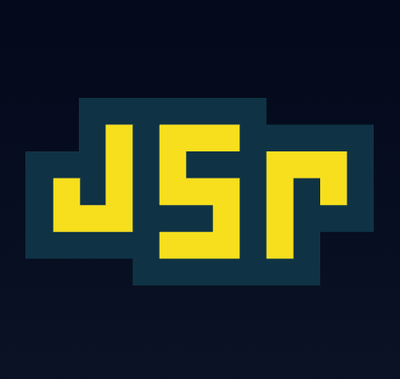
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@kentico/kontent-delivery
Advanced tools
A client library for retrieving content from Kentico Kontent written in TypeScript and published in following formats: UMD
, ES2015
and CommonJs
. Works both in browser & node.
Resources |
---|
Full Documentation |
Example apps |
Upgrade guide |
You can install this library using npm
or you can use global CDNs such jsdelivr
directly. In both cases, you will also need to include rxjs
as its listed as peer dependency.
npm i rxjs --save
npm i @kentico/kontent-delivery --save
When using UMD bundle and including this library in script
tag on your html
page, you can find it under the kontentDelivery
global variable.
Bundles are distributed in _bundles
folder and there are several options that you can choose from.
kontent-delivery.browser.legacy.umd.min
if you need to support legacy browsers (IE9, IE10, IE11)kontent-delivery.browser.umd.min
if you intend to use SDK only in browsers (strips code specific to Node.js = smaller bundle)kontent-delivery.umd.min
if you need to use it in Node.jshttps://cdn.jsdelivr.net/npm/@kentico/kontent-delivery/_bundles/kontent-delivery.browser.legacy.umd.min.js
https://cdn.jsdelivr.net/npm/@kentico/kontent-delivery/_bundles/kontent-delivery.browser.umd.min.js
https://cdn.jsdelivr.net/npm/@kentico/kontent-delivery/_bundles/kontent-delivery.umd.min.js
import {
ContentItem,
Elements,
TypeResolver,
DeliveryClient
} from '@kentico/kontent-delivery';
/**
* This is optional, but it is considered a best practice to define your models
* so you can leverage intellisense and so that you can extend your models with
* additional properties / methods.
*/
export class Movie extends ContentItem {
public title: Elements.TextElement;
}
const deliveryClient = new DeliveryClient({
projectId: 'xxx',
typeResolvers: [
new TypeResolver('movie', () => new Movie()),
]
});
/** Getting items from Kentico Kontent as Promise */
deliveryClient.items<Movie>()
.type('movie')
.toPromise()
.then(response => {
const movieText = response.items[0].title.value;
});
/** Getting items from Kentico Kontent as Observable */
deliveryClient.items<Movie>()
.type('movie')
.toObservable()
.subscribe(response => {
const movieText = response.items[0].title.value;
});
/**
* Get data without having custom models
*/
deliveryClient.items<ContentItem>()
.type('movie')
.get()
.subscribe(response => {
// you can access properties same way as with strongly typed models, but note
// that you don't get any intellisense and the underlying object
// instance is of 'ContentItem' type
console.log(response.items[0].title.value);
});
const KontentDelivery = require('@kentico/kontent-delivery');
class Movie extends KontentDelivery.ContentItem {
constructor() {
super();
}
}
const deliveryClient = new KontentDelivery.DeliveryClient({
projectId: 'xxx',
typeResolvers: [
new KontentDelivery.TypeResolver('movie', () => new Movie()),
]
});
/** Getting items from Kentico Kontent as Promise */
deliveryClient.items()
.type('movie')
.toPromise()
.then(response => {
const movieText = response.items[0].title.value;
});
/** Getting items from Kentico Kontent as Observable */
const subscription = deliveryClient.items()
.type('movie')
.toObservable()
.subscribe(response => {
const movieText = response.items[0].title.value;
});
/*
Don't forget to unsubscribe from your Observables. You can use 'takeUntil' or 'unsubscribe' method for this purpose. Unsubsription is usually done when you no longer need to process the result of Observable. (Example: 'ngOnDestroy' event in Angular app)
*/
subscription.unsubscribe();
/**
* Fetch all items of 'movie' type and given parameters from Kentico Kontent.
* Important note: SDK will convert items to your type if you registered it. For example,
* in this case the objects will be of 'Movie' type we defined above.
* If you don't use custom models, 'ContentItem' object instances will be returned.
*/
deliveryClient.items()
.type('movie')
.toObservable()
.subscribe(response => console.log(response));
Bundles are distributed in _bundles
folder and there are several options that you can choose from.
kontent-delivery.browser.legacy.umd.min
if you need to support legacy browsers (IE9, IE10, IE11)kontent-delivery.browser.umd.min
if you intend to use SDK only in browsers (strips code specific to Node.js = smaller bundle)kontent-delivery.umd.min
if you need to use it in Node.js<!DOCTYPE html>
<html>
<head>
<title>Kentico Kontent SDK - Html sample</title>
<script type="text/javascript" src="https://unpkg.com/rxjs@6.4.0/bundles/rxjs.umd.min.js"></script>
<script type="text/javascript" src="https://cdn.jsdelivr.net/npm/@kentico/kontent-delivery/_bundles/kontent-delivery.browser.umd.min.js"></script>
</head>
<body>
<script type="text/javascript">
var Kc = window['kontentDelivery'];
var deliveryClient = new Kc.DeliveryClient({
projectId: 'da5abe9f-fdad-4168-97cd-b3464be2ccb9'
});
deliveryClient.items()
.type('movie')
.toPromise()
.then(response => console.log(response));
</script>
<h1>See console</h1>
</body>
</html>
Note: You need to have Chrome
installed in order to run tests via Karma.
npm run test:browser
Runs tests in Chromenpm run test:node
Runs tests in node.jsnpm run test:dev
Runs developer tests (useful for testing functionality)npm run test:travis
Runs browser tests that are executed by travisIf you want to mock http responses, it is possible to use external implementation of configurable Http Service as a part of the delivery client configuration.
Feedback & Contributions are welcomed. Feel free to take/start an issue & submit PR.
FAQs
Official Kentico Kontent Delivery API SDK
The npm package @kentico/kontent-delivery receives a total of 1,541 weekly downloads. As such, @kentico/kontent-delivery popularity was classified as popular.
We found that @kentico/kontent-delivery demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 11 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.