImportMaps(
{
imports: {
foo: "bar",
},
},
{
imports: {
foo: "whatever",
},
},
)
console.log(JSON.stringify(importMap, null, " "))
{
"imports": {
"foo": "whatever"
}
}
— source code at src/composeTwoImportMaps.js.
normalizeImportMap
returns an importMap
resolved against an url
and sorted.
import { normalizeImportMap } from "@jsenv/import-map"
const importMap = normalizeImportMap(
{
imports: {
foo: "./bar",
"./ding.js": "./dong.js"
},
},
"http://your-domain.com",
)
console.log(JSON.stringify(importMap, null, ' ')
{
"imports": {
"foo": "http://your-domain.com/bar",
"http://your-domain.com/ding.js": "http://your-domain.com/dong.js"
}
}
— source code at src/normalizeImportMap.js.
resolveImport
returns an import url
applying an importMap
to specifier
and importer
. The provided importMap
must be resolved and sorted to work as expected. You can use normalizeImportMap to do that.
import { resolveImport } from "@jsenv/import-map"
const importUrl = resolveImport({
specifier: "../index.js",
importer: "http://domain.com/folder/file.js",
importMap: {
imports: {
"http://domain.com/index.js": "http://domain.com/main.js",
},
},
})
console.log(importUrl)
http://domain.com/main.js
— source code at src/resolveImport.js.
FAQs
Helpers to implement importmaps.
The npm package @jsenv/import-map receives a total of 349 weekly downloads. As such, @jsenv/import-map popularity was classified as not popular.
We found that @jsenv/import-map demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.