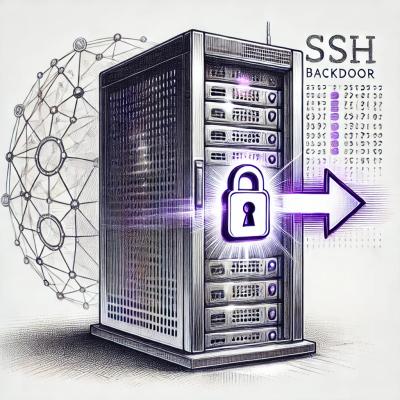
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@katomaran/nest-jwt-auth
Advanced tools
This package provides utilities for creating, verifying, and decoding JSON Web Tokens (JWTs) with support for both access and refresh tokens. It is designed for NestJS applications that require JWT-based authentication.
This package provides utilities for creating, verifying, and decoding JSON Web Tokens (JWTs) with support for both access and refresh tokens. It is designed for NestJS applications that require JWT-based authentication.
Environment Variables This package relies on the following environment variables to be set in the parent application’s .env file:
REFRESH_TOKEN_KEY: Secret key for signing refresh tokens. ACCESS_PRIVATE_KEY: RSA private key for signing access tokens. ACCESS_PUBLIC_KEY: RSA public key for verifying access tokens. Example .env file:
env
REFRESH_TOKEN_KEY=your_refresh_token_secret
ACCESS_PRIVATE_KEY="your_private_key"
ACCESS_PUBLIC_KEY="your_public_key"
npm install @katomaran/nest-jwt-auth dotenv
createRefreshToken(_id: string): string Generates a refresh token signed with the REFRESH_TOKEN_KEY.
Parameters: _id (string) - The unique identifier of the user. Returns: A JWT refresh token as a string, valid for 30 days. Usage Example:
const refreshToken = jwtAuthService.createRefreshToken(user._id);
createAccessToken(payload: JwtPayload): string
Generates an access token using the ACCESS_PRIVATE_KEY, which is signed with the RS256 algorithm.
Parameters: payload (JwtPayload) - An object containing user data (_id, role, email). Returns: A JWT access token as a string, valid for 30 days. Usage Example:
const accessToken = jwtAuthService.createAccessToken({
_id: user._id,
role: user.role,
email: user.email,
});
createVerificationAccessToken(payload: JwtPayload): string
Generates a verification access token with a shorter expiry, suitable for tasks like email verification.
Parameters: payload (JwtPayload) - An object containing user data (_id, role, email). Returns: A JWT verification token as a string, valid for 6 hours. Usage Example:
const verificationToken = jwtAuthService.createVerificationAccessToken({
_id: user._id,
role: user.role,
email: user.email,
});
verifyAccessToken(token: string): JwtPayload | string
Verifies an access token using the ACCESS_PUBLIC_KEY. If the token is valid, it returns the payload; otherwise, it throws an error.
Parameters: token (string) - The JWT access token to be verified. Returns: The decoded payload if the token is valid; otherwise, an error is thrown. Usage Example:
try {
const payload = jwtAuthService.verifyAccessToken(token);
// Token is valid, proceed with payload data
} catch (error) {
// Handle invalid token
}
verifyRefreshToken(token: string): JwtPayload | string
Verifies a refresh token using the REFRESH_TOKEN_KEY. If the token is valid, it returns the payload; otherwise, it throws an error.
Parameters: token (string) - The JWT refresh token to be verified. Returns: The decoded payload if the token is valid; otherwise, an error is thrown. Usage Example:
try {
const payload = jwtAuthService.verifyRefreshToken(refreshToken);
// Token is valid, proceed with payload data
} catch (error) {
// Handle invalid token
}
verifyAdminToken(token: string): JwtPayload | string
Verifies an admin token using the ACCESS_PUBLIC_KEY, allowing secure access for admin-specific endpoints.
Parameters: token (string) - The JWT admin token to be verified. Returns: The decoded payload if the token is valid; otherwise, an error is thrown. Usage Example:
try {
const payload = jwtAuthService.verifyAdminToken(adminToken);
// Token is valid, proceed with payload data
} catch (error) {
// Handle invalid token
}
decodeAdminToken(token: string): JwtPayload | null
Decodes an admin token without verifying its signature, useful for extracting information without validating authenticity.
Parameters: token (string) - The JWT admin token to decode. Returns: The decoded payload if the token is valid; null if decoding fails. Usage Example:
const decoded = jwtAuthService.decodeAdminToken(adminToken);
if (decoded) {
// Proceed with decoded information
}
Example Usage in a NestJS Service
Here’s how you might use these functions in a NestJS authentication service:
import { Injectable } from '@nestjs/common';
import { JwtAuthService, JwtPayload } from '@katomaran/nest-jwt-auth';
@Injectable()
export class AuthService {
constructor(private readonly jwtAuthService: JwtAuthService) {}
async login(user: JwtPayload) {
const accessToken = this.jwtAuthService.createAccessToken(user);
const refreshToken = this.jwtAuthService.createRefreshToken(user._id);
return { accessToken, refreshToken };
}
async validateAccessToken(token: string) {
return this.jwtAuthService.verifyAccessToken(token);
}
}
This setup provides all the tools needed to handle JWT-based authentication in a modular way, utilizing environment variables for security and flexibility.
FAQs
This package provides utilities for creating, verifying, and decoding JSON Web Tokens (JWTs) with support for both access and refresh tokens. It is designed for NestJS applications that require JWT-based authentication.
We found that @katomaran/nest-jwt-auth demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.