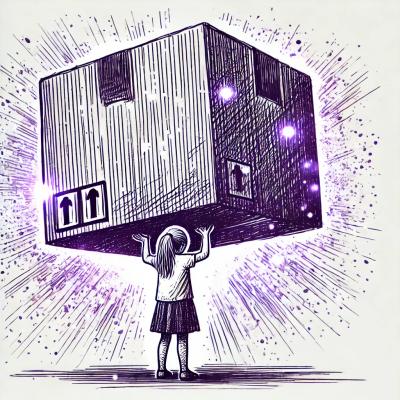
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
@jsenv/url-meta
Advanced tools
Associate data to urls using patterns.
jsenv-url-meta
github repository corresponds to @jsenv/url-meta
package published on github and npm package registries.
@jsenv/url-meta
can be used to you associate any information to one or more url at once using pattern matching.
import { urlToMeta } from "@jsenv/url-meta"
const specifierMetaMap = {
"http://your-domain.com/*": {
fromYourDomain: true,
color: "black",
},
"http://your-domain.com/*.js": {
color: "red",
},
}
const urlA = "http://your-domain.com/file.json"
const urlB = "http://your-domain.com/file.js"
const urlAMeta = urlToMeta({ specifierMetaMap, url: urlA })
const urlBMeta = urlToMeta({ specifierMetaMap, url: urlB })
console.log(`${urlA}: ${JSON.stringify(urlAMeta, null, " ")}`)
console.log(`${urlB}: ${JSON.stringify(urlBMeta, null, " ")}`)
Code above logs
http://your-domain.com/file.json: {
"fromYourDomain": true,
"color": "black",
}
http://your-domain.com/file.js: {
"fromYourDomain": true,
"color": "red",
}
The table below gives an idea of how pattern matching behaves.
specifier | url | matches |
---|---|---|
/folder | http://domain.com/folder/file.js | false |
/folder/*.js | http://domain.com/folder/file.js | true |
/folder/**/*.js | http://domain.com/folder/file.js | true |
/**/*.js | http://domain.com/folder/file.js | true |
/folder/file.js | http://domain.com/folder/file.js | true |
/folder/file.jsx | http://domain.com/folder/file.js | false |
@jsenv/url-meta
api is documented here.
applySpecifierPatternMatching
is a function returning amatchResult
indicating if and how aspecifier
matches anurl
.
Implemented in src/applySpecifierPatternMatching/applySpecifierPatternMatching.js and could be used as shown below.
import { applySpecifierPatternMatching } from "@jsenv/url-meta"
const matchResult = applySpecifierPatternMatching({
specifier: "file:///**/*",
url: "file://Users/folder/file.js",
})
specifier
is a string looking like an url but where*
and**
can be used so that one specifier can match several url.
This parameter is required, an example value could be:
"http://domain.com/**/*.js"
url
is a string representing a url.
This parameter is required, an example value could be:
"http://domain.com/folder/file.js"
matchResult
represents if and howspecifier
matchesurl
.
It is returned by applySpecifierPatternMatching
, an example value could be:
{
matched: false,
index: 4,
patternIndex: 1
}
Meaning specifier
partially matched url
.
Or
{
matched: true,
index: 4,
patternIndex: 1
}
Meaning specifier
full matched url
.
metaMapToSpecifierMetaMap
is a function used to convert ametaMap
into aspecifierMetaMap
.
Implemented in src/metaMapToSpecifierMetaMap/metaMapToSpecifierMetaMap.js, you can use it as shown below.
import { metaMapToSpecifierMetaMap } from "@jsenv/url-meta"
const specifierMetaMap = metaMapToSpecifierMetaMap({
visible: {
"file:///**/*": true,
"file://**/.git": false,
},
})
metaMap
is an object where values are conditionnaly applied by specifiers.
This parameter is required, an example value could be:
{
visible: {
"file:///**/*": true,
"file://**/.git": false,
}
}
specifierMetaMap
is an object where meta (other objects) are conditionnaly applied by specifier.
It is returned by metaMapToSpecifierMetaMap
, an example value could be:
{
"file:///**/*": { visible: true },
"file://**/.git": { visible: false },
}
normalizeSpecifierMetaMap
is a function resolvingspecifierMetaMap
keys against anurl
Implemented in src/normalizeSpecifierMetaMap/normalizeSpecifierMetaMap.js, you can use it as shown below.
import { normalizeSpecifierMetaMap } from "@jsenv/url-meta"
const specifierMetaMapNormalized = normalizeSpecifierMetaMap(
{
"./**/*": { visible: true },
"./**/.git": { visible: false },
},
"file:///Users/folder",
)
urlCanContainsMetaMatching
is a function designed to ignore folder content that would never have specific metas.
Implemented in src/urlCanContainsMetaMatching/urlCanContainsMetaMatching.js, you can use it as shown below.
import { urlCanContainsMetaMatching } from "@jsenv/url-meta"
const specifierMetaMap = {
"file:///**/*": {
source: true,
},
"file:///**/node_modules": {
source: false,
},
}
const predicate = ({ source }) => source === true
const urlA = "file:///node_modules/src"
const urlB = "file:///src"
console.log(
`${urlA} can contains meta matching source: ${urlCanContainsMetaMatching({
url: urlA,
specifierMetaMap,
predicate,
})}`,
)
console.log(
`${urlB} can contains meta matching source: ${urlCanContainsMetaMatching({
url: urlB,
specifierMetaMap,
predicate,
})}`,
)
Console output
file:///node_modules/src can contains meta matching source: false
file:///src can contains meta matching source: true
urlToMeta
is a function returning an object being the composition of all object associated with a matching specifier.
Implemented in src/urlToMeta/urlToMeta.js, you can use it as shown below.
import { urlToMeta } from "@jsenv/url-meta"
const specifierMetaMap = {
"file:///src": {
insideSrcDirectory: true,
},
"file:///**/*.js": {
extensionIsJs: true,
},
}
const urlA = "file:///src/file.js"
const urlB = "file:///src/file.json"
console.log(`${urlA}: ${JSON.stringify(urlToMeta({ url: urlA, specifierMetaMap }), null, " ")}`)
console.log(`${urlB}: ${JSON.stringify(urlToMeta({ url: urlB, specifierMetaMap }), null, " ")}`)
Console output
file:///src/file.js: {
"insideSrcDirectory": true,
"extensionIsJs": true,
}
file:///src/file.json: {
"insideSrcDirectory": true
}
If you never installed a jsenv package, read Installing a jsenv package before going further.
This documentation is up-to-date with a specific version so prefer any of the following commands
npm install --save-dev @jsenv/url-meta@4.0.0
yarn add --dev @jsenv/url-meta@4.0.0
34.0.0
import.meta.resolve
FAQs
Associate value to urls using pattern matching.
The npm package @jsenv/url-meta receives a total of 332 weekly downloads. As such, @jsenv/url-meta popularity was classified as not popular.
We found that @jsenv/url-meta demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.