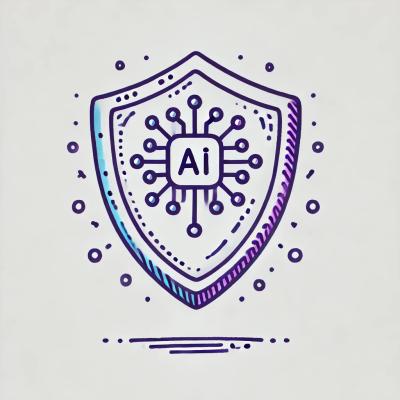
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
@kev_nz/async-tools
Advanced tools
Async tools - a collection of utility functions for working with async/await code.
This is a collection of utility functions for working with async/await code.
npm install @kev_nz/async-tools
Here are the functions provided. Additionally feel free to look at the tests in the project as well.
The delay method allows you to use wait a number of milliseconds before continuing the exection of your code.
const { delay } = require('@kev_nz/async-tools')
(async () => {
await delay(100)
console.info('~100 milliseconds later')
})()
Execute an asynchronous mapping function over an array of returning the result.
const { mapper } = require('@kev_nz/async-tools')
(async () => {
const items = [1, 2, 3]
const mappedItems = await mapper(items, anAsynchronousFunction)
// passing in an additional concurrency value specifies the number of async methods executed at a time
const mappedItemsAgain = await mapper(items, anAsynchronousFunction, 2)
})()
Execute asynchronous reducer functions over a starting value.
const { delay, reducer } = require('@kev_nz/async-tools')
const anAsyncFunction = async val => {
await delay(10)
return val + 1
}
(async () => {
const finalValue = await reducer(0,
anAsyncFunction,
anAsyncFunction,
anAsyncFunction
)
//finalValue 3
})()
Execute an asynchronous function over an array one item at a time.
const { each } = require('@kev_nz/async-tools')
(async () => {
const items = [1, 2, 3]
const [first, second, third] = await each(items, anAsyncFunction)
})()
Take number of functions and compose them together.
const { composer } = require('@kev_nz/async-tools')
const anAsyncFunction = async val => {
await delay(10)
return val + 1
}
const anotherAsyncFunction = async val => {
await delay(10)
return val + 2
}
(async () => {
const asyncChain = composer(
anAsyncFunction,
anotherAsyncFunction,
anAsyncFunction
)
const finalValue = await asyncChain(0)
//finalValue 4
})()
When used with composer it lets you pass additional parameters to a function in the composed.
const { composer, holder } = require('@kev_nz/async-tools')
const anAsyncFunction = async val => {
await delay(10)
return val + 1
}
const anotherAsyncFunction = async (val, secondValue) => {
await delay(10)
return val + secondValue
}
(async () => {
const asyncChain = composer(
anAsyncFunction,
holder(anotherAsyncFunction, 3),
anAsyncFunction
)
const finalValue = await asyncChain(0)
//finalValue 5
})()
Take number of functions and compose them together.
const { timeout } = require('@kev_nz/async-tools')
(async () => {
const result = await timeout(() => thingThatIsLongRunning())
})()
Execute an async function while a condition is true
const { doWhile } = require('@kev_nz/async-tools')
(async () => {
const results = await doWhile(async () => {
await asyncFunction()
}, () => something === true)
})()
While a condition is true execute an async function
const { whileDo } = require('@kev_nz/async-tools')
(async () => {
const results = await whileDo(
() => something === true),
async () => {
await asyncFunction()
})
})()
FAQs
Async tools - a collection of utility functions for working with async/await code.
We found that @kev_nz/async-tools demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.