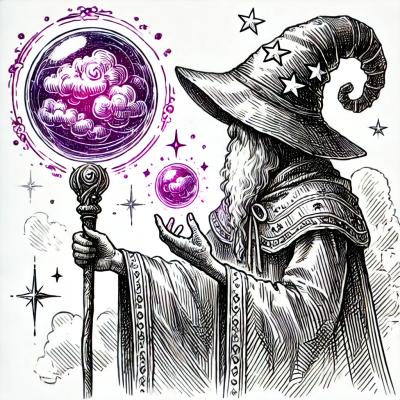
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@lerna/describe-ref
Advanced tools
@lerna/describe-ref is a utility package that helps in describing the current Git reference in a Lerna-managed monorepo. It provides information about the current Git branch, tag, or commit, which can be useful for versioning and release management in monorepos.
Describe the current Git reference
This feature allows you to get a description of the current Git reference, including branch name, tag, or commit hash. The `describeRef` function returns an object with details about the current Git state.
const { describeRef } = require('@lerna/describe-ref');
async function getGitRef() {
const ref = await describeRef();
console.log(ref);
}
getGitRef();
Custom base ref
This feature allows you to specify a custom base reference to compare against. The `describeRef` function can take an options object where you can set the `baseRef` to a specific branch or tag.
const { describeRef } = require('@lerna/describe-ref');
async function getCustomBaseRef(baseRef) {
const ref = await describeRef({ baseRef });
console.log(ref);
}
getCustomBaseRef('main');
Include distance from base ref
This feature includes the distance (number of commits) from the base reference in the description. The `includeDistance` option can be set to `true` to get this additional information.
const { describeRef } = require('@lerna/describe-ref');
async function getRefWithDistance() {
const ref = await describeRef({ includeDistance: true });
console.log(ref);
}
getRefWithDistance();
git-rev-sync is a package that provides synchronous access to Git information such as the current branch, commit hash, and tag. It is similar to @lerna/describe-ref but operates synchronously and is not specifically designed for monorepos.
git-describe is a package that provides a way to describe a Git commit using tags and commit distance. It is similar to @lerna/describe-ref but focuses on general Git repositories rather than Lerna-managed monorepos.
simple-git is a lightweight interface for running Git commands in any Node.js application. It provides a broader range of Git functionalities compared to @lerna/describe-ref, which is more specialized for describing Git references in monorepos.
@lerna/describe-ref
Parse git describe output for lerna-related tags
const { describe } = require("@lerna/describe-ref");
(async () => {
const { lastTagName, lastVersion, refCount, sha, isDirty } = await describe();
})();
// values listed here are their defaults
const options = {
cwd: process.cwd(),
// pass a glob to match tag name, e.g. "v*.*.*"
match: undefined,
// if true, omit --first-parent option
includeMergedTags: false,
};
const { lastTagName, lastVersion, refCount, sha, isDirty } = describe.sync(options);
const result = describe.parse("v1.0.0-5-gdeadbeef");
// { lastTagName, lastVersion, refCount, sha, isDirty }
Install lerna for access to the lerna
CLI.
FAQs
Parse git describe output for lerna-related tags
The npm package @lerna/describe-ref receives a total of 424,325 weekly downloads. As such, @lerna/describe-ref popularity was classified as popular.
We found that @lerna/describe-ref demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.