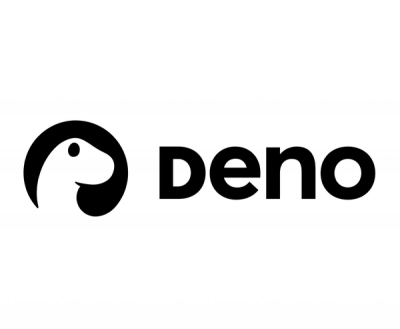
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@lecoupa/v-hotkey
Advanced tools
Vue 2.x directive for binding hotkeys to components.
https://dafrok.github.io/v-hotkey
$ npm i --save v-hotkey
import Vue from 'vue'
import VueHotkey from 'v-hotkey'
Vue.use(VueHotkey)
<template>
<span v-hotkey="keymap" v-show="show"> Press `ctrl + esc` to toggle me! Hold `enter` to hide me! </span>
</template>
<script>
export default {
data () {
return {
show: true
}
},
methods: {
toggle () {
this.show = !this.show
},
show () {
this.show = true
},
hide () {
this.show = false
}
},
computed: {
keymap () {
return {
// 'esc+ctrl' is OK.
'ctrl+esc': this.toggle,
'enter': {
keydown: this.hide,
keyup: this.show
}
}
}
}
}
</script>
Use one or more of following keys to fire your hotkeys.
Add the prevent modifier to the directive to prevent default browser behavior.
<template>
<span v-hotkey.prevent="keymap" v-show="show"> Press `ctrl + esc` to toggle me! Hold `enter` to hide me! </span>
</template>
Add the stop modifier to the directive to stop event propagation.
<template>
<div v-hotkey.stop="keymap">
<span> Enter characters in editable areas doesn't trigger any hotkeys. </span>
<input>
</div>
</template>
The default key code map is based on US standard keyboard. This ability to provide their own key code alias for developers who using keyboards with different layouts. The alias name must be a single character.
import Vue from 'vue'
import VueHotkey from 'v-hotkey'
Vue.use(VueHotkey, {
'①': 49 // the key code of character '1'
})
<span v-hotkey="keymap"></span>
<script>
export default {
foo () {
console.log('Hooray!')
},
computed: {
keymap () {
return {
'①': foo
}
}
}
}
</script>
FAQs
Vue 2.x directive - binding hotkeys for components.
The npm package @lecoupa/v-hotkey receives a total of 1 weekly downloads. As such, @lecoupa/v-hotkey popularity was classified as not popular.
We found that @lecoupa/v-hotkey demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.