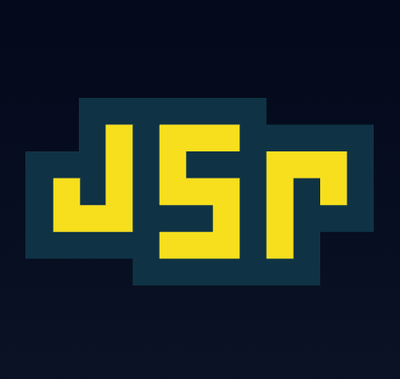
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@lexical/list
Advanced tools
@lexical/list is a package designed to provide list functionalities within the Lexical framework, a modern web text editor. It allows developers to create, manage, and manipulate ordered and unordered lists in a rich text editor environment.
Creating Ordered Lists
This feature allows you to create an ordered list (numbered list) within the Lexical editor. The code sample demonstrates how to create a new ordered list node and append it to the editor's root.
import { $createListNode, ListNode } from '@lexical/list';
const orderedListNode = $createListNode('number');
editor.update(() => {
const root = $getRoot();
root.append(orderedListNode);
});
Creating Unordered Lists
This feature allows you to create an unordered list (bulleted list) within the Lexical editor. The code sample shows how to create a new unordered list node and append it to the editor's root.
import { $createListNode, ListNode } from '@lexical/list';
const unorderedListNode = $createListNode('bullet');
editor.update(() => {
const root = $getRoot();
root.append(unorderedListNode);
});
Converting List Types
This feature allows you to convert an existing list from one type to another (e.g., from ordered to unordered). The code sample demonstrates how to find a list node in the current selection and change its type.
import { $createListNode, ListNode } from '@lexical/list';
editor.update(() => {
const selection = $getSelection();
if (selection !== null) {
const listNode = selection.getNodes().find(node => node instanceof ListNode);
if (listNode !== null) {
listNode.setListType('bullet'); // Convert to unordered list
}
}
});
Draft.js is a rich text editor framework for React. It provides extensive support for creating and managing lists, including ordered and unordered lists. Compared to @lexical/list, Draft.js offers a more comprehensive set of features for building rich text editors but may have a steeper learning curve.
Slate is another popular framework for building rich text editors in React. It offers robust support for lists and other rich text features. Slate is highly customizable and flexible, making it a strong alternative to @lexical/list for developers needing more control over their editor's behavior.
ProseMirror is a toolkit for building rich text editors with a focus on extensibility and performance. It includes built-in support for lists and other rich text elements. ProseMirror is known for its modular architecture, which allows developers to create highly customized editing experiences.
@lexical/list
This package exposes the primitives for implementing lists in Lexical. If you're trying to implement conventional lists with React, take a look at the ListPlugin exposed by @lexical/react, which wraps these primitives into a neat component that you can drop into any LexicalComposer.
The API of @lexical/list primarily consists of Lexical Nodes that encapsulate list behaviors and a set of functions that can be called to trigger typical list manipulation functionality:
As the name suggests, this inserts a list of the provided type according to an algorithm that tries to determine the best way to do that based on the current Selection. For instance, if some text is selected, insertList may try to move it into the first item in the list. See the API documentation for more detail.
Attempts to remove lists inside the current selection based on a set of opinionated heuristics that implement conventional editor behaviors. For instance, it converts empty ListItemNodes into empty ParagraphNodes.
For convenience, we provide a set of commands that can be used to connect a plugin to trigger typical list manipulation functionality:
It's important to note that these commands don't have any functionality on their own. They are just for convenience and require you to register a handler for them in order to actually change the editor state when they are dispatched, as below:
// MyListPlugin.ts
editor.registerCommand(INSERT_UNORDERED_LIST_COMMAND, () => {
insertList(editor, 'bullet');
return true;
}, COMMAND_PRIORITY_LOW);
// MyInsertListToolbarButton.ts
function onButtonClick(e: MouseEvent) {
editor.dispatchCommand(INSERT_UNORDERED_LIST_COMMAND, undefined);
}
Lists can be styled using the following properties in the EditorTheme passed to the editor in the initial config (the values are classes that will be applied in the denoted contexts):
{
list?: {
// Applies to all lists of type "bullet"
ul?: EditorThemeClassName;
// Used to apply specific styling to nested levels of bullet lists
// e.g., [ 'bullet-list-level-one', 'bullet-list-level-two' ]
ulDepth?: Array<EditorThemeClassName>;
// Applies to all lists of type "number"
ol?: EditorThemeClassName;
// Used to apply specific styling to nested levels of number lists
// e.g., [ 'number-list-level-one', 'number-list-level-two' ]
olDepth?: Array<EditorThemeClassName>;
// Applies to all list items
listitem?: EditorThemeClassName;
// Applies to all list items with checked property set to "true"
listitemChecked?: EditorThemeClassName;
// Applies to all list items with checked property set to "false"
listitemUnchecked?: EditorThemeClassName;
// Applies only to list and list items that are not at the top level.
nested?: {
list?: EditorThemeClassName;
listitem?: EditorThemeClassName;
};
};
}
FAQs
This package provides the list feature for Lexical.
The npm package @lexical/list receives a total of 348,526 weekly downloads. As such, @lexical/list popularity was classified as popular.
We found that @lexical/list demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.